@microsoft/microsoft-graph-client
Advanced tools
Comparing version 1.0.0 to 1.1.0
"use strict"; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
require("isomorphic-fetch"); | ||
var ResponseHandler_1 = require("../../src/ResponseHandler"); | ||
var assert = require("assert"); | ||
var _200_SUPERAGENT_RES = { | ||
ok: true, | ||
statusCode: 200, | ||
body: { | ||
a: 1 | ||
var _200_RES_BODY = { a: 1 }; | ||
var _200_RES_INIT = { status: 200 }; | ||
var _200_RES = new Response(_200_RES_BODY, _200_RES_INIT); | ||
var _500_RES_BODY = { | ||
error: { | ||
"code": "SearchEvents", | ||
"message": "The parameter $search is not currently supported on the Events resource.", | ||
"innerError": { | ||
"request-id": "b31c83fd-944c-4663-aa50-5d9ceb367e19", | ||
"date": "2016-11-17T18:37:45" | ||
} | ||
} | ||
}; | ||
var _500_SUPERAGENT_RES = { | ||
ok: false, | ||
response: { | ||
body: { | ||
error: { | ||
"code": "SearchEvents", | ||
"message": "The parameter $search is not currently supported on the Events resource.", | ||
"innerError": { | ||
"request-id": "b31c83fd-944c-4663-aa50-5d9ceb367e19", | ||
"date": "2016-11-17T18:37:45" | ||
} | ||
} | ||
} | ||
}, | ||
statusCode: 500 | ||
}; | ||
var _500_RES_INIT = { status: 500 }; | ||
var _500_RES = new Response(_500_RES_BODY, _500_RES_INIT); | ||
describe('#handleResponse()', function () { | ||
it('passes through response to callback', function () { | ||
ResponseHandler_1.ResponseHandler.init(null, _200_SUPERAGENT_RES, function (err, res) { | ||
assert.equal(res, _200_SUPERAGENT_RES.body); | ||
ResponseHandler_1.ResponseHandler.init(_200_RES, null, _200_RES_BODY, function (err, res) { | ||
assert.equal(res, _200_RES.body); | ||
}); | ||
}); | ||
it('200 response => err is null', function () { | ||
ResponseHandler_1.ResponseHandler.init(null, _200_SUPERAGENT_RES, function (err, res) { | ||
ResponseHandler_1.ResponseHandler.init(_200_RES, null, _200_RES_BODY, function (err, res) { | ||
assert.equal(err, null); | ||
@@ -40,20 +33,18 @@ }); | ||
}); | ||
describe('#ParseResponse()', function () { | ||
it('extracts code and request-id from the JSON body of 500 errors', function () { | ||
ResponseHandler_1.ResponseHandler.init(_500_RES, null, _500_RES_BODY, function (err, res) { | ||
assert.equal(err.code, _500_RES_BODY.error.code); | ||
assert.equal(err.requestId, _500_RES_BODY.error.innerError["request-id"]); | ||
}); | ||
}); | ||
}); | ||
describe('#ParseError()', function () { | ||
it('500 error => res param in callback is null', function () { | ||
ResponseHandler_1.ResponseHandler.init(null, _500_SUPERAGENT_RES, function (err, res) { | ||
it('res param in callback is null', function () { | ||
ResponseHandler_1.ResponseHandler.init(null, null, null, function (err, res) { | ||
assert.equal(res, null); | ||
assert.equal(err.statusCode, -1); | ||
}); | ||
}); | ||
it('extracts code and request-id from the JSON body of 500 errors', function () { | ||
ResponseHandler_1.ResponseHandler.init(null, _500_SUPERAGENT_RES, function (err, res) { | ||
assert.equal(err.code, _500_SUPERAGENT_RES.response.body.error.code); | ||
assert.equal(err.requestId, _500_SUPERAGENT_RES.response.body.error.innerError["request-id"]); | ||
}); | ||
}); | ||
it('parses a 404 superagent error', function () { | ||
var rawErr = JSON.parse('{"original":null,"response":{"req":{"method":"GET","url":"https://graph.microsoft.com/v1.0/users/4470c514-8ac5-4f2d-8116-870d2c41bdf6/photo/$value","headers":{"authorization":"Bearer abc","cache-control":"no-cache","sdkversion":"graph-js-0.2.0"}},"xhr":{},"text":null,"statusText":"Not Found","statusCode":404,"status":404,"statusType":4,"info":false,"ok":false,"clientError":true,"serverError":false,"error":{"status":404,"method":"GET","url":"https://graph.microsoft.com/v1.0/users/4470c514-8ac5-4f2d-8116-870d2c41bdf6/photo/$value"},"accepted":false,"noContent":false,"badRequest":false,"unauthorized":false,"notAcceptable":false,"notFound":true,"forbidden":false,"headers":{"client-request-id":"3726fdf7-8ae6-47c0-9f6a-5847982738d2","content-type":"text/plain","cache-control":"private","request-id":"3726fdf7-8ae6-47c0-9f6a-5847982738d2"},"header":{"client-request-id":"3726fdf7-8ae6-47c0-9f6a-5847982738d2","content-type":"text/plain","cache-control":"private","request-id":"3726fdf7-8ae6-47c0-9f6a-5847982738d2"},"type":"text/plain","body":null},"status":404}'); | ||
var graphErr = ResponseHandler_1.ResponseHandler.ParseError(rawErr); | ||
assert.equal(graphErr.statusCode, 404); | ||
}); | ||
}); | ||
//# sourceMappingURL=responseHandling.js.map |
export declare let oDataQueryNames: string[]; | ||
export declare const DEFAULT_VERSION = "v1.0"; | ||
export declare const GRAPH_BASE_URL = "https://graph.microsoft.com/"; | ||
export declare const PACKAGE_VERSION = "1.0.0"; | ||
export interface AuthProviderCallback { | ||
@@ -24,2 +25,6 @@ (error: any, accessToken: string): void; | ||
} | ||
export interface DefaultRequestHeaders { | ||
Authorization: string; | ||
SdkVersion: string; | ||
} | ||
export interface GraphRequestCallback { | ||
@@ -26,0 +31,0 @@ (error: GraphError, response: any, rawResponse?: any): void; |
@@ -6,3 +6,4 @@ "use strict"; | ||
exports.GRAPH_BASE_URL = "https://graph.microsoft.com/"; | ||
exports.PACKAGE_VERSION = "1.0.0"; | ||
exports.oDataQueryNames = exports.oDataQueryNames.concat(exports.oDataQueryNames.map(function (s) { return "$" + s; })); | ||
//# sourceMappingURL=common.js.map |
import { Promise } from 'es6-promise'; | ||
import 'isomorphic-fetch'; | ||
import { Options, URLComponents, GraphRequestCallback } from "./common"; | ||
@@ -16,8 +17,8 @@ export declare class GraphRequest { | ||
parsePath(rawPath: string): void; | ||
private urlJoin(urlSegments); | ||
private urlJoin; | ||
buildFullUrl(): string; | ||
version(v: string): GraphRequest; | ||
select(properties: string | [string]): GraphRequest; | ||
expand(properties: string | [string]): GraphRequest; | ||
orderby(properties: string | [string]): GraphRequest; | ||
select(properties: string | string[]): GraphRequest; | ||
expand(properties: string | string[]): GraphRequest; | ||
orderby(properties: string | string[]): GraphRequest; | ||
filter(filterStr: string): GraphRequest; | ||
@@ -29,3 +30,3 @@ top(n: number): GraphRequest; | ||
responseType(responseType: string): GraphRequest; | ||
private addCsvQueryParamater(propertyName, propertyValue, additionalProperties); | ||
private addCsvQueryParamater; | ||
delete(callback?: GraphRequestCallback): Promise<any>; | ||
@@ -39,12 +40,15 @@ patch(content: any, callback?: GraphRequestCallback): Promise<any>; | ||
get(callback?: GraphRequestCallback): Promise<any>; | ||
private routeResponseToPromise(requestBuilder); | ||
private routeResponseToCallback(requestBuilder, callback); | ||
private sendRequestAndRouteResponse(requestBuilder, callback?); | ||
private routeResponseToPromise; | ||
private handleFetch; | ||
private routeResponseToCallback; | ||
private sendRequestAndRouteResponse; | ||
getStream(callback: GraphRequestCallback): void; | ||
putStream(stream: any, callback: Function): void; | ||
private configureRequest(requestBuilder, accessToken); | ||
putStream(stream: any, callback: GraphRequestCallback): void; | ||
private getDefaultRequestHeaders; | ||
private configureRequest; | ||
query(queryDictionaryOrString: string | { | ||
[key: string]: string | number; | ||
}): GraphRequest; | ||
private createQueryString(); | ||
private createQueryString; | ||
private convertResponseType; | ||
} |
"use strict"; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
var request = require("superagent"); | ||
var es6_promise_1 = require("es6-promise"); | ||
require("isomorphic-fetch"); | ||
var common_1 = require("./common"); | ||
var ResponseHandler_1 = require("./ResponseHandler"); | ||
var packageInfo = require('../../package.json'); | ||
var RequestMethod_1 = require("./RequestMethod"); | ||
var GraphHelper_1 = require("./GraphHelper"); | ||
var GraphRequest = (function () { | ||
@@ -137,22 +138,27 @@ function GraphRequest(config, path) { | ||
var url = this.buildFullUrl(); | ||
return this.sendRequestAndRouteResponse(request.del(url), callback); | ||
return this.sendRequestAndRouteResponse(new Request(url, { method: RequestMethod_1.RequestMethod.DELETE, headers: new Headers() }), callback); | ||
}; | ||
GraphRequest.prototype.patch = function (content, callback) { | ||
var url = this.buildFullUrl(); | ||
return this.sendRequestAndRouteResponse(request | ||
.patch(url) | ||
.send(content), callback); | ||
return this.sendRequestAndRouteResponse(new Request(url, { | ||
method: RequestMethod_1.RequestMethod.PATCH, | ||
body: GraphHelper_1.GraphHelper.serializeContent(content), | ||
headers: new Headers({ 'Content-Type': 'application/json' }) | ||
}), callback); | ||
}; | ||
GraphRequest.prototype.post = function (content, callback) { | ||
var url = this.buildFullUrl(); | ||
return this.sendRequestAndRouteResponse(request | ||
.post(url) | ||
.send(content), callback); | ||
return this.sendRequestAndRouteResponse(new Request(url, { | ||
method: RequestMethod_1.RequestMethod.POST, | ||
body: GraphHelper_1.GraphHelper.serializeContent(content), | ||
headers: new Headers((content.constructor !== undefined && content.constructor.name === "FormData") ? {} : { 'Content-Type': 'application/json' }) | ||
}), callback); | ||
}; | ||
GraphRequest.prototype.put = function (content, callback) { | ||
var url = this.buildFullUrl(); | ||
return this.sendRequestAndRouteResponse(request | ||
.put(url) | ||
.type('application/octet-stream') | ||
.send(content), callback); | ||
return this.sendRequestAndRouteResponse(new Request(url, { | ||
method: RequestMethod_1.RequestMethod.PUT, | ||
body: GraphHelper_1.GraphHelper.serializeContent(content), | ||
headers: new Headers({ 'Content-Type': 'application/octet-stream' }) | ||
}), callback); | ||
}; | ||
@@ -170,9 +176,8 @@ GraphRequest.prototype.create = function (content, callback) { | ||
var url = this.buildFullUrl(); | ||
return this.sendRequestAndRouteResponse(request | ||
.get(url), callback); | ||
return this.sendRequestAndRouteResponse(new Request(url, { method: RequestMethod_1.RequestMethod.GET, headers: new Headers() }), callback); | ||
}; | ||
GraphRequest.prototype.routeResponseToPromise = function (requestBuilder) { | ||
GraphRequest.prototype.routeResponseToPromise = function (request) { | ||
var _this = this; | ||
return new es6_promise_1.Promise(function (resolve, reject) { | ||
_this.routeResponseToCallback(requestBuilder, function (err, body) { | ||
_this.routeResponseToCallback(request, function (err, body) { | ||
if (err != null) { | ||
@@ -187,10 +192,20 @@ reject(err); | ||
}; | ||
GraphRequest.prototype.routeResponseToCallback = function (requestBuilder, callback) { | ||
GraphRequest.prototype.handleFetch = function (request, callback, options) { | ||
var _this = this; | ||
this.config.authProvider(function (err, accessToken) { | ||
((request.constructor.name === "Request") ? fetch(request) : fetch(request, options)).then(function (response) { | ||
_this.convertResponseType(response).then(function (responseValue) { | ||
ResponseHandler_1.ResponseHandler.init(response, undefined, responseValue, callback); | ||
}).catch(function (error) { | ||
ResponseHandler_1.ResponseHandler.init(response, error, undefined, callback); | ||
}); | ||
}).catch(function (error) { | ||
ResponseHandler_1.ResponseHandler.init(undefined, error, undefined, callback); | ||
}); | ||
}; | ||
GraphRequest.prototype.routeResponseToCallback = function (request, callback) { | ||
var self = this; | ||
self.config.authProvider(function (err, accessToken) { | ||
if (err == null && accessToken != null) { | ||
var request_1 = _this.configureRequest(requestBuilder, accessToken); | ||
request_1.end(function (err, res) { | ||
ResponseHandler_1.ResponseHandler.init(err, res, callback); | ||
}); | ||
request = self.configureRequest(request, accessToken); | ||
self.handleFetch(request, callback); | ||
} | ||
@@ -202,16 +217,22 @@ else { | ||
}; | ||
GraphRequest.prototype.sendRequestAndRouteResponse = function (requestBuilder, callback) { | ||
GraphRequest.prototype.sendRequestAndRouteResponse = function (request, callback) { | ||
if (callback == null && typeof es6_promise_1.Promise !== "undefined") { | ||
return this.routeResponseToPromise(requestBuilder); | ||
return this.routeResponseToPromise(request); | ||
} | ||
else { | ||
this.routeResponseToCallback(requestBuilder, callback || function () { }); | ||
this.routeResponseToCallback(request, callback || function () { }); | ||
} | ||
}; | ||
GraphRequest.prototype.getStream = function (callback) { | ||
var _this = this; | ||
this.config.authProvider(function (err, accessToken) { | ||
var self = this; | ||
self.config.authProvider(function (err, accessToken) { | ||
if (err === null && accessToken !== null) { | ||
var url = _this.buildFullUrl(); | ||
callback(null, _this.configureRequest(request.get(url), accessToken)); | ||
var url = self.buildFullUrl(); | ||
var options_1 = { | ||
method: RequestMethod_1.RequestMethod.GET, | ||
headers: self.getDefaultRequestHeaders(accessToken) | ||
}; | ||
self.responseType("stream"); | ||
Object.keys(self._headers).forEach(function (key) { return options_1.headers[key] = self._headers[key]; }); | ||
self.handleFetch(url, callback, options_1); | ||
} | ||
@@ -224,27 +245,30 @@ else { | ||
GraphRequest.prototype.putStream = function (stream, callback) { | ||
var _this = this; | ||
this.config.authProvider(function (err, accessToken) { | ||
var self = this; | ||
self.config.authProvider(function (err, accessToken) { | ||
if (err === null && accessToken !== null) { | ||
var url = _this.buildFullUrl(); | ||
var req = _this.configureRequest(request.put(url), accessToken); | ||
req.type('application/octet-stream'); | ||
stream | ||
.pipe(req) | ||
.on('error', function (err) { | ||
callback(err, null); | ||
}) | ||
.on('end', function () { | ||
callback(null); | ||
}); | ||
var url = self.buildFullUrl(); | ||
var options_2 = { | ||
method: RequestMethod_1.RequestMethod.PUT, | ||
headers: { | ||
'Content-Type': 'application/octet-stream', | ||
}, | ||
body: stream | ||
}; | ||
var defaultHeaders_1 = self.getDefaultRequestHeaders(accessToken); | ||
Object.keys(defaultHeaders_1).forEach(function (key) { return options_2.headers[key] = defaultHeaders_1[key]; }); | ||
Object.keys(self._headers).forEach(function (key) { return options_2.headers[key] = self._headers[key]; }); | ||
self.handleFetch(url, callback, options_2); | ||
} | ||
}); | ||
}; | ||
GraphRequest.prototype.configureRequest = function (requestBuilder, accessToken) { | ||
var request = requestBuilder | ||
.set('Authorization', 'Bearer ' + accessToken) | ||
.set(this._headers) | ||
.set('SdkVersion', "graph-js-" + packageInfo.version); | ||
if (this._responseType !== undefined) { | ||
request.responseType(this._responseType); | ||
} | ||
GraphRequest.prototype.getDefaultRequestHeaders = function (accessToken) { | ||
return { | ||
Authorization: "Bearer " + accessToken, | ||
SdkVersion: "graph-js-" + common_1.PACKAGE_VERSION | ||
}; | ||
}; | ||
GraphRequest.prototype.configureRequest = function (request, accessToken) { | ||
var self = this, defaultHeaders = self.getDefaultRequestHeaders(accessToken); | ||
Object.keys(defaultHeaders).forEach(function (key) { return request.headers.set(key, defaultHeaders[key]); }); | ||
Object.keys(self._headers).forEach(function (key) { return request.headers.set(key, self._headers[key]); }); | ||
return request; | ||
@@ -283,2 +307,32 @@ }; | ||
}; | ||
GraphRequest.prototype.convertResponseType = function (response) { | ||
var responseValue; | ||
if (!this._responseType) { | ||
this._responseType = ''; | ||
} | ||
switch (this._responseType.toLowerCase()) { | ||
case "arraybuffer": | ||
responseValue = response.arrayBuffer(); | ||
break; | ||
case "blob": | ||
responseValue = response.blob(); | ||
break; | ||
case "document": | ||
responseValue = response.json(); | ||
break; | ||
case "json": | ||
responseValue = response.json(); | ||
break; | ||
case "text": | ||
responseValue = response.text(); | ||
break; | ||
case "stream": | ||
responseValue = es6_promise_1.Promise.resolve(response.body); | ||
break; | ||
default: | ||
responseValue = response.json(); | ||
break; | ||
} | ||
return responseValue; | ||
}; | ||
return GraphRequest; | ||
@@ -285,0 +339,0 @@ }()); |
@@ -8,1 +8,4 @@ import { Options } from "./common"; | ||
} | ||
export * from "./GraphRequest"; | ||
export * from "./common"; | ||
export * from "./ResponseHandler"; |
"use strict"; | ||
function __export(m) { | ||
for (var p in m) if (!exports.hasOwnProperty(p)) exports[p] = m[p]; | ||
} | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
@@ -26,2 +29,5 @@ var common_1 = require("./common"); | ||
exports.Client = Client; | ||
__export(require("./GraphRequest")); | ||
__export(require("./common")); | ||
__export(require("./ResponseHandler")); | ||
//# sourceMappingURL=index.js.map |
import { GraphRequestCallback, GraphError } from "./common"; | ||
export declare class ResponseHandler { | ||
static init(err: any, res: any, callback: GraphRequestCallback): void; | ||
static ParseError(rawErr: any): GraphError; | ||
static init(res: any, err: any, resContents: any, callback: GraphRequestCallback): void; | ||
static ParseError(rawErr: Error): GraphError; | ||
static defaultGraphError(statusCode: number): GraphError; | ||
static buildGraphErrorFromErrorObject(errObj: Error): GraphError; | ||
static buildGraphErrorFromResponseObject(errObj: any, statusCode: number): GraphError; | ||
} |
@@ -6,9 +6,12 @@ "use strict"; | ||
} | ||
ResponseHandler.init = function (err, res, callback) { | ||
ResponseHandler.init = function (res, err, resContents, callback) { | ||
if (res && res.ok) { | ||
callback(null, res.body, res); | ||
callback(null, resContents, res); | ||
} | ||
else { | ||
if (err == null && res.error !== null) | ||
callback(ResponseHandler.ParseError(res), null, res); | ||
if (err == null && res != null) | ||
if (resContents != null && resContents.error != null) | ||
callback(ResponseHandler.buildGraphErrorFromResponseObject(resContents.error, res.status), null, res); | ||
else | ||
callback(ResponseHandler.defaultGraphError(res.status), null, res); | ||
else | ||
@@ -19,30 +22,27 @@ callback(ResponseHandler.ParseError(err), null, res); | ||
ResponseHandler.ParseError = function (rawErr) { | ||
var errObj; | ||
if (!('rawResponse' in rawErr)) { | ||
if (rawErr.response !== undefined && rawErr.response.body !== null && 'error' in rawErr.response.body) { | ||
errObj = rawErr.response.body.error; | ||
} | ||
if (!rawErr) { | ||
return ResponseHandler.defaultGraphError(-1); | ||
} | ||
else { | ||
errObj = JSON.parse(rawErr.rawResponse.replace(/^\uFEFF/, '')).error; | ||
} | ||
var statusCode; | ||
if (rawErr.response !== undefined && rawErr.response.status !== undefined) { | ||
statusCode = rawErr.response.status; | ||
} | ||
else { | ||
statusCode = rawErr.statusCode; | ||
} | ||
if (errObj === undefined) { | ||
return { | ||
statusCode: statusCode, | ||
code: null, | ||
message: null, | ||
requestId: null, | ||
date: new Date(), | ||
body: null | ||
}; | ||
} | ||
var err = { | ||
return ResponseHandler.buildGraphErrorFromErrorObject(rawErr); | ||
}; | ||
ResponseHandler.defaultGraphError = function (statusCode) { | ||
return { | ||
statusCode: statusCode, | ||
code: null, | ||
message: null, | ||
requestId: null, | ||
date: new Date(), | ||
body: null | ||
}; | ||
}; | ||
ResponseHandler.buildGraphErrorFromErrorObject = function (errObj) { | ||
var error = ResponseHandler.defaultGraphError(-1); | ||
error.body = errObj.toString(); | ||
error.message = errObj.message; | ||
error.date = new Date(); | ||
return error; | ||
}; | ||
ResponseHandler.buildGraphErrorFromResponseObject = function (errObj, statusCode) { | ||
return { | ||
statusCode: statusCode, | ||
code: errObj.code, | ||
@@ -54,3 +54,2 @@ message: errObj.message, | ||
}; | ||
return err; | ||
}; | ||
@@ -57,0 +56,0 @@ return ResponseHandler; |
{ | ||
"name": "@microsoft/microsoft-graph-client", | ||
"version": "1.0.0", | ||
"//": "NOTE: The version here should match exactly the exported const PACKAGE_VERSION in common.ts. If you change it here, also change it there.", | ||
"version": "1.1.0", | ||
"description": "Microsoft Graph Client Library", | ||
@@ -12,10 +13,11 @@ "main": "lib/src/index.js", | ||
"devDependencies": { | ||
"@types/superagent": "^2.0.36", | ||
"@types/mocha": "^2.2.34", | ||
"@types/mocha": "^5.2.4", | ||
"@types/node": "^9.4.0", | ||
"browserify": "^13.1.0", | ||
"mocha": "^3.2.0", | ||
"typescript": "^2.2.1" | ||
"mocha": "^5.2.0", | ||
"typescript": "^2.2.1", | ||
"uglify-js": "^3.4.5" | ||
}, | ||
"scripts": { | ||
"build": "tsc && node node-browserify.js > lib/graph-js-sdk-web.js", | ||
"build": "tsc && node node-browserify.js > lib/graph-js-sdk-web.js && uglifyjs ./lib/graph-js-sdk-web.js --output ./lib/graph-js-sdk-web.js", | ||
"test": "mocha lib/spec/core", | ||
@@ -25,4 +27,4 @@ "test:types": "tsc --p spec/types && mocha spec/types" | ||
"dependencies": { | ||
"superagent": "^3.5.2", | ||
"es6-promise": "^4.1.0" | ||
"es6-promise": "^4.1.0", | ||
"isomorphic-fetch": "^2.2.1" | ||
}, | ||
@@ -33,2 +35,2 @@ "repository": { | ||
} | ||
} | ||
} |
@@ -5,11 +5,15 @@ # Microsoft Graph JavaScript Client Library | ||
The Microsoft Graph JavaScript client library is a lightweight wrapper around the Microsoft Graph API that supports both Node and the browser. See the [samples](samples) folder for code examples. You can also use our [TypeScript graph types](https://github.com/microsoftgraph/msgraph-typescript-typings) with this library. We love your feedback! You can file an issue in this repository or write on our [UserVoice page](https://officespdev.uservoice.com/). | ||
The Microsoft Graph JavaScript client library is a lightweight wrapper around the Microsoft Graph API that can be used server-side and in the browser. See the [samples](samples) folder for code examples. You can also use our [TypeScript graph types](https://github.com/microsoftgraph/msgraph-typescript-typings) with this library. We love your feedback! You can file an issue in this repository or write on our [UserVoice page](https://officespdev.uservoice.com/). | ||
**Looking for IntelliSense on models (Users, Groups, etc.)? Check out the [Microsoft Graph Types](https://github.com/microsoftgraph/msgraph-typescript-typings) repository!** | ||
[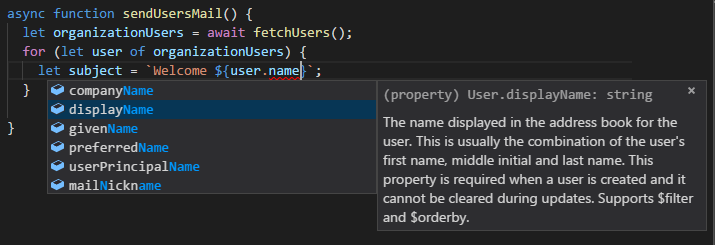](https://github.com/microsoftgraph/msgraph-typescript-typings) | ||
## Installation | ||
### Node | ||
You can find installation instructions at the [Node.js website](https://nodejs.org/). | ||
1) Install [Node.js and npm](https://nodejs.org/). | ||
```npm install @microsoft/microsoft-graph-client``` | ||
2) ```npm install @microsoft/microsoft-graph-client``` | ||
Include the library in your JavaScript file with `const MicrosoftGraph = require("@microsoft/microsoft-graph-client");` | ||
3) Include the library in your JavaScript file with `const MicrosoftGraph = require("@microsoft/microsoft-graph-client");` | ||
@@ -22,26 +26,3 @@ ### Browser | ||
``` | ||
## Changelog | ||
#### 1.0.0 | ||
* Added tests for new Graph functionality - Delta query, Extensibility, OneNote, and more. | ||
#### 0.4.0 | ||
* Add support for ES5. Make sure to use `graph-js-sdk-web.js` for web apps | ||
* Removed iterator helper method. | ||
#### 0.3.1 | ||
* Support for Node.js versions 4 and 5 | ||
#### 0.3.0 | ||
* Migrated away from typings in client library core and TypeScript sample | ||
#### 0.2.2 | ||
* Updated SuperAgent to version ``` 3.3.0 ``` | ||
#### 0.2.0 | ||
* **Breaking change for existing apps** - Initialize the client library with `MicrosoftGraph.Client.init({...})`. See the updated usage section below for code samples. | ||
* Added response handling tests to simulate Graph calls | ||
* Added type declarations file for core client library, which adds intellisense for chained methods. | ||
## Usage | ||
@@ -70,4 +51,2 @@ ### Initialize client with access token provider | ||
For more examples of accepted paths, see the [test cases](tests/urlParsing.js). | ||
```javascript | ||
@@ -178,3 +157,3 @@ // Example calling /me with no parameters | ||
.api('/me/drive/root/children/logo.png/content') // path to the destination in OneDrive | ||
.put(stream, (err) => { | ||
.putStream(stream, (err) => { | ||
console.log(err); | ||
@@ -271,2 +250,12 @@ }); | ||
## Running node samples | ||
You can run and debug the node samples found under [./samples/node/node-sample.js](./samples/node/node-sample.js) by running the *Run node samples* configuration from the **Debug** (Ctrl + Shift + D) menu in Visual Studio Code. Alternately, you can run the node samples from the CLI by entering `node ./samples/node/node-sample.js` (assuming you are at the root of this repo). You'll need to rename the *secrets.example.json* file to *secrets.json* and add a valid access token to it. You can get an access token by doing the following: | ||
1. Go to [Graph Explorer](https://developer.microsoft.com/en-us/graph/graph-explorer). | ||
2. Login with the account you want to use to run the node samples. | ||
3. Open the F12 dev tools. | ||
4. Type `tokenPlease()` into the console to get an access token. | ||
5. Copy the access token and put it into the *secrets.json* file and save the file. | ||
We suggest that you become acquainted with these samples as they show some of the main use scenarios for this client library. | ||
## Additional information | ||
@@ -316,3 +305,3 @@ ### Options in `MicrosoftGraph.Client.init()` | ||
```npm test``` runs tests of the core library (URL parsing, mock responses, etc) | ||
```npm test``` runs tests of the core library (URL parsing, mock responses, etc). You can also set breakpoints and run this from within Visual Studio Code by selecting the *Run core test* configuration from the Debug view. | ||
@@ -333,3 +322,26 @@ ```npm run test:types``` to run tests against the Graph API for users, groups, Excel, OneNote, etc. | ||
Please see the [contributing guidelines](CONTRIBUTING.md). | ||
## Changelog | ||
#### 1.0.0 | ||
* Added tests for new Graph functionality - Delta query, Extensibility, OneNote, and more. | ||
#### 0.4.0 | ||
* Add support for ES5. Make sure to use `graph-js-sdk-web.js` for web apps | ||
* Removed iterator helper method. | ||
#### 0.3.1 | ||
* Support for Node.js versions 4 and 5 | ||
#### 0.3.0 | ||
* Migrated away from typings in client library core and TypeScript sample | ||
#### 0.2.2 | ||
* Updated SuperAgent to version ``` 3.3.0 ``` | ||
#### 0.2.0 | ||
* **Breaking change for existing apps** - Initialize the client library with `MicrosoftGraph.Client.init({...})`. See the updated usage section below for code samples. | ||
* Added response handling tests to simulate Graph calls | ||
* Added type declarations file for core client library, which adds intellisense for chained methods. | ||
## Additional resources | ||
@@ -344,2 +356,7 @@ | ||
## Copyright | ||
Copyright (c) 2016 Microsoft. All rights reserved. | ||
Copyright (c) Microsoft Corporation. All rights reserved. | ||
This project has adopted the [Microsoft Open Source Code of Conduct](https://opensource.microsoft.com/codeofconduct/). For more information see the [Code of Conduct FAQ](https://opensource.microsoft.com/codeofconduct/faq/) or contact [opencode@microsoft.com](mailto:opencode@microsoft.com) with any additional questions or comments. | ||
## Third Party Notices | ||
See [Third Party Notices](https://github.com/microsoftgraph/msgraph-sdk-javascript/blob/master/THIRD%20PARTY%20NOTICES) for information on the packages that are included in the [package.json](https://github.com/microsoftgraph/msgraph-sdk-javascript/blob/master/package.json) |
Sorry, the diff of this file is too big to display
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
Network access
Supply chain riskThis module accesses the network.
Found 1 instance in 1 package
New author
Supply chain riskA new npm collaborator published a version of the package for the first time. New collaborators are usually benign additions to a project, but do indicate a change to the security surface area of a package.
Found 1 instance in 1 package
Uses eval
Supply chain riskPackage uses dynamic code execution (e.g., eval()), which is a dangerous practice. This can prevent the code from running in certain environments and increases the risk that the code may contain exploits or malicious behavior.
Found 1 instance in 1 package
Minified code
QualityThis package contains minified code. This may be harmless in some cases where minified code is included in packaged libraries, however packages on npm should not minify code.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Uses eval
Supply chain riskPackage uses dynamic code execution (e.g., eval()), which is a dangerous practice. This can prevent the code from running in certain environments and increases the risk that the code may contain exploits or malicious behavior.
Found 1 instance in 1 package
Long strings
Supply chain riskContains long string literals, which may be a sign of obfuscated or packed code.
Found 1 instance in 1 package
31
355
3
144574
6
763
2
8
+ Addedisomorphic-fetch@^2.2.1
+ Addedencoding@0.1.13(transitive)
+ Addediconv-lite@0.6.3(transitive)
+ Addedis-stream@1.1.0(transitive)
+ Addedisomorphic-fetch@2.2.1(transitive)
+ Addednode-fetch@1.7.3(transitive)
+ Addedsafer-buffer@2.1.2(transitive)
+ Addedwhatwg-fetch@3.6.20(transitive)
- Removedsuperagent@^3.5.2
- Removedasynckit@0.4.0(transitive)
- Removedcall-bind-apply-helpers@1.0.1(transitive)
- Removedcall-bound@1.0.3(transitive)
- Removedcombined-stream@1.0.8(transitive)
- Removedcomponent-emitter@1.3.1(transitive)
- Removedcookiejar@2.1.4(transitive)
- Removedcore-util-is@1.0.3(transitive)
- Removeddebug@3.2.7(transitive)
- Removeddelayed-stream@1.0.0(transitive)
- Removeddunder-proto@1.0.1(transitive)
- Removedes-define-property@1.0.1(transitive)
- Removedes-errors@1.3.0(transitive)
- Removedes-object-atoms@1.0.1(transitive)
- Removedextend@3.0.2(transitive)
- Removedform-data@2.5.2(transitive)
- Removedformidable@1.2.6(transitive)
- Removedfunction-bind@1.1.2(transitive)
- Removedget-intrinsic@1.2.7(transitive)
- Removedget-proto@1.0.1(transitive)
- Removedgopd@1.2.0(transitive)
- Removedhas-symbols@1.1.0(transitive)
- Removedhasown@2.0.2(transitive)
- Removedinherits@2.0.4(transitive)
- Removedisarray@1.0.0(transitive)
- Removedmath-intrinsics@1.1.0(transitive)
- Removedmethods@1.1.2(transitive)
- Removedmime@1.6.0(transitive)
- Removedmime-db@1.52.0(transitive)
- Removedmime-types@2.1.35(transitive)
- Removedms@2.1.3(transitive)
- Removedobject-inspect@1.13.3(transitive)
- Removedprocess-nextick-args@2.0.1(transitive)
- Removedqs@6.13.1(transitive)
- Removedreadable-stream@2.3.8(transitive)
- Removedsafe-buffer@5.1.25.2.1(transitive)
- Removedside-channel@1.1.0(transitive)
- Removedside-channel-list@1.0.0(transitive)
- Removedside-channel-map@1.0.1(transitive)
- Removedside-channel-weakmap@1.0.2(transitive)
- Removedstring_decoder@1.1.1(transitive)
- Removedsuperagent@3.8.3(transitive)
- Removedutil-deprecate@1.0.2(transitive)