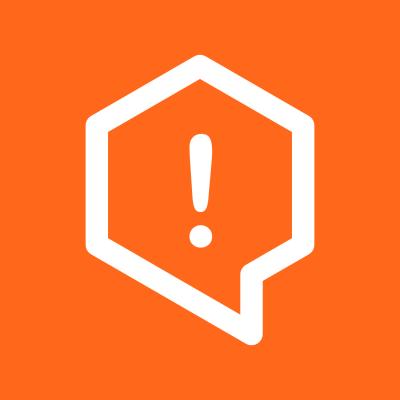
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
@lifespikes/laravel-precognition-react
Advanced tools
A set of React Hooks for Laravel Precognition
A set of React hooks that integrates Laravel Precognition with Inertia.js and simple forms.
The hooks are highly based on laravel-precognition-vue.
npm i @lifespikes/laravel-precognition-react
yarn add @lifespikes/laravel-precognition-react
pnpm add @lifespikes/laravel-precognition-react
The package provides two hooks: usePrecognitionForm
and useInertiaPrecognitionForm
.
Both hooks receive a props
object, that follows the following structure:
{
precognition: { // This is where the precognition configuration
method: 'put' // PUT, PATCH, POST, DELETE
url: 'https://example.com' // URL to send the precognition request
},
form: {
initialValues: {
example: 'value value'
} // Initial values of the form
}
}
Also, both hooks return the next properties and methods related to the usePrecognition
hook:
validator
- The validator instancevalidate(name)
- Validate a specific fieldisValidating
- Whether the form is validatingisProcessingValidation
- Whether the form is processing the validationlastTouched
- The last touched fieldsetLastTouched
- Set the last touched field(This will also trigger the validation)touched
- The touched fields,setValidatorTimeout(duration)
- Set the timeout for the validation.For using with Inertia.js, you need to import useInertiaPrecognitionForm
hook.
The useInertiaPrecognitionForm
hook uses Inertia.js useForm
form helper hook under the hood. So it'll return the same properties and methods as the useForm
hook.
import React, { ChangeEvent, FormEvent } from 'react';
import {useInertiaPrecognitionForm} from '@lifespikes/laravel-precognition-react';
type FormFields = {
name: string;
email: string;
}
const ExampleForm = () => {
const route = 'https://example.com/user/1';
const {
data,
setData,
put,
processing,
errors,
validateAndSetDataByKeyValuePair,
isProcessingValidation,
} = useInertiaPrecognitionForm<FormFields>({
precognition: {
method: 'put',
url: route,
},
form: {
initialValues: {
name: '',
email: '',
},
},
});
const onHandleChange = (event: ChangeEvent<HTMLInputElement>) => {
// This will validate the input and update the form data
validateAndSetDataByKeyValuePair(
event.target.name as keyof FormFields,
event.target.value,
);
}
const onSubmit = (event: FormEvent<HTMLFormElement>) => {
event.preventDefault();
return put(route, {
onSuccess: () => {
setData({
name: '',
email: '',
});
},
});
};
return (
<form onSubmit={onSubmit}>
<div>
<input
type="text"
name="name"
value={data.name}
onChange={onHandleChange}
/>
{errors.name ? <p>{errors.name}</p> : null}
</div>
<div>
<input
type="email"
name="email"
value={data.email}
onChange={onHandleChange}
/>
{errors.name ? <p>{errors.email}</p>: null}
</div>
<button type="submit" disabled={processing || isProcessingValidation}>
Submit
</button>
</form>
);
}
This is a small example of the usage of the usePrecognitionForm
hook with a simple/basic form.
Also, this hook returns the following additional properties:
data
- The form data.setData
- A function that sets the form data.errors
- The form errors.setErrors
- A function that sets the form errors.clearErrors
- A function that clears the form errors.import React, { ChangeEvent, FormEvent } from 'react';
import {usePrecognitionForm} from '@lifespikes/laravel-precognition-react';
type FormFields = {
name: string;
email: string;
}
const ExampleForm = () => {
const route = 'https://example.com/user/1';
const {
data,
setData,
errors,
validateAndSetDataByKeyValuePair,
isProcessingValidation,
} = usePrecognitionForm<FormFields>({
precognition: {
method: 'put',
url: route,
},
form: {
initialValues: {
name: '',
email: '',
},
},
});
const onHandleChange = (event: ChangeEvent<HTMLInputElement>) => {
// This will validate the input and update the form data
validateAndSetDataByKeyValuePair(
event.target.name as keyof FormFields,
event.target.value,
);
}
const onSubmit = (event: FormEvent<HTMLFormElement>) => {
event.preventDefault();
// Implement your own logic here
};
return (
<form onSubmit={onSubmit}>
<div>
<input
type="text"
name="name"
value={data.name}
onChange={onHandleChange}
/>
{errors.name ? <p>{errors.name}</p> : null}
</div>
<div>
<input
type="email"
name="email"
value={data.email}
onChange={onHandleChange}
/>
{errors.name ? <p>{errors.email}</p>: null}
</div>
<button type="submit" disabled={processing || isProcessingValidation}>
Submit
</button>
</form>
);
}
FAQs
A set of React Hooks for Laravel Precognition
The npm package @lifespikes/laravel-precognition-react receives a total of 10 weekly downloads. As such, @lifespikes/laravel-precognition-react popularity was classified as not popular.
We found that @lifespikes/laravel-precognition-react demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.