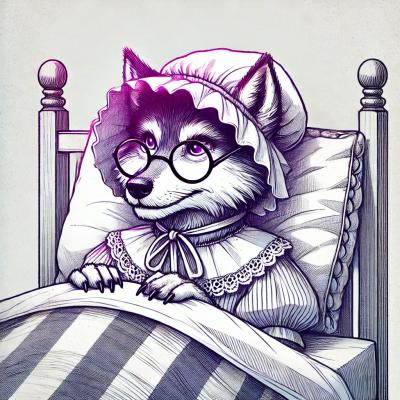
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@metaplex-foundation/beet-solana
Advanced tools
Solana specific extension for beet, the borsh compatible de/serializer
Solana specific extension for beet, the borsh compatible de/serializer
Please find the API docs here.
solana-beet uses beet
s knowledge about account layouts to provide GpaBuilder
s for
them which allow to filter by account data size and content.
const gpaBuilder = GpaBuilder.fromStruct(programId, accountStruct)
gpaBuilder.dataSize
, gpaBuilder.addFilter
or gpaBuilder.addInnerFilter
gpaBuilder.run(connection)
which will return all accounts matching the specified
filtersexport type ResultsArgs = Pick<Results, 'win' | 'totalWin' | 'losses'>
export class Results {
constructor(
readonly win: number,
readonly totalWin: number,
readonly losses: number
) {}
static readonly struct = new BeetStruct<Results, ResultsArgs>(
[
['win', u8],
['totalWin', u16],
['losses', i32],
],
(args: ResultsArgs) => new Results(args.win!, args.totalWin!, args.losses!),
'Results'
)
}
const gpaBuilder = GpaBuilder.fromStruct(PROGRAM_ID, Results.struct)
const accounts = await gpaBuilder
.addFilter('totalWin', 8)
.addFilter('losses', -7)
.run()
Using Results
struct from above
export type TraderArgs = Pick<Trader, 'name' | 'results' | 'age'>
export class Trader {
constructor(
readonly name: string,
readonly results: Results,
readonly age: number
) {}
static readonly struct = new BeetStruct<Trader, TraderArgs>(
[
['name', fixedSizeUtf8String(4)],
['results', Results.struct],
['age', u8],
],
(args) => new Trader(args.name!, args.results!, args.age!),
'Trader'
)
}
const gpaBuilder = GpaBuilder.fromStruct<Trader>(
PROGRAM_ID,
Trader.struct
)
const results = {
win: 3,
totalWin: 4,
losses: -100,
}
const accounts = await gpaBuilder.addFilter('results', results).run()
Using Trader
struct from above
const gpaBuilder = GpaBuilder.fromStruct<Trader>(
PROGRAM_ID,
Trader.struct
)
const account = await gpaBuilder
.addInnerFilter('results.totalWin', 8)
.addInnerFilter('results.win', 2)
.run()
solana-beet provides a de/serializer for solana public keys. They can either be used directly or as part of a struct.
import { publicKey } from '@metaplex-foundation/beet-solana'
const generatedKey = Keypair.generate().publicKey
const buf = Buffer.alloc(publicKey.byteSize)
beet.write(buf, 0, generatedKey)
beet.read(buf, 0) // same as generatedKey
import * as web3 from '@solana/web3.js'
import * as beet from '@metaplex-foundation/beet'
import * as beetSolana from '@metaplex-foundation/beet-solana'
type InstructionArgs = {
authority: web3.PublicKey
}
const createStruct = new beet.BeetArgsStruct<InstructionArgs>(
[
['authority', beetSolana.publicKey]
],
'InstructionArgs'
)
Apache-2.0
FAQs
Solana specific extension for beet, the borsh compatible de/serializer
The npm package @metaplex-foundation/beet-solana receives a total of 45,806 weekly downloads. As such, @metaplex-foundation/beet-solana popularity was classified as popular.
We found that @metaplex-foundation/beet-solana demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.