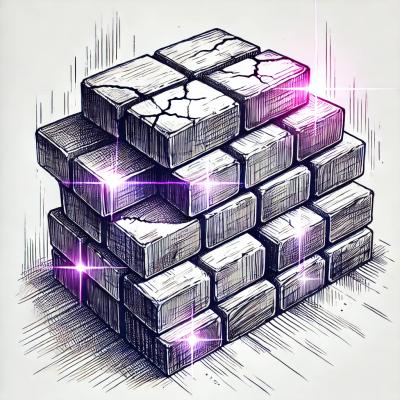
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
@mikro-orm/core
Advanced tools
TypeScript ORM for Node.js based on Data Mapper, Unit of Work and Identity Map patterns. Supports MongoDB, MySQL, PostgreSQL and SQLite databases as well as usage with vanilla JavaScript.
@mikro-orm/core is an Object-Relational Mapper (ORM) for Node.js and TypeScript. It provides a powerful and flexible way to interact with databases using JavaScript/TypeScript objects. It supports multiple database drivers, including MySQL, PostgreSQL, SQLite, and MongoDB. The package offers features like entity management, query building, migrations, and more.
Entity Management
Entity management allows you to define and manage your database entities using decorators. In this example, a `User` entity is defined with `id`, `name`, and `email` properties.
const { Entity, PrimaryKey, Property } = require('@mikro-orm/core');
@Entity()
class User {
@PrimaryKey()
id;
@Property()
name;
@Property()
email;
}
const user = new User();
user.name = 'John Doe';
user.email = 'john.doe@example.com';
Query Building
Query building allows you to perform database operations using a fluent API. In this example, a query is built to find all users with the name 'John Doe'.
const { MikroORM } = require('@mikro-orm/core');
async function main() {
const orm = await MikroORM.init({
entities: [User],
dbName: 'my-db-name',
type: 'postgresql',
});
const userRepository = orm.em.getRepository(User);
const users = await userRepository.find({ name: 'John Doe' });
console.log(users);
}
main();
Migrations
Migrations allow you to manage database schema changes over time. In this example, a migration is created and then applied to the database.
const { MikroORM } = require('@mikro-orm/core');
async function main() {
const orm = await MikroORM.init({
entities: [User],
dbName: 'my-db-name',
type: 'postgresql',
});
const migrator = orm.getMigrator();
await migrator.createMigration(); // creates file Migration20201019195930.ts
await migrator.up(); // runs all pending migrations
}
main();
TypeORM is another ORM for TypeScript and JavaScript (ES7, ES6, ES5). It supports multiple databases like MySQL, PostgreSQL, MariaDB, SQLite, and more. TypeORM is known for its active community and extensive documentation. Compared to @mikro-orm/core, TypeORM offers a similar feature set but with a different API and some additional features like Active Record pattern support.
Sequelize is a promise-based Node.js ORM for Postgres, MySQL, MariaDB, SQLite, and Microsoft SQL Server. It features solid transaction support, relations, eager and lazy loading, read replication, and more. Sequelize is widely used and has a large community. Compared to @mikro-orm/core, Sequelize is more mature and has broader database support but lacks some of the TypeScript-specific features.
Objection.js is an ORM for Node.js that aims to stay as close to the relational database as possible. It is built on top of the SQL query builder Knex.js. Objection.js is known for its simplicity and flexibility. Compared to @mikro-orm/core, Objection.js provides a more lightweight and less opinionated approach to ORM, making it suitable for developers who prefer more control over their SQL queries.
FAQs
TypeScript ORM for Node.js based on Data Mapper, Unit of Work and Identity Map patterns. Supports MongoDB, MySQL, PostgreSQL and SQLite databases as well as usage with vanilla JavaScript.
The npm package @mikro-orm/core receives a total of 199,388 weekly downloads. As such, @mikro-orm/core popularity was classified as popular.
We found that @mikro-orm/core demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.