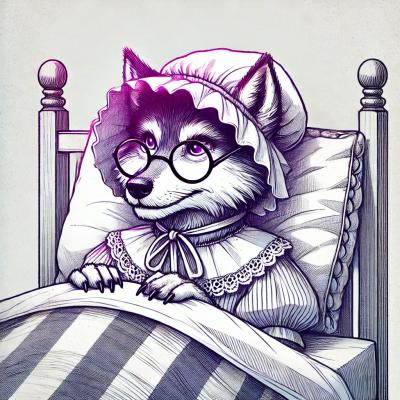
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@material/slider
Advanced tools
The Material Components for the web slider component
@material/slider is a Material Design slider component for the web. It provides a range of features for creating sliders that are consistent with the Material Design guidelines. This package allows developers to create sliders with various configurations, including discrete and continuous sliders, and provides customization options for styling and behavior.
Basic Slider
This code sample demonstrates how to create a basic slider using the @material/slider package. The slider is initialized and an event listener is added to log the value whenever it changes.
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="https://unpkg.com/@material/slider/dist/mdc.slider.css">
<script src="https://unpkg.com/@material/slider/dist/mdc.slider.js"></script>
</head>
<body>
<div class="mdc-slider" tabindex="0" role="slider" aria-valuemin="0" aria-valuemax="100" aria-valuenow="50" aria-label="Select Value">
<div class="mdc-slider__track-container">
<div class="mdc-slider__track"></div>
</div>
<div class="mdc-slider__thumb-container">
<div class="mdc-slider__pin">
<span class="mdc-slider__pin-value-marker"></span>
</div>
<svg class="mdc-slider__thumb" width="21" height="21">
<circle cx="10.5" cy="10.5" r="7.875"></circle>
</svg>
<div class="mdc-slider__focus-ring"></div>
</div>
</div>
<script>
const slider = new mdc.slider.MDCSlider(document.querySelector('.mdc-slider'));
slider.listen('MDCSlider:change', () => console.log(`Value changed to ${slider.value}`));
</script>
</body>
</html>
Discrete Slider with Tick Marks
This code sample demonstrates how to create a discrete slider with tick marks using the @material/slider package. The slider is configured to display discrete values and markers along the track.
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="https://unpkg.com/@material/slider/dist/mdc.slider.css">
<script src="https://unpkg.com/@material/slider/dist/mdc.slider.js"></script>
</head>
<body>
<div class="mdc-slider mdc-slider--discrete mdc-slider--display-markers" tabindex="0" role="slider" aria-valuemin="0" aria-valuemax="100" aria-valuenow="50" aria-label="Select Value">
<div class="mdc-slider__track-container">
<div class="mdc-slider__track"></div>
<div class="mdc-slider__track-marker-container"></div>
</div>
<div class="mdc-slider__thumb-container">
<div class="mdc-slider__pin">
<span class="mdc-slider__pin-value-marker"></span>
</div>
<svg class="mdc-slider__thumb" width="21" height="21">
<circle cx="10.5" cy="10.5" r="7.875"></circle>
</svg>
<div class="mdc-slider__focus-ring"></div>
</div>
</div>
<script>
const slider = new mdc.slider.MDCSlider(document.querySelector('.mdc-slider'));
slider.listen('MDCSlider:change', () => console.log(`Value changed to ${slider.value}`));
</script>
</body>
</html>
Range Slider
This code sample demonstrates how to create a range slider using the @material/slider package. The range slider allows users to select a range of values within a specified minimum and maximum.
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="https://unpkg.com/@material/slider/dist/mdc.slider.css">
<script src="https://unpkg.com/@material/slider/dist/mdc.slider.js"></script>
</head>
<body>
<div class="mdc-slider mdc-slider--range" tabindex="0" role="slider" aria-valuemin="0" aria-valuemax="100" aria-valuenow="50" aria-label="Select Value">
<div class="mdc-slider__track-container">
<div class="mdc-slider__track"></div>
</div>
<div class="mdc-slider__thumb-container">
<div class="mdc-slider__pin">
<span class="mdc-slider__pin-value-marker"></span>
</div>
<svg class="mdc-slider__thumb" width="21" height="21">
<circle cx="10.5" cy="10.5" r="7.875"></circle>
</svg>
<div class="mdc-slider__focus-ring"></div>
</div>
<div class="mdc-slider__thumb-container">
<div class="mdc-slider__pin">
<span class="mdc-slider__pin-value-marker"></span>
</div>
<svg class="mdc-slider__thumb" width="21" height="21">
<circle cx="10.5" cy="10.5" r="7.875"></circle>
</svg>
<div class="mdc-slider__focus-ring"></div>
</div>
</div>
<script>
const slider = new mdc.slider.MDCSlider(document.querySelector('.mdc-slider'));
slider.listen('MDCSlider:change', () => console.log(`Value changed to ${slider.value}`));
</script>
</body>
</html>
rc-slider is a React component for creating sliders. It offers a wide range of customization options and supports both single and range sliders. Compared to @material/slider, rc-slider is more focused on React applications and provides more flexibility in terms of customization and styling.
nouislider is a lightweight JavaScript range slider with full touch support. It is highly customizable and supports a wide range of features, including tooltips, pips, and more. Compared to @material/slider, nouislider is more versatile and can be used in various frameworks and environments, not just Material Design.
react-slider is a small, accessible slider component for React. It is designed to be simple and easy to use, with a focus on accessibility. Compared to @material/slider, react-slider is more lightweight and specifically tailored for React applications, making it a good choice for projects that prioritize accessibility and simplicity.
Sliders allow users to make selections from a range of values.
The MDC Slider implementation supports both single point sliders (one thumb)
and range sliders (two thumbs). It is backed by the browser
<input type="range">
element, is fully accessible, and is RTL-aware.
Contents
npm install @material/slider
@use "@material/slider/styles";
import {MDCSlider} from '@material/slider';
const slider = new MDCSlider(document.querySelector('.mdc-slider'));
Note: See Importing the JS component for more information on how to import JavaScript.
Sliders are backed by an <input>
element, meaning that they are fully
accessible. Unlike the ARIA-based slider,
MDC sliders are adjustable using touch-based assistive technologies such as
TalkBack on Android.
Per the spec, ensure that the following attributes are added to the
input
element(s):
value
: Value representing the current value.min
: Value representing the minimum allowed value.max
: Value representing the maximum allowed value.aria-label
or aria-labelledby
: Accessible label for the slider.If the value is not user-friendly (e.g. a number to represent the day of the week), also set the following:
aria-valuetext
: Set this input attribute to a string that makes the slider
value understandable, e.g. 'Monday'.aria-valuetext
via the
MDCSlider#setValueToAriaValueTextFn
method.There are two types of sliders:
Continuous sliders allow users to make meaningful selections that don’t require a specific value.
Note: The step size for value quantization is, by default, 1. To specify
a custom step size, provide a value for the step
attribute on the input
element.
<div class="mdc-slider">
<div class="mdc-slider__track">
<div class="mdc-slider__track--inactive"></div>
<div class="mdc-slider__track--active">
<div class="mdc-slider__track--active_fill"></div>
</div>
</div>
<div class="mdc-slider__thumb">
<div class="mdc-slider__thumb-knob"></div>
<input class="mdc-slider__input" type="range" min="0" max="100" value="50" name="volume" aria-label="Continuous slider demo">
</div>
</div>
Note: By default there's no minimum distance between the two thumbs. To specify
one, provide a value for the data-min-range
attribute on the root element and
adjust the min
and max
attributes on the input elements accordingly.
<div class="mdc-slider mdc-slider--range" data-min-range="10">
<div class="mdc-slider__track">
<div class="mdc-slider__track--inactive"></div>
<div class="mdc-slider__track--active">
<div class="mdc-slider__track--active_fill"></div>
</div>
</div>
<div class="mdc-slider__thumb">
<div class="mdc-slider__thumb-knob"></div>
<input class="mdc-slider__input" type="range" min="0" max="60" value="30" name="rangeStart" aria-label="Continuous range slider demo">
</div>
<div class="mdc-slider__thumb">
<div class="mdc-slider__thumb-knob"></div>
<input class="mdc-slider__input" type="range" min="40" max="100" value="70" name="rangeEnd" aria-label="Continuous range slider demo">
</div>
</div>
Discrete sliders display a numeric value label upon pressing the thumb, which allows a user to select an exact value.
To create a discrete slider, add the following:
mdc-slider--discrete
class on the root element.mdc-slider__value-indicator-container
), as shown
below.<div class="mdc-slider mdc-slider--discrete">
<div class="mdc-slider__track">
<div class="mdc-slider__track--inactive"></div>
<div class="mdc-slider__track--active">
<div class="mdc-slider__track--active_fill"></div>
</div>
</div>
<div class="mdc-slider__thumb">
<div class="mdc-slider__value-indicator-container" aria-hidden="true">
<div class="mdc-slider__value-indicator">
<span class="mdc-slider__value-indicator-text">
50
</span>
</div>
</div>
<div class="mdc-slider__thumb-knob"></div>
<input class="mdc-slider__input" type="range" min="0" max="100" value="50" name="volume" step="10" aria-label="Discrete slider demo">
</div>
</div>
Discrete sliders can optionally display tick marks. Tick marks represent predetermined values to which the user can move the slider.
To add tick marks to a discrete slider, add the following:
mdc-slider--tick-marks
class on the root elementmdc-slider__tick-marks
element as a child of the mdc-slider__track
elementmdc-slider__tick-mark--active
and mdc-slider__tick-mark--inactive
elements as children of the mdc-slider__tick-marks
element<div class="mdc-slider mdc-slider--discrete mdc-slider--tick-marks">
<div class="mdc-slider__track">
<div class="mdc-slider__track--inactive"></div>
<div class="mdc-slider__track--active">
<div class="mdc-slider__track--active_fill"></div>
</div>
<div class="mdc-slider__tick-marks">
<div class="mdc-slider__tick-mark--active"></div>
<div class="mdc-slider__tick-mark--active"></div>
<div class="mdc-slider__tick-mark--active"></div>
<div class="mdc-slider__tick-mark--active"></div>
<div class="mdc-slider__tick-mark--active"></div>
<div class="mdc-slider__tick-mark--active"></div>
<div class="mdc-slider__tick-mark--inactive"></div>
<div class="mdc-slider__tick-mark--inactive"></div>
<div class="mdc-slider__tick-mark--inactive"></div>
<div class="mdc-slider__tick-mark--inactive"></div>
<div class="mdc-slider__tick-mark--inactive"></div>
</div>
</div>
<div class="mdc-slider__thumb">
<div class="mdc-slider__value-indicator-container" aria-hidden="true">
<div class="mdc-slider__value-indicator">
<span class="mdc-slider__value-indicator-text">
50
</span>
</div>
</div>
<div class="mdc-slider__thumb-knob"></div>
<input class="mdc-slider__input" type="range" min="0" max="100" value="50" name="volume" step="10" aria-label="Discrete slider with tick marks demo">
</div>
</div>
<div class="mdc-slider mdc-slider--range mdc-slider--discrete">
<div class="mdc-slider__track">
<div class="mdc-slider__track--inactive"></div>
<div class="mdc-slider__track--active">
<div class="mdc-slider__track--active_fill"></div>
</div>
</div>
<div class="mdc-slider__thumb">
<div class="mdc-slider__value-indicator-container" aria-hidden="true">
<div class="mdc-slider__value-indicator">
<span class="mdc-slider__value-indicator-text">
20
</span>
</div>
</div>
<div class="mdc-slider__thumb-knob"></div>
<input class="mdc-slider__input" type="range" min="0" max="50" value="20" step="10" name="rangeStart" aria-label="Discrete range slider demo">
</div>
<div class="mdc-slider__thumb">
<div class="mdc-slider__value-indicator-container" aria-hidden="true">
<div class="mdc-slider__value-indicator">
<span class="mdc-slider__value-indicator-text">
50
</span>
</div>
</div>
<div class="mdc-slider__thumb-knob"></div>
<input class="mdc-slider__input" type="range" min="20" max="100" value="50" step="10" name="rangeEnd" aria-label="Discrete range slider demo">
</div>
</div>
To disable a slider, add the following:
mdc-slider--disabled
class on the root elementdisabled
attribute on the input element<div class="mdc-slider mdc-slider--disabled">
<div class="mdc-slider__track">
<div class="mdc-slider__track--inactive"></div>
<div class="mdc-slider__track--active">
<div class="mdc-slider__track--active_fill"></div>
</div>
</div>
<div class="mdc-slider__thumb">
<div class="mdc-slider__thumb-knob"></div>
<input class="mdc-slider__input" type="range" min="0" max="100" value="50" step="10" disabled name="volume" aria-label="Disabled slider demo">
</div>
</div>
When MDCSlider
is initialized, it reads the input element's min
,
max
, and value
attributes if present, using them to set
the component's internal min
, max
, and value
properties.
Use these attributes to initialize the slider with a custom range and values, as shown below:
<div class="mdc-slider">
<!-- ... -->
<div class="mdc-slider__thumb">
<!-- ... -->
<input class="mdc-slider__input" aria-label="Slider demo" min="0" max="100" value="75">
</div>
</div>
When MDCSlider
is initialized, it updates the slider track and thumb
positions based on the internal value(s). To set the correct track and thumb
positions before component initialization, mark up the DOM as follows:
rangePercentDecimal
, the active track range as a percentage of
the entire track, i.e. (valueEnd - valueStart) / (max - min)
.
Set transform:scaleX(<rangePercentDecimal>)
as an inline style on the
mdc-slider__track--active_fill
element.thumbEndPercent
, the initial position of the end thumb as a
percentage of the entire track. Set left:calc(<thumbEndPercent>% - 24px)
as an inline style on the end thumb (mdc-slider__thumb
) element
(or right
for RTL layouts).thumbStartPercent
, the initial position
of the start thumb as a percentage of the entire track. Set
left:calc(<thumbStartPercent>% - 24px)
as an inline style on the
start thumb (mdc-slider__thumb
) element (or right
for RTL layouts).thumbStartPercent
,
set left:<thumbStartPercent>%
as an inline style on the
mdc-slider__track--active_fill
element (or right
for RTL layouts).Additionally, the MDCSlider component should be initialized with
skipInitialUIUpdate
set to true.
This is an example of a range slider with internal values of
[min, max] = [0, 100]
and [start, end] = [30, 70]
, and a minimum range of
10.
<div class="mdc-slider mdc-slider--range" data-min-range="10">
<div class="mdc-slider__track">
<div class="mdc-slider__track--inactive"></div>
<div class="mdc-slider__track--active">
<div class="mdc-slider__track--active_fill"
style="transform:scaleX(.4); left:30%"></div>
</div>
</div>
<div class="mdc-slider__thumb" style="left:calc(30%-24px)">
<div class="mdc-slider__thumb-knob"></div>
<input class="mdc-slider__input" type="range" min="0" max="60" value="30" name="rangeStart" aria-label="Range slider demo">
</div>
<div class="mdc-slider__thumb" style="left:calc(70%-24px)">
<div class="mdc-slider__thumb-knob"></div>
<input class="mdc-slider__input" type="range" min="40" max="100" value="70" name="rangeEnd" aria-label="Range slider demo">
</div>
</div>
Mixin | Description |
---|---|
track-active-color($color) | Sets the color of the active track. |
track-inactive-color($color, $opacity) | Sets the color and opacity of the inactive track. |
thumb-color($color) | Sets the color of the thumb. |
thumb-ripple-color($color) | Sets the color of the thumb ripple. |
tick-mark-active-color($color) | Sets the color of tick marks on the active track. |
tick-mark-inactive-color($color) | Sets the color of tick marks on the inactive track. |
value-indicator-color($color, $opaicty) | Sets the color and opacity of the value indicator. |
value-indicator-text-color($color, $opaicty) | Sets the color of the value indicator text. |
MDCSlider
eventsEvent name | event.detail | Description |
---|---|---|
MDCSlider:change | MDCSliderChangeEventDetail | Emitted when a value has been changed and committed from a user event. Mirrors the native change event: https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/change_event |
MDCSlider:input | MDCSliderChangeEventDetail | Emitted when a value has been changed from a user event. Mirrors the native input event: https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/input_event |
MDCSlider
methodsMethod Signature | Description |
---|---|
getValueStart() => number | Gets the value of the start thumb (only applicable for range sliders). |
setValueStart(valueStart: number) => void | Sets the value of the start thumb (only applicable for range sliders). |
getValue() => number | Gets the value of the thumb (for single point sliders), or the end thumb (for range sliders). |
setValue(value: number) => void | Sets the value of the thumb (for single point sliders), or the end thumb (for range sliders). |
getDisabled() => boolean | Gets the disabled state of the slider. |
setDisabled(disabled: boolean) => void | Sets the disabled state of the slider. |
setValueToAriaValueTextFn((mapFn: ((value: number) => string) | null) => void | Sets a function that maps the slider value to value of the aria-valuetext attribute on the thumb element. If not set, the aria-valuetext attribute is unchanged when the value changes. |
If you are using a JavaScript framework such as React or Angular, you can create a slider for your framework. Depending on your needs, you can use the Simple Approach: Wrapping MDC Web Vanilla Components, or the Advanced Approach: Using Foundations and Adapters. Please follow the instructions here.
See MDCSliderAdapter and MDCSliderFoundation for up-to-date code documentation of slider foundation API's.
FAQs
The Material Components for the web slider component
We found that @material/slider demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 15 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.