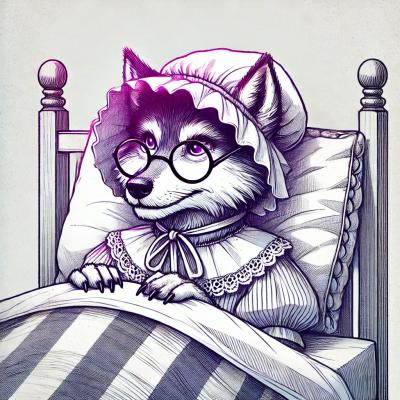
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@lexical/react
Advanced tools
This package provides Lexical components and hooks for React applications.
@lexical/react is a powerful and flexible text editor framework for React applications. It provides a set of tools and components to build rich text editors with ease, offering features like collaborative editing, custom formatting, and extensibility.
Basic Editor Setup
This code sets up a basic Lexical editor with rich text capabilities and history support. The LexicalComposer component initializes the editor with a configuration, and the RichTextPlugin and HistoryPlugin add rich text editing and undo/redo functionality.
import React from 'react';
import { LexicalComposer } from '@lexical/react/LexicalComposer';
import { RichTextPlugin } from '@lexical/react/LexicalRichTextPlugin';
import { ContentEditable } from '@lexical/react/LexicalContentEditable';
import { HistoryPlugin } from '@lexical/react/LexicalHistoryPlugin';
const editorConfig = {
namespace: 'MyEditor',
theme: {},
onError: (error) => {
console.error(error);
},
};
function MyEditor() {
return (
<LexicalComposer initialConfig={editorConfig}>
<RichTextPlugin
contentEditable={<ContentEditable />}
placeholder={<div>Enter some text...</div>}
/>
<HistoryPlugin />
</LexicalComposer>
);
}
export default MyEditor;
Custom Formatting
This code adds a toolbar to the Lexical editor, allowing users to apply custom formatting to their text. The ToolbarPlugin provides a set of formatting options like bold, italic, and underline.
import React from 'react';
import { LexicalComposer } from '@lexical/react/LexicalComposer';
import { RichTextPlugin } from '@lexical/react/LexicalRichTextPlugin';
import { ContentEditable } from '@lexical/react/LexicalContentEditable';
import { HistoryPlugin } from '@lexical/react/LexicalHistoryPlugin';
import { ToolbarPlugin } from '@lexical/react/LexicalToolbarPlugin';
import { $createTextNode } from 'lexical';
const editorConfig = {
namespace: 'MyEditor',
theme: {},
onError: (error) => {
console.error(error);
},
};
function MyEditor() {
return (
<LexicalComposer initialConfig={editorConfig}>
<ToolbarPlugin />
<RichTextPlugin
contentEditable={<ContentEditable />}
placeholder={<div>Enter some text...</div>}
/>
<HistoryPlugin />
</LexicalComposer>
);
}
export default MyEditor;
Collaborative Editing
This code enables collaborative editing in the Lexical editor. The CollaborationPlugin allows multiple users to edit the same document in real-time, identified by a unique room ID.
import React from 'react';
import { LexicalComposer } from '@lexical/react/LexicalComposer';
import { RichTextPlugin } from '@lexical/react/LexicalRichTextPlugin';
import { ContentEditable } from '@lexical/react/LexicalContentEditable';
import { HistoryPlugin } from '@lexical/react/LexicalHistoryPlugin';
import { CollaborationPlugin } from '@lexical/react/LexicalCollaborationPlugin';
const editorConfig = {
namespace: 'MyEditor',
theme: {},
onError: (error) => {
console.error(error);
},
};
function MyEditor() {
return (
<LexicalComposer initialConfig={editorConfig}>
<RichTextPlugin
contentEditable={<ContentEditable />}
placeholder={<div>Enter some text...</div>}
/>
<HistoryPlugin />
<CollaborationPlugin id="my-collab-room" />
</LexicalComposer>
);
}
export default MyEditor;
Draft.js is a framework for building rich text editors in React. It provides a set of immutable models and helper functions to manage editor state and content. Compared to @lexical/react, Draft.js is more mature but may require more boilerplate code for advanced features.
Slate is a highly customizable framework for building rich text editors in React. It offers a more flexible and extensible architecture compared to @lexical/react, allowing developers to define their own data models and editor behaviors. However, this flexibility can come with a steeper learning curve.
Quill is a modern WYSIWYG editor built for the web. It provides a rich set of features out of the box, including formatting, themes, and modules for extending functionality. Quill is less focused on React specifically but can be integrated with React applications using wrappers like react-quill.
@lexical/react
This package provides a set of components and hooks for Lexical that allow for text editing in React applications.
Install lexical
and @lexical/react
:
npm install --save lexical @lexical/react
Below is an example of a basic plain text editor using lexical
and @lexical/react
(try it yourself).
import {$getRoot, $getSelection} from 'lexical';
import {useEffect} from 'react';
import LexicalComposer from '@lexical/react/LexicalComposer';
import LexicalPlainTextPlugin from '@lexical/react/LexicalPlainTextPlugin';
import LexicalContentEditable from '@lexical/react/LexicalContentEditable';
import {HistoryPlugin} from '@lexical/react/LexicalHistoryPlugin';
import LexicalOnChangePlugin from '@lexical/react/LexicalOnChangePlugin';
import {useLexicalComposerContext} from '@lexical/react/LexicalComposerContext';
const theme = {
// Theme styling goes here
...
}
// When the editor changes, you can get notified via the
// LexicalOnChangePlugin!
function onChange(editorState) {
editorState.read(() => {
// Read the contents of the EditorState here.
const root = $getRoot();
const selection = $getSelection();
console.log(root, selection);
});
}
// Lexical React plugins are React components, which makes them
// highly composable. Furthermore, you can lazy load plugins if
// desired, so you don't pay the cost for plugins until you
// actually use them.
function MyCustomAutoFocusPlugin() {
const [editor] = useLexicalComposerContext();
useEffect(() => {
// Focus the editor when the effect fires!
editor.focus();
}, [editor]);
return null;
}
// Catch any errors that occur during Lexical updates and log them
// or throw them as needed. If you don't throw them, Lexical will
// try to recover gracefully without losing user data.
function onError(error) {
throw error;
}
function Editor() {
const initialConfig = {
theme,
onError,
};
return (
<LexicalComposer initialConfig={initialConfig}>
<LexicalPlainTextPlugin
contentEditable={<LexicalContentEditable />}
placeholder={<div>Enter some text...</div>}
/>
<LexicalOnChangePlugin onChange={onChange} />
<HistoryPlugin />
<MyCustomAutoFocusPlugin />
</LexicalComposer>
);
}
0.2.5 (April 28, 2022)
FAQs
This package provides Lexical components and hooks for React applications.
The npm package @lexical/react receives a total of 216,683 weekly downloads. As such, @lexical/react popularity was classified as popular.
We found that @lexical/react demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.