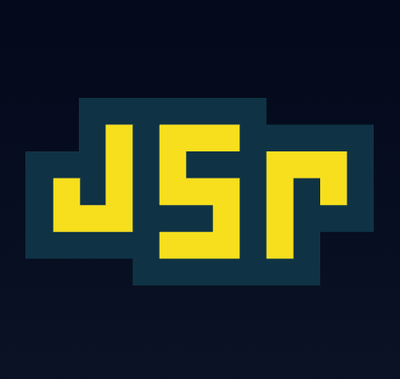
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@metamask/sdk
Advanced tools
@metamask/sdk is a JavaScript library that allows developers to integrate MetaMask functionalities into their web applications. It provides a set of tools to interact with the Ethereum blockchain, manage user accounts, and facilitate transactions.
Connecting to MetaMask
This feature allows you to connect to the MetaMask wallet and request access to the user's Ethereum accounts. The code sample demonstrates how to initialize the MetaMask SDK, get the provider, and request account access.
const { MetaMaskSDK } = require('@metamask/sdk');
const sdk = new MetaMaskSDK();
const ethereum = sdk.getProvider();
async function connect() {
try {
const accounts = await ethereum.request({ method: 'eth_requestAccounts' });
console.log('Connected account:', accounts[0]);
} catch (error) {
console.error('Error connecting to MetaMask:', error);
}
}
connect();
Sending Transactions
This feature allows you to send transactions through the MetaMask wallet. The code sample demonstrates how to create a transaction object and send it using the MetaMask provider.
const { MetaMaskSDK } = require('@metamask/sdk');
const sdk = new MetaMaskSDK();
const ethereum = sdk.getProvider();
async function sendTransaction() {
try {
const transactionParameters = {
to: '0xRecipientAddress',
value: '0x29a2241af62c0000', // 0.1 ETH in hexadecimal
gas: '0x5208', // 21000 GWEI in hexadecimal
};
const txHash = await ethereum.request({
method: 'eth_sendTransaction',
params: [transactionParameters],
});
console.log('Transaction hash:', txHash);
} catch (error) {
console.error('Error sending transaction:', error);
}
}
sendTransaction();
Listening for Events
This feature allows you to listen for events such as account changes and network changes. The code sample demonstrates how to set up event listeners for 'accountsChanged' and 'chainChanged' events.
const { MetaMaskSDK } = require('@metamask/sdk');
const sdk = new MetaMaskSDK();
const ethereum = sdk.getProvider();
ethereum.on('accountsChanged', (accounts) => {
console.log('Accounts changed:', accounts);
});
ethereum.on('chainChanged', (chainId) => {
console.log('Chain changed:', chainId);
});
web3 is a comprehensive library for interacting with the Ethereum blockchain. It provides functionalities for sending transactions, interacting with smart contracts, and more. Compared to @metamask/sdk, web3 offers a broader range of blockchain interactions but requires more setup for MetaMask-specific functionalities.
ethers is a lightweight library for interacting with the Ethereum blockchain. It provides utilities for managing keys, interacting with smart contracts, and sending transactions. Similar to web3, ethers is more general-purpose and requires additional setup for MetaMask-specific interactions.
web3modal is a library that provides a simple way to connect to various wallet providers, including MetaMask. It offers a user-friendly modal for selecting and connecting to wallets. Compared to @metamask/sdk, web3modal focuses on providing a seamless wallet connection experience across multiple providers.
The MetaMask SDK enables developers to easily connect their dapps with a MetaMask wallet (Extension or Mobile) no matter the dapp environment or platform.
The MetaMask SDK is a library that can be installed by developers on their projects and will automatically guide their users to easily connect with a MetaMask wallet client. For instance, for dapps running on a desktop browser, the SDK will check if Extension is installed and if not it will prompt the user to install it or to connect via QR code with their MetaMask Mobile wallet. Another example, for native mobile applications, the SDK will automatically deeplink into MetaMask Mobile wallet to make the connection.
The MetaMask SDK instance returns a provider, this provider is the ethereum
object that developers are already used to which is here. This provider will now be available for:
The following code examplifies importing the SDK into a javascript-based app. For other languages, check the sections bellow.
Install the SDK:
yarn add @metamask/sdk
or
npm i @metamask/sdk
Import the SDK (for possible parameters check this):
import MetaMaskSDK from '@metamask/sdk'
const ethereum = new MetaMaskSDK({})
Use the SDK:
ethereum.request({method: 'eth_requestAccounts', params: []})
Please access this to read the MetaMask SDK full documentation
Contact the MetaMask SDK team for a complimentary design optimization workshop here
FAQs
Unknown package
The npm package @metamask/sdk receives a total of 154,152 weekly downloads. As such, @metamask/sdk popularity was classified as popular.
We found that @metamask/sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 11 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.