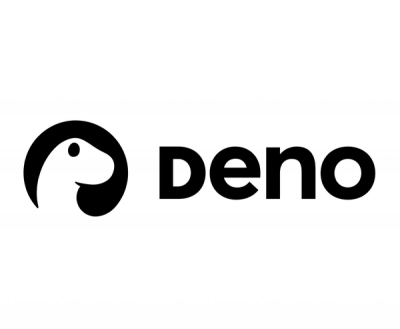
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@microsoft/teams-ai
Advanced tools
Welcome to the Teams AI SDK JavaScript package. See the Teams AI repo README.md, for general information, including updates on dotnet support.
Requirements:
If you're migrating an existing project, switching to add on the Teams AI layer is quick and simple. For a more-detailed walkthrough, see the migration guide. The basics are listed below.
In your existing Teams bot, you'll need to add the Teams AI SDK package and import it into your bot code.
yarn add @microsoft/teams-ai
#or
npm install @microsoft/teams-ai
Replace BotActivityHandler
and ApplicationTurnState
in your bot. Note that here, TurnState
is constructed to include ConversationState
, but can also have UserState
and TempState
.
js index.ts
:
// Old code:
// const bot = BotActivityHandler();
interface ConversationState {
count: number;
}
type ApplicationTurnState = TurnState<ConversationState>;
const app =
new Application<ApplicationTurnState>()
{
storage // in this case, MemoryStorage
};
The rest of the code, including server.post
and await app.run(context)
stays the same.
That's it!
Run your bot (with ngrok) and sideload your manifest to test.
For migrating specific features such as Message Extension and Adaptive Card capabilities, please see the Migration Guide.
If you are starting a new project, you can use the Teams AI SDK echobot sample as a starting point. You don't need to make any changes to the sample to get it running, but you can use it as your base setup. Echo Bot supports the Teams AI SDK out of the box.
You can either copy-paste the code into your own project, or clone the repo and run the Teams Toolkit features to explore.
You may also use the ApplicationBuilder
class to instantiate your Application
instance. This option provides greater readability and separates the management of the various configuration options (e.g., storage, turn state, AI module options, etc).
js index.ts
:
// Old method:
// const app = new Application()<ApplicationTurnState>
// {
// storage
// };
const app = new ApplicationBuilder()<ApplicationTurnState>
.withStorage(storage)
.build(); // this function internally calls the Application constructor
The detailed steps for setting up your bot to use AI are in the GPT Setup Guide.
On top of your Microsoft App Id and password, you will need an Azure OpenAI or OpenAI API key. You can get one from the OpenAI platform. Once you have your key, add it to your .env
file as OPEN_AI_KEY
const promptManager = new DefaultPromptManager<ApplicationTurnState>(path.join(__dirname, '../src/prompts'));
// Define storage and application
// - Note that we're not passing a prompt for our AI options as we won't be chatting with the app.
const storage = new MemoryStorage();
const app = new Application<ApplicationTurnState>({
storage,
adapter,
botAppId: process.env.MicrosoftAppId,
ai: {
planner,
promptManager
}
});
For more information on how to create and use prompts, see APIREFERENCE and look at the samples numbered 04._.xxx
).
Happy coding!
FAQs
SDK focused on building AI based applications for Microsoft Teams.
We found that @microsoft/teams-ai demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.