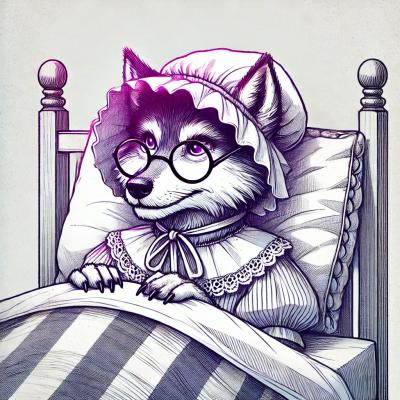
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@metaplex-foundation/beet
Advanced tools
Strict borsh compatible de/serializer.
Table of Contents generated with DocToc
coption(array(utf8String))
is handled
correctlybyteSize
of any fixed size type, no matter how deeply nested or composed it isbeet:debug struct GameStruct {
beet:debug win: u8 1 B
beet:debug totalWin: u16 2 B
beet:debug whaleAccount: u128 16 B
beet:debug losses: i32 4 B
beet:debug } 23 B +0ms
beet:trace serializing [GameStruct] _GameScore { win: 1, totalWin: 100, whaleAccount: <BN: fffffffffffffffffffffffffffffffb>, losses: -234 } to 23 bytes buffer +0ms
beet:trace deserializing [GameStruct] from 23 bytes buffer +2ms
beet:trace <Buffer 01 64 00 fb ff ff ff ff ff ff ff ff ff ff ff ff ff ff ff 16 ff ff ff> +0ms
beet:trace [
beet:trace 1, 100, 0, 251, 255, 255,
beet:trace 255, 255, 255, 255, 255, 255,
beet:trace 255, 255, 255, 255, 255, 255,
beet:trace 255, 22, 255, 255, 255
beet:trace ] +0ms
Beet is optimized for fixed types as this allows logging detailed diagnostics about the structure of data that it is processing as well as avoiding Buffer resizes.
Only beets that have fixed in the name are of fixed size, all others are fixable types
which expose toFixedFromData
and toFixedFromValue
methods to convert to a fixed beet from
serialized data or a value respectively.
Beet provides the FixableBeetStruct
to de/serialize args that have non-fixed size fields.
Thus beet implements the entire borsh spec, however if you want a library that processes dynamic types directly use one of the alternatives, i.e. borsh-js.
Please find the API docs here.
import { BeetStruct, i32, u16, u8 } from '@metaplex-foundation/beet'
class Result {
constructor(
readonly win: number,
readonly totalWin: number,
readonly losses: number
) {}
static readonly struct = new BeetStruct<Result>(
[
['win', u8],
['totalWin', u16],
['losses', i32],
],
(args) => new Result(args.win!, args.totalWin!, args.losses!),
'Results'
)
}
import { FixableBeetStruct, i32, u16, u8, array } from '@metaplex-foundation/beet'
class Result {
constructor(
readonly win: number,
readonly totalWin: number,
readonly losses: number[]
) {}
static readonly struct = new FixableBeetStruct<Result>(
[
['win', u8],
['totalWin', u16],
['losses', array(i32)],
],
(args) => new Result(args.win!, args.totalWin!, args.losses!),
'Results'
)
}
NOTE: uses Result
struct from the above example for the results
field of Trader
import { BeetStruct, fixedSizeUtf8String } from '@metaplex-foundation/beet'
class Trader {
constructor(
readonly name: string,
readonly results: Results,
readonly age: number
) {}
static readonly struct = new BeetStruct<Trader>(
[
['name', fixedSizeUtf8String(4)],
['results', Results.struct],
['age', u8],
],
(args) => new Trader(args.name!, args.results!, args.age!),
'Trader'
)
}
const trader = new Trader('bob ', new Results(20, 1200, -455), 22)
const [buf] = Trader.struct.serialize(trader)
const [deserializedTrader] = Trader.struct.deserialize(buf)
NOTE: depends on beet-solana
extension package for the PublicKey
implementation
import * as web3 from '@solana/web3.js'
import * as beet from '@metaplex-foundation/beet'
import * as beetSolana from '@metaplex-foundation/beet-solana'
type InstructionArgs = {
instructionDiscriminator: number[]
authority: web3.PublicKey
maybePublickKey: beet.COption<web3.PublicKey>
}
// Uses the BeetArgsStruct wrapper around BeetStruct
const createStruct = new beet.BeetArgsStruct<InstructionArgs>(
[
['instructionDiscriminator', beet.fixedSizeArray(beet.u8, 8)],
['authority', beetSolana.publicKey],
['maybePublickKey', beet.coption(beetSolana.publicKey)],
],
'InstructionArgs'
)
import { u8 } from '@metaplex-foundation/beet'
const n = 1
const buf = Buffer.alloc(u8.byteSize)
u8.write(buf, 0, n)
u8.read(buf, 0) // === 1
import { u8, array } from '@metaplex-foundation/beet'
const xs = [ 1, 2 ]
const beet = array(u8)
const fixedBeet = beet.toFixedFromValue(xs)
const buf = Buffer.alloc(fixedBeet.byteSize)
fixedBeet.write(buf, 0, xs)
fixedBeet.read(buf, 0) // === [ 1, 2 ]
NOTE: use Result
struct from the above example to wrap in a Composite type
NOTE: that the coption
is a fixable beet since it has a different size for the Some vs.
the None case.
const resultOption: Beet<COption<Result>> = coption(Result.struct)
const result = new Result(20, 1200, -455)
const fixedBeet = resultOption.toFixedFromValue(result)
const buf = Buffer.alloc(fixedBeet.byteSize)
fixedBeet.write(buf, 0, result)
beet.read(buf, 0) // same as result
const resultArray: Beet<Array<Result>> = uniformFixedSizeArray(Result.struct, 3)
const results =[ new Result(20, 1200, -455), new Result(3, 999, 0), new Result(30, 100, -3) ]
const buf = Buffer.alloc(resultArray.byteSize)
beet.write(buf, 0, results)
beet.read(buf, 0) // same as results
const resultArray: Beet<Array<Result>> = array(Result.struct)
const results =[ new Result(20, 1200, -455), new Result(3, 999, 0), new Result(30, 100, -3) ]
const fixedBeet = resultsArray.toFixedFromValue(results)
const buf = Buffer.alloc(fixedBeet.byteSize)
fixedBeet.write(buf, 0, results)
fixedBeet.read(buf, 0) // same as results
NOTE: this sample is what solita will generate from a provided IDL. solita is the recommended way to create TypeScript that uses beet for de/serialization.
// -----------------
// Setup
// -----------------
type CollectionInfoRecord = {
V1: {
symbol: string
verifiedCreators: web3.PublicKey[]
whitelistRoot: number[] /* size: 32 */
}
V2: { collectionMint: web3.PublicKey }
}
type CollectionInfo = beet.DataEnumKeyAsKind<CollectionInfoRecord>
const collectionInfoBeet = beet.dataEnum<CollectionInfoRecord>([
[
'V1',
new beet.FixableBeetArgsStruct<CollectionInfoRecord['V1']>(
[
['symbol', beet.utf8String],
['verifiedCreators', beet.array(beetSolana.publicKey)],
['whitelistRoot', beet.uniformFixedSizeArray(beet.u8, 32)],
],
'CollectionInfoRecord["V1"]'
),
],
[
'V2',
new beet.BeetArgsStruct<CollectionInfoRecord['V2']>(
[['collectionMint', beetSolana.publicKey]],
'CollectionInfoRecord["V2"]'
),
],
]) as beet.FixableBeet<CollectionInfo>
// -----------------
// Usage
// -----------------
const collectionV1: CollectionInfo & { __kind: 'V1' } = {
__kind: 'V1',
symbol: 'SYM',
verifiedCreators: [new web3.PublicKey(1), new web3.PublicKey(2)],
whitelistRoot: new Array(32).fill(33),
}
const fixedBeet = collectionInfoBeet.toFixedFromValue(collectionV1)
// Serialize
const buf = Buffer.alloc(fixedBeet.byteSize)
fixedBeet.write(buf, 0, collectionV1)
// Deserialize
const val = fixedBeet.read(buf, 0)
console.log(val)
Apache-2.0
FAQs
Strict borsh compatible de/serializer
We found that @metaplex-foundation/beet demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.