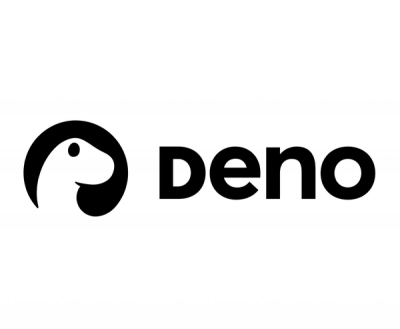
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@mapbox/magic-cfn-resources
Advanced tools
Builds Lambda-backed custom Cloudformation resources. When you use magic-cfn-resources
's build
method, a Lambda function is created in your stack and used to build a custom resource. Resources that can be built with magic-cfn-resources
are: SnsSubscription
, DynamoDBStreamLabel
, StackOutputs
, and SpotFleet
.
This allows you to manage SNS subscriptions as though they are first-class CloudFormation resources.
This does not actually create any backend resource, but looks up the label for the stream associated with a DynamoDB table.
This resource will use the stream's label as its PhysicalResourceId, so you can then access the label itself in your template via:
{ "Ref": "LogicalNameOfYourCustomResource" }
Looks up the Outputs for an existing CloudFormation stack.
You can access the values of the stack's outputs with Fn::GetAtt
{ "Fn::GetAtt": ["LogicalNameOfYourCustomResource", "LogicalNameOfStackOutput"] }
Makes SpotFleet requests.
Looks up the default VPC in the region you've launched your stack in, and provides information about the VPC via Fn::GetAtt
.
{ "Fn::GetAtt": ["LogicalNameOfYourCustomResource", "VpcId"] }
You can use Fn::GetAtt
to obtain the following data:
sns-subscription.js
):// Purpose: create a handler for your Lambda function to reference
const magicCfnResources = require('@mapbox/magic-cfn-resources');
// export the custom function needed for your stack.
module.exports.SnsSubscription = magicCfnResources.SnsSubscription;
Another example: module.exports.SpotFleet = magicCfnResources.SpotFleet;
const magicCfnResources = require('@mapbox/magic-cfn-resources');
magicCfnResources.build
. These are the parameters needed for each resource:SnsSubscription
const SnsSubscription = magicCfnResources.build({
CustomResourceName: 'SnsSubscription',
LogicalName: 'Logical Name', // a name to refer to the custom resource being built
S3Bucket: 'Bucket Name', // the S3 bucket the code for the handler lives in
S3Key: 'Key', // the S3 key for where the handler lives
Handler: 'sns-subscription.SnsSubscription', // references the handler created in the repository
Properties: {
SnsTopicArn: 'Topic Arn', // the ARN of the SNS Topic you are subscribing to
Protocol: 'Protocol', // the SNS protocol, i.e. 'sqs', 'email'
Endpoint: 'Endpoint' // the endpoint you are subscribing
}
});
DynamoDBStreamLabel
const DynamoDBStreamLabel = magicCfnResources.build({
CustomResourcenName: 'DynamoDBStreamLabel',
LogicalName: 'Logical Name', // a name to refer to the custom resource being built
S3Bucket: 'Bucket Name', // the S3 bucket the code for the handler lives in
S3Key: 'Key', // the S3 key for where the handler lives
Handler: 'dynamodb-stream-label.DynamoDBStreamLabel', // references the handler created in the repository
Properties: {
TableName: 'Name of Table', // the name of the DynamoDB table
TableRegion: 'Region' // the region of the DynamoDB table i.e.: 'us-east-1'
}
});
StackOutputs
const StackOutputs = magicCfnResources.build({
CustomResourceName: 'StackOutputs',
LogicalName: 'Logical Name', // a name to refer to the custom resource being built
S3Bucket: 'Bucket Name', // the S3 bucket the code for the handler lives in
S3Key: 'Key', // the S3 key for where the handler lives
Handler: 'stack-outputs.StackOutputs', // references the handler created in the repository
Properties: {
StackName: 'Name', // name of the CloudFormation stack
StackRegion: 'region' // region of the CloudFormation stack i.e.: 'us-east-1'
}
});
SpotFleet
const SpotFleet = magicCfnResources.build({
CustomResourceName: 'SpotFleet',
LogicalName: 'Logical Name', // a name to refer to the custom resource being built
S3Bucket: 'Bucket Name', // the S3 bucket the code for the handler lives in
S3Key: 'Key', // the S3 key for where the handler lives
Handler: 'spot-fleet.SpotFleet', // references the handler created in the repository
Properties: {
SpotFleetRequestConfigData: { }, // object with SpotFleet configuration specifics
Region: 'region', // region of the SpotFleet i.e.: 'us-east-1'
}
});
DefaultVpc
const DefaultVpd = magicCfnResources.build({
CustomResourceName: 'DefaultVpc',
LogicalName: 'Logical Name', // a name to refer to the custom resource being built
S3Bucket: 'Bucket Name', // the S3 bucket the code for the handler lives in
S3Key: 'Key', // the S3 key for where the handler lives
Handler: 'index.DefaultVpc', // references the handler created in the repository
Properties: {}
});
A Condition from your template can also be passed into build
.
i.e.:
const SpotFleet = magicCfnResources.build({
CustomResourceName: 'SpotFleet',
LogicalName: 'Logical Name', // a name to refer to the custom resource being built
S3Bucket: 'Bucket Name', // the S3 bucket the code for the handler lives in
S3Key: 'Key', // the S3 key for where the handler lives
Handler: 'spot-fleet.SpotFleet', // references the handler created in the repository
Properties: {
SpotFleetRequestConfigData: { }, // object with SpotFleet configuration specifics
Region: 'region', // region of the SpotFleet i.e.: 'us-east-1'
},
Condition: 'Condition' // the Logical ID of a condition
});
build
with the resources already in the stack's template:. i.e.:const cloudfriend = require('@mapbox/cloudfriend');
const magicCfnResources = require('@mapbox/magic-cfn-resources');
module.exports = cloudfriend.merge(SnsSubscription, <Stack Resources>);
Check out contributing.md for a discussion of the framework this library provides for writing other functions.
1.3.0
Added default-vpc
resource
FAQs
Build Lambda-backed custom CloudFormation resources
The npm package @mapbox/magic-cfn-resources receives a total of 4 weekly downloads. As such, @mapbox/magic-cfn-resources popularity was classified as not popular.
We found that @mapbox/magic-cfn-resources demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 28 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.