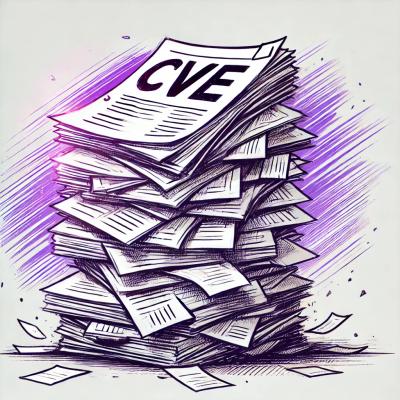
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
@lit/ts-transformers
Advanced tools
TypeScript transformers for the Lit decorators.
npm i @lit/ts-transformers
import {idiomaticDecoratorsTransformer} from '@lit/ts-transformers';
Replaces all of the official Lit class and property decorators with idiomatic vanilla JavaScript.
Must run as a before
transformer.
import {LitElement, html} from 'lit';
import {customElement, property} from 'lit/decorators.js';
@customElement('simple-greeting')
class SimpleGreeting extends LitElement {
@property()
name = 'World';
render() {
return html`<p>Hello ${this.name}!</p>`;
}
}
import {LitElement, html} from 'lit';
class SimpleGreeting extends LitElement {
static properties = {
str: {},
};
constructor() {
super();
this.name = 'World';
}
render() {
return html`Hello ${this.name}!`;
}
}
customElements.define('simple-greeting', SimpleGreeting);
Decorator | Transformer behavior |
---|---|
@customElement | Adds a customElements.define call |
@property | Adds an entry to static properties , and moves initializers to the constructor |
@state | Same as @property with {state: true} |
@query | Defines a getter that calls querySelector |
@querySelectorAll | Defines a getter that calls querySelectorAll |
@queryAsync | Defines an async getter that awaits updateComplete and then calls querySelector |
@queryAssignedElements | Defines a getter that calls querySelector('slot[name=foo]').assignedElements |
@queryAssignedNodes | Defines a getter that calls querySelector('slot[name=foo]').assignedNodes |
@localized | Adds an updateWhenLocaleChanges call to the constructor |
import {
preserveBlankLinesTransformer,
BLANK_LINE_PLACEHOLDER_COMMENT,
BLANK_LINE_PLACEHOLDER_COMMENT_REGEXP,
} from '@lit/ts-transformers';
A readability transformer that replaces blank lines in the original source with a special comment, because TypeScript does not otherwise preserve blank lines when it emits (see TypeScript#843).
The comment is always exactly:
//__BLANK_LINE_PLACEHOLDER_G1JVXUEBNCL6YN5NFE13MD1PT3H9OIHB__
Must run as a before
transformer, and should usually placed in front of
all other transformers.
import {constructorCleanupTransformer} from '@lit/ts-transformers';
A readability transformer that does the following:
Moves constructors back to their original source position, or below the last
static
field if they are fully synthetic. By default, constructors will move
to the top of a class whenever they are modified by TypeScript.
Simplifies super(...)
calls to super()
in class constructors, unless the
class has any super-classes with constructors that takes parameters according
to the type-checker.
Must run as an after
transformer.
There are a number of ways to compile a TypeScript program with transformers.
If you are using the TypeScript compiler
API
directly, pass the transformer to the customTransformers
parameter of emit
.
Example:
import ts from 'typescript';
import {
idiomaticDecoratorsTransformer,
preserveBlankLinesTransformer,
constructorCleanupTransformer,
} from '@lit/ts-transformers';
// Note this is not a complete example. For more information see
// https://github.com/microsoft/TypeScript-wiki/blob/master/Using-the-Compiler-API.md
const program = ts.createProgram(...);
const result = program.emit(undefined, undefined, undefined, undefined, {
before: [
// Optionally preserve blank lines for better readability.
preserveBlankLinesTransformer(),
// Transform Lit decorators to idiomatic vanilla JavaScript.
idiomaticLitDecoratorTransformer(program),
],
after: [
// Optional readability improvements for constructors.
constructorCleanupTransformer(program),
],
});
ttypescript and
ts-patch are two similar tools that
augment the TypeScript compiler, adding the ability to declare transforms in
your tsconfig.json
.
Example:
{
"compilerOptions": {
"plugins": [
{
"transform": "@lit/ts-transformers",
"import": "preserveBlankLinesTransformer"
},
{
"transform": "@lit/ts-transformers",
"import": "idiomaticDecoratorsTransformer"
},
{
"transform": "@lit/ts-transformers",
"import": "constructorCleanupTransformer",
"after": true
}
]
}
}
If preserveBlankLinesTransformer
is used, one way to remove blank line
placeholder comments is with sed:
sed -i $'s/\s*\/\/__BLANK_LINE_PLACEHOLDER_G1JVXUEBNCL6YN5NFE13MD1PT3H9OIHB__/\\\n/g' lib/*.js lib/**/*.js
@rollup/plugin-typescript is a Rollup plugin for compiling TypeScript that includes support for transformers.
Example:
import typescript from '@rollup/plugin-typescript';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import {
idiomaticDecoratorsTransformer,
preserveBlankLinesTransformer,
constructorCleanupTransformer,
BLANK_LINE_PLACEHOLDER_COMMENT,
} from '@lit/ts-transformers';
export default {
input: './src/my-element.ts',
plugins: [
typescript({
transformers: {
before: [
// Optionally preserve blank lines for better readability.
{factory: preserveBlankLinesTransformer},
// Transform Lit decorators to idiomatic vanilla JavaScript.
{type: 'program', factory: idiomaticDecoratorsTransformer},
],
after: [
// Optional readability improvements for constructors.
{type: 'program', factory: constructorCleanupTransformer},
],
},
}),
// Only for when using preserveBlankLinesTransformer.
replace({
values: {
[`//${BLANK_LINE_PLACEHOLDER_COMMENT}`]: '',
},
delimiters: ['', ''],
}),
resolve(),
],
output: {
file: 'dist/my-element.js',
format: 'esm',
},
};
FAQs
TypeScript transformers for Lit
The npm package @lit/ts-transformers receives a total of 59 weekly downloads. As such, @lit/ts-transformers popularity was classified as not popular.
We found that @lit/ts-transformers demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 9 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.