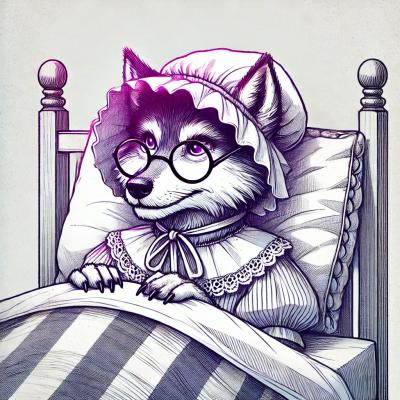
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@material/tab-indicator
Advanced tools
The Material Components for the web tab indicator component
@material/tab-indicator is a package from Material Design Components (MDC) that provides a visual indicator for tabs. It is used to highlight the active tab in a tab bar, enhancing the user experience by clearly showing which tab is currently selected.
Basic Tab Indicator
This code snippet demonstrates how to create a basic tab indicator with an underline style. The `mdc-tab-indicator` class is used to define the tab indicator, and the `mdc-tab-indicator__content` class with the `mdc-tab-indicator__content--underline` modifier is used to style the indicator as an underline.
```html
<div class="mdc-tab-indicator">
<span class="mdc-tab-indicator__content mdc-tab-indicator__content--underline"></span>
</div>
```
Icon Tab Indicator
This code snippet shows how to create a tab indicator with an icon. The `mdc-tab-indicator__content--icon` modifier is used to style the indicator with an icon, and the `material-icons` class is used to specify the icon.
```html
<div class="mdc-tab-indicator">
<span class="mdc-tab-indicator__content mdc-tab-indicator__content--icon">
<i class="material-icons">star</i>
</span>
</div>
```
Sliding Tab Indicator
This code snippet demonstrates how to create a sliding tab indicator. The `mdc-tab-indicator--active` class is used to activate the sliding animation for the tab indicator.
```html
<div class="mdc-tab-indicator mdc-tab-indicator--active">
<span class="mdc-tab-indicator__content mdc-tab-indicator__content--underline"></span>
</div>
```
react-tabs is a package for creating accessible and customizable tab components in React. It provides a simple API for creating tabbed interfaces and includes features like keyboard navigation and ARIA attributes. Compared to @material/tab-indicator, react-tabs is more focused on accessibility and React integration.
Bootstrap is a popular front-end framework that includes a wide range of components, including tabs. Bootstrap's tab component provides a simple way to create tabbed interfaces with various styles and animations. While @material/tab-indicator is specifically for Material Design, Bootstrap offers a more general-purpose solution with a different design aesthetic.
semantic-ui-react is the official React integration for Semantic UI, a UI framework that provides a variety of components, including tabs. The tab component in semantic-ui-react is highly customizable and integrates well with React applications. Compared to @material/tab-indicator, semantic-ui-react offers a broader range of UI components and a different design philosophy.
A Tab Indicator is a visual guide that shows which Tab is active.
npm install @material/tab-indicator
<span class="mdc-tab-indicator">
<span class="mdc-tab-indicator__content"></span>
</span>
@use "@material/tab-indicator/mdc-tab-indicator";
import {MDCTabIndicator} from '@material/tab-indicator';
const tabIndicator = new MDCTabIndicator(document.querySelector('.mdc-tab-indicator'));
See Importing the JS component for more information on how to import JavaScript.
Add the mdc-tab-indicator--active
class to the mdc-tab-indicator
element to make the Tab Indicator active.
The Tab Indicator may be represented in one of two ways:
mdc-tab-indicator__content--underline
classmdc-tab-indicator__content--icon
classNOTE: One of these classes must be applied to the Tab Indicator's content element.
The Tab Indicator may transition in one of two ways:
mdc-tab-indicator--fade
class<span class="mdc-tab-indicator">
<span class="mdc-tab-indicator__content mdc-tab-indicator__content--underline"></span>
</span>
We recommend using Material Icons from Google Fonts:
<head>
<link rel="stylesheet" href="https://fonts.googleapis.com/icon?family=Material+Icons">
</head>
However, you can also use SVG, Font Awesome, or any other icon library you wish.
Remember to include aria-hidden="true"
, since the active indicator is already signified via the
aria-selected
attribute on the tab.
<span class="mdc-tab-indicator mdc-tab-indicator--fade">
<span class="mdc-tab-indicator__content mdc-tab-indicator__content--icon material-icons" aria-hidden="true">star</span>
</span>
<span class="mdc-tab-indicator">
<span class="mdc-tab-indicator__content mdc-tab-indicator__content--icon material-icons" aria-hidden="true">star</span>
</span>
CSS Class | Description |
---|---|
mdc-tab-indicator | Mandatory. Contains the tab indicator content. |
mdc-tab-indicator__content | Mandatory. Denotes the tab indicator content. |
mdc-tab-indicator--active | Optional. Visually activates the indicator. |
mdc-tab-indicator--fade | Optional. Sets up the tab indicator to fade in on activation and fade out on deactivation. |
mdc-tab-indicator__content--underline | Optional. Denotes an underline tab indicator. |
mdc-tab-indicator__content--icon | Optional. Denotes an icon tab indicator. |
NOTE: Exactly one of the
--underline
or--icon
content modifier classes should be present.
To customize the tab indicator, use the following mixins.
Mixin | Description |
---|---|
surface | Mandatory. Must be applied to the parent element of the mdc-tab-indicator . |
underline-color($color) | Customizes the color of the underline. |
icon-color($color) | Customizes the color of the icon subelement. |
underline-height($height) | Customizes the height of the underline. |
icon-height($height) | Customizes the height of the icon subelement. |
underline-top-corner-radius($radius) | Customizes the top left and top right border radius of the underline child element. |
MDCTabIndicator
MethodsMethod Signature | Description |
---|---|
activate(previousIndicatorClientRect?: ClientRect) => void | Activates the tab indicator. |
deactivate() => void | Deactivates the tab indicator. |
computeContentClientRect() => ClientRect | Returns the content element bounding client rect. |
If you are using a JavaScript framework, such as React or Angular, you can create a Tab Indicator for your framework. Depending on your needs, you can use the Simple Approach: Wrapping MDC Web Vanilla Components, or the Advanced Approach: Using Foundations and Adapters. Please follow the instructions here.
MDCTabIndicatorAdapter
Method Signature | Description |
---|---|
addClass(className: string) => void | Adds a class to the root element. |
removeClass(className: string) => void | Removes a class from the root element. |
setContentStyleProperty(property: string, value: string) => void | Sets the style property of the content element. |
computeContentClientRect() => ClientRect | Returns the content element's bounding client rect. |
MDCTabIndicatorFoundation
Method Signature | Description |
---|---|
handleTransitionEnd(evt: Event) => void | Handles the logic for the "transitionend" event on the root element. |
activate(previousIndicatorClientRect?: ClientRect) => void | Activates the tab indicator. |
deactivate() => void | Deactivates the tab indicator. |
computeContentClientRect() => ClientRect | Returns the content element's bounding client rect. |
14.0.0 (2022-04-27)
unset
is unsupported in IE. (f460e23)validateTooltipWithCaretDistances
method. (3e30054)theme-styles
mixin... the value being retreived from the $theme
map and css property name was swapped. The mixin would request font-size
/font-weight
/letter-spacing
from the $theme
map (which expects size
/weight
/tracking
)... so these values would always be null
. (32b3913)attachTo
. (05db65e)showTimeout
is not set (indicating that this tooltip is about to be re-shown). (6ca8b8f)minRange
param to range sliders to request a minimum gap between the two thumbs. (8fffcb5)valueToAriaValueTextFn
and valueToValueIndicatorTextFn
functions are called for. (b6510c8)PiperOrigin-RevId: 444830518
PiperOrigin-RevId: 419837612
FAQs
The Material Components for the web tab indicator component
The npm package @material/tab-indicator receives a total of 0 weekly downloads. As such, @material/tab-indicator popularity was classified as not popular.
We found that @material/tab-indicator demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 15 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.