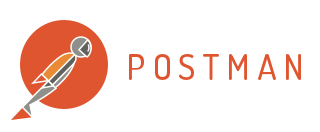
Supercharge your API workflow.
Modern software is built on APIs. Postman helps you develop APIs faster.
OpenAPI 3.0 to Postman Collection v2.1.0 Converter

Contents
- Getting Started
- Using the converter as a NodeJS module
- Convert Function
- Options
- ConversionResult
- Sample usage
- Validate function
- Command Line Interface
- Options
- Usage
- Conversion Schema
Getting Started
To use the converter as a Node module, you need to have a copy of the NodeJS runtime. The easiest way to do this is through npm. If you have NodeJS installed you have npm installed as well.
$ npm install openapi-to-postmanv2
Using the converter as a NodeJS module
In order to use the convert in your node application, you need to import the package using require
.
var Converter = require('openapi-to-postmanv2')
The converter provides the following functions:
Convert
The convert function takes in your OpenAPI specification ( YAML / JSON ) and converts it to a Postman collection.
Signature: convert (data, options, callback);
data:
{ type: 'file', data: 'filepath' }
OR
{ type: 'string', data: '<entire OpenAPI string - JSON or YAML>' }
OR
{ type: 'json', data: OpenAPI-JS-object }
options:
{
schemaFaker: true,
requestNameSource: 'fallback',
indentCharacter: ' '
}
callback:
function (err, result) {
}
Options:
'schemaFaker'(boolean)
: whether to use json-schema-faker for schema conversion. Default: true
'requestNameSource'(string)
: The strategy to use to generate request names. url: use the request's URL as the name, fallback: Use the summary/operationId/URL (in that order) Default: fallback
'indentCharacter' (string)
: The character to use per level of indentation for JSON/XML data. Default: ' '(space)
ConversionResult
-
result
- Flag responsible for providing a status whether the conversion was successful or not
-
reason
- Provides the reason for an unsuccessful conversion, defined only if result: false
-
output
- Contains an array of Postman objects, each one with a type
and data
. The only type currently supported is collection
.
Sample Usage:
var fs = require('fs'),
Converter = require('openapi-to-postmanv2'),
openapiData = fs.readFileSync('sample-spec.yaml', {encoding: 'UTF8'});
Converter.convert({ type: 'string', data: openapiData },
{}, (err, conversionResult) => {
if (!conversionResult.result) {
console.log('Could not convert', conversionResult.reason);
}
else {
console.log('The collection object is: ', conversionResult.output[0].data);
}
}
);
Validate Function
The validate function is meant to ensure that the data that is being passed to the convert function is a valid JSON object or a valid (YAML/JSON) string.
The validate function is synchronous and returns a status object which conforms to the following schema
Validation object schema
{
type: 'object',
properties: {
result: { type: 'boolean'},
reason: { type: 'string' }
},
required: ['result']
}
Validation object explanation
Command Line Interface
The converter can be used as a CLI tool as well. The following command line options are available.
openapi2postmanv2 [options]
Options
-
-V
, --version
Specifies the version of the converter
-
-s <source>
, --spec <source>
Used to specify the OpenAPI specification (file path) which is to be converted
-
-o <destination>
, --output <destination>
Used to specify the destination file in which the collection is to be written
-
-t
, --test
Used to test the collection with an in-built sample specification
-
-p
, --pretty
Used to pretty print the collection object while writing to a file
-
-h
, --help
Specifies all the options along with a few usage examples on the terminal
Usage
Sample usage examples of the converter CLI
- Takes a specification (spec.yaml) as an input and writes to a file (collection.json) with pretty printing
$ openapi2postmanv2 -s spec.yaml -o collection.json -p
$ openapi2postmanv2 --test
Conversion Schema
postman | openapi | options | examples |
---|
collectionName | info.title | - | |
description | info.description + info.contact | - | |
collectionVariables | server.variables + pathVariables | - | |
folderName | paths.path | - | |
requestName | operationItem(method).operationId | default(operationId)-(requestName )enum['operationId','summary','url'] | |
request.method | path.method | - | |
request.headers | parameter (in = header ) | - | link |
request.body | operationItem(method).requestBody | - | |
request.url.raw | server.url (path level server >> openapi server) + path | - | |
request.url.variables | parameter (in = path ) | - | link |
request.url.params | parameter (in = query ) | - | {"key": param.name, "value": link} |
api_key in (query or header) | components.securitySchemes.api_key | - | |