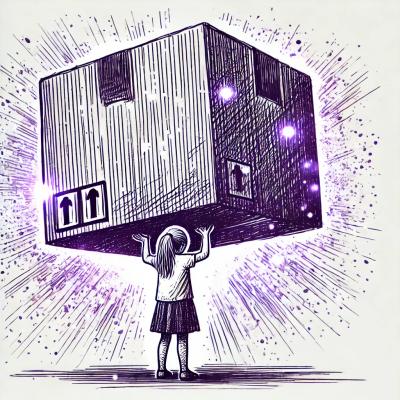
Security News
The Unpaid Backbone of Open Source: Solo Maintainers Face Increasing Security Demands
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
@littlemissrobot/sass-breakpoints
Advanced tools
Little Miss Robot breakpoints setup for defining breakpoints and applying media queries
This repository contains logic and functionality to define and insert breakpoint based media queries through the use of a configuration.
This package does not contain or generate any CSS. It simply provides a system
with @function
and @mixin
statement to manage breakpoints. It is part of the
@littlemissrobot/sass-system package.
This library makes use of Dart Sass, which is the primary implementation of Sass. Make sure that your Sass compiler is making use of Dart Sass.
This means that if you are using a task manager (like Gulp) or a module bundler (like Webpack), you must indicate which compiler it should use for compiling Sass to CSS.
Furthermore, this library makes heavy use of Sass modules:
@use
. Therefore we
recommend importing and using this library with the @use
statement.
# As a dependency
$ npm install @littlemissrobot/sass-breakpoints
# As a dev-dependency
$ npm install --save-dev @littlemissrobot/sass-breakpoints
@use "YOUR-PATH-TO-NODE_MODULES/@littlemissrobot/sass-breakpoints" as _breakpoints;
// Dependency
@use "YOUR-PATH-TO-NODE_MODULES/@littlemissrobot/sass-spacing" as _spacing with (
$base-font-size: 16px
);
// Library
@use "YOUR-PATH-TO-NODE_MODULES/@littlemissrobot/sass-breakpoints" as _breakpoints with (
$viewports: (
viewport-3: 360px,
viewport-4: 480px,
viewport-7: 720px,
viewport-9: 992px,
viewport-12: 1200px
)
);
_breakpoints
.The main focus of this library is to create consistent usage of breakpoints. Each breakpoint should have a name and a width in pixels when it should get triggered.
Naming the breakpoints can be contextual like mobile, tablet, desktop. But this limits the amount of breakpoints we can create. Here we try avoiding using $desktop-small, $desktop-small-x, ... because these are difficult to maintain.
We recommend naming breakpoints after the hundreth they belong too. For example:
We recommend staying with just one value every hundreth. This is done to avoid very small gaps and inconsistencies.
The eventual breakpoint is placed in converted and placed in em
. This is done
because this is the only value consistent in every
browser
As a dependency, @littlemissrobot/sass-spacing, is used to calculate the breakpoint value in em.
// Dependency
@use "YOUR-PATH-TO-NODE_MODULES/@littlemissrobot/sass-spacing" as _spacing with (
$base-font-size: 16px
);
// Library
@use "YOUR-PATH-TO-NODE_MODULES/@littlemissrobot/sass-breakpoints" as _breakpoints with (
$viewports: (
viewport-3: 360px,
viewport-4: 480px,
viewport-7: 720px,
viewport-9: 992px,
viewport-12: 1200px
)
);
$viewports: (
viewport-0: 0px,
viewport-3: 360px,
viewport-4: 480px,
viewport-7: 720px,
viewport-9: 992px,
viewport-12: 1200px,
);
The $viewports
is a sass map where every key is the name of the viewport name
and the value is its width when it should get triggered.
Apply a breakpoint, to every element and prop above the given minimum width of the breakpoint.
Parameters:
$value: the minimum width, when below this width, the styling is not applied. The value can either be:
@use "@littlemissrobot/sass-breakpoints" as _breakpoints with (
$viewports: (
viewport-7: 720px
)
);
body {
// Name of the breakpoint
@include _breakpoints.at("viewport-7") {
background-color: blue;
}
// Number in pixels
@include _breakpoints.at(720px) {
background-color: blue;
}
}
// Name of the breakpoint
@include _breakpoints.at("viewport-7") {
body {
background-color: blue;
}
}
// Number in pixels
@include _breakpoints.at(720px) {
body {
background-color: blue;
}
}
Apply a breakpoint, to every element and prop below the given maximum width of the breakpoint.
Parameters:
$value: the maximum width, when above this width, the styling is not applied. The value can either be:
@use "@littlemissrobot/sass-breakpoints" as _breakpoints with (
$viewports: (
viewport-7: 720px
)
);
body {
// Name of the breakpoint
@include _breakpoints.to("viewport-7") {
background-color: blue;
}
// Number in pixels
@include _breakpoints.to(720px) {
background-color: blue;
}
}
// Name of the breakpoint
@include _breakpoints.to("viewport-7") {
body {
background-color: blue;
}
}
// Number in pixels
@include _breakpoints.to(720px) {
body {
background-color: blue;
}
}
Apply a breakpoint, to every element and prop between the given minimum and maximum width of the breakpoint.
Parameters:
$min: the minimum width, when below this width, the styling is not applied. The value can either be:
$max: the maximum width, when above this width, the styling is not applied. The value can either be:
@use "@littlemissrobot/sass-breakpoints" as _breakpoints with (
$viewports: (
viewport-7: 720px,
viewport-9: 992px
)
);
body {
// Name of the breakpoint
@include _breakpoints.between("viewport-7", "viewport-9") {
background-color: blue;
}
// Number in pixels
@include _breakpoints.between(720px, 992px) {
background-color: blue;
}
}
// Name of the breakpoint
@include _breakpoints.between("viewport-7", "viewport-9") {
body {
background-color: blue;
}
}
// Number in pixels
@include _breakpoints.between(720px, 992px) {
body {
background-color: blue;
}
}
Create a class that is suffixed with one of the viewports defined in the
$viewports
variable. This declaration will apply styles until a breakpoint is
reached.
Parameters:
$at: A list of names from $viewports variable to apply the class declaration to. This declaration will be applied if the viewport is above the breakpoint.
$at: A list of names from $viewports variable to apply the class declaration to. This declaration will be applied if the viewport is below the breakpoint.
@use "@littlemissrobot/sass-breakpoints" as _breakpoints with (
$viewports: (
viewport-4: 480px,
viewport-7: 720px,
viewport-9: 992px,
viewport-12: 1200px
)
);
.u-hide {
@include _breakpoints.suffixicate(
$at: (viewport-7, viewport-9),
$to: (viewport-4, viewport-12)
) {
display: none !important;
}
}
The example above will generate:
FAQs
Little Miss Robot breakpoints setup for defining breakpoints and applying media queries.
The npm package @littlemissrobot/sass-breakpoints receives a total of 34 weekly downloads. As such, @littlemissrobot/sass-breakpoints popularity was classified as not popular.
We found that @littlemissrobot/sass-breakpoints demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.
Security News
A developer is accusing Tencent of violating the GPL by modifying a Python utility and changing its license to BSD, highlighting the importance of copyleft compliance.