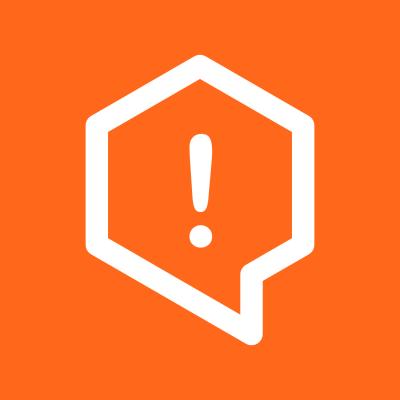
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
@mirrorworld/library.banksharedpool.new
Advanced tools
@mirrorworld/library.banksharedpool
Client SDKThis SDK contains the client side methods for the Bank Shared Pool Solana Program
🚨 Please make sure to add this NPM token in your
.npmrc
file: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
yarn add @mirrorworld/library.banksharedpool
Import the BankSharedPoolLib
instance into your client. It expects a connection
and wallet
instance. You can
get these
by using one of the Solana Wallet Adapters your application will use to connect to a Solana RPC.
These transactions require you to sign the transaction using your wallet. That means you need to have SOL. You can request SOL from the SolFaucet
import {
BankSharedPoolLib,
BANK_SHARED_POOL_PROGRAM_ID
} from '@mirrorworld/library.banksharedpool';
const connection = useConnection()
/** Make sure your wallet is initialized and connected to the browser before providing to BankSharedPoolLib */
const wallet = useWallet()
/** BankSharedPoolLib instance */
const bankSharedPoolLib = new BankSharedPoolLib(
BANK_SHARED_POOL_PROGRAM_ID,
connection,
wallet
);
Example: You can see example project in this repo here:
Main Config Account pda is the top level config for the program.
let tx = await bankSharedPoolLib.createInitializeMainConfigTransaction(mainSigningAuthorityWalletKeypair.publicKey, feePayerWalletKeypair.publicKey,
SystemProgram.programId, SYSVAR_RENT_PUBKEY);
bankSharedPoolLib.signTransaction(tx, feePayerWalletSecretKey);
bankSharedPoolLib.signTransaction(tx, mainSigningAuthorityWalletSecretKey);
let txHash = await connection.sendRawTransaction(tx.serialize(), {skipPreflight: false});
console.log("Tx Hash: ", txHash);
See example here
This transaction create bank config account pda from the bank name seed.
let tx = await bankSharedPoolLib.createBankConfigTransaction(bankName, bankSigningAuthorityWalletKeypair.publicKey,
feePayerWalletKeypair.publicKey, SystemProgram.programId, SYSVAR_RENT_PUBKEY);
bankSharedPoolLib.signTransaction(tx, feePayerWalletSecretKey);
bankSharedPoolLib.signTransaction(tx, bankSigningAuthorityWalletSecretKey);
let txHash = await connection.sendRawTransaction(tx.serialize(), {skipPreflight: false});
console.log("Tx Hash: ", txHash);
See example here
Create Sol Config transaction make the sol payment config for the bank.
const isMaxWithdrawEnable = false;
const isMinWithdrawEnable = false;
const maxWithdraw = 0.1 * LAMPORTS_PER_SOL;
const minWithdraw = 0.3 * LAMPORTS_PER_SOL;
let tx = await bankSharedPoolLib.createSolConfigTransaction(bankName, isMaxWithdrawEnable, isMinWithdrawEnable,
maxWithdraw, minWithdraw, incomeAccount, solSigningAuthorityWalletKeypair.publicKey, bankSigningAuthorityWalletKeypair.publicKey,
feePayerWalletKeypair.publicKey, SystemProgram.programId, SYSVAR_RENT_PUBKEY);
bankSharedPoolLib.signTransaction(tx, feePayerWalletSecretKey);
bankSharedPoolLib.signTransaction(tx, bankSigningAuthorityWalletSecretKey);
bankSharedPoolLib.signTransaction(tx, solSigningAuthorityWalletSecretKey);
let txHash = await connection.sendRawTransaction(tx.serialize(), {skipPreflight: false});
console.log("Tx Hash: ", txHash);
See example here
Update Sol Config transaction update the sol payment config for the bank, but sol signing authority can call this transaction.
const isMaxWithdrawEnable = false;
const isMinWithdrawEnable = false;
const maxWithdraw = 0.1 * LAMPORTS_PER_SOL;
const minWithdraw = 0.3 * LAMPORTS_PER_SOL;
let tx = await bankSharedPoolLib.createUpdateSolConfigTransaction(bankName, isMaxWithdrawEnable, isMinWithdrawEnable,
maxWithdraw, minWithdraw, incomeAccount, solSigningAuthorityWalletKeypair.publicKey, feePayerWalletKeypair.publicKey,
SystemProgram.programId, SYSVAR_RENT_PUBKEY);
bankSharedPoolLib.signTransaction(tx, feePayerWalletSecretKey);
bankSharedPoolLib.signTransaction(tx, solSigningAuthorityWalletSecretKey);
let txHash = await connection.sendRawTransaction(tx.serialize(), {skipPreflight: false});
console.log("Tx Hash: ", txHash);
See example here
This transaction add the sol in the distribution supply
let tx = await bankSharedPoolLib.createAddSolDistributionSupplyTransaction(bankName, amount, solSigningAuthorityWalletKeypair.publicKey, solSigningAuthorityWalletKeypair.publicKey,
feePayerWalletKeypair.publicKey, SystemProgram.programId, SYSVAR_RENT_PUBKEY);
bankSharedPoolLib.signTransaction(tx, feePayerWalletSecretKey);
bankSharedPoolLib.signTransaction(tx, solSigningAuthorityWalletSecretKey);
let txHash = await connection.sendRawTransaction(tx.serialize(), {skipPreflight: false});
console.log("Tx Hash: ", txHash);
See example here
This transaction add the sol in the deposit supply
let tx = await bankSharedPoolLib.createAddSolDepositSupplyTransaction(bankName, amount, solSigningAuthorityWalletKeypair.publicKey, solSigningAuthorityWalletKeypair.publicKey,
feePayerWalletKeypair.publicKey, SystemProgram.programId, SYSVAR_RENT_PUBKEY);
bankSharedPoolLib.signTransaction(tx, feePayerWalletSecretKey);
bankSharedPoolLib.signTransaction(tx, solSigningAuthorityWalletSecretKey);
let txHash = await connection.sendRawTransaction(tx.serialize(), {skipPreflight: false});
console.log("Tx Hash: ", txHash);
See example here
This transaction create user sol config pda if it is not created and add the sol in the deposit pool
let tx = await bankSharedPoolLib.createDepositSolTransaction(bankName, amount, userKeypair.publicKey, userKeypair.publicKey,
solSigningAuthorityWalletKeypair.publicKey, feePayerWalletKeypair.publicKey, SystemProgram.programId, SYSVAR_RENT_PUBKEY);
bankSharedPoolLib.signTransaction(tx, feePayerWalletSecretKey);
bankSharedPoolLib.signTransaction(tx, userSecretKey);
bankSharedPoolLib.signTransaction(tx, solSigningAuthorityWalletSecretKey);
let txHash = await connection.sendRawTransaction(tx.serialize(), {skipPreflight: false});
See example here
This transaction withdraw the sol from the deposit pool for the user
let tx = await bankSharedPoolLib.createWithdrawSolTransaction(bankName, amount, userKeypair.publicKey,
userKeypair.publicKey, solSigningAuthorityWalletKeypair.publicKey, feePayerWalletKeypair.publicKey, SystemProgram.programId, SYSVAR_RENT_PUBKEY);
bankSharedPoolLib.signTransaction(tx, feePayerWalletSecretKey);
bankSharedPoolLib.signTransaction(tx, userSecretKey);
bankSharedPoolLib.signTransaction(tx, solSigningAuthorityWalletSecretKey);
let txHash = await connection.sendRawTransaction(tx.serialize(), {skipPreflight: false});
console.log("Tx Hash: ", txHash);
See example here
This transaction withdraw the sol from the deposit pool for the user without user
let tx = await bankSharedPoolLib.createWithdrawSolWithoutUserTransaction(bankName, amount, userKeypair.publicKey, userKeypair.publicKey,
solSigningAuthorityWalletKeypair.publicKey, feePayerWalletKeypair.publicKey, SystemProgram.programId, SYSVAR_RENT_PUBKEY);
bankSharedPoolLib.signTransaction(tx, feePayerWalletSecretKey);
bankSharedPoolLib.signTransaction(tx, solSigningAuthorityWalletSecretKey);
let txHash = await connection.sendRawTransaction(tx.serialize(), {skipPreflight: false});
console.log("Tx Hash: ", txHash);
See example here
This Transaction Spend the sol from user deposit pool and add the in the income account
let tx = await bankSharedPoolLib.createSpendSolTransaction(bankName, amount, incomeAccount, solSigningAuthorityWalletKeypair.publicKey,
userKeypair.publicKey, feePayerWalletKeypair.publicKey, SystemProgram.programId, SYSVAR_RENT_PUBKEY);
bankSharedPoolLib.signTransaction(tx, feePayerWalletSecretKey);
bankSharedPoolLib.signTransaction(tx, userSecretKey);
bankSharedPoolLib.signTransaction(tx, solSigningAuthorityWalletSecretKey);
let txHash = await connection.sendRawTransaction(tx.serialize(), {skipPreflight: false});
console.log("Tx Hash: ", txHash);
See example here
This Transaction Spend the sol from user deposit pool and add the in the income account without user
let tx = await bankSharedPoolLib.createSpendSolWithoutUserTransaction(bankName, amount, incomeAccount, solSigningAuthorityWalletKeypair.publicKey,
userKeypair.publicKey, feePayerWalletKeypair.publicKey, SystemProgram.programId, SYSVAR_RENT_PUBKEY);
bankSharedPoolLib.signTransaction(tx, feePayerWalletSecretKey);
bankSharedPoolLib.signTransaction(tx, solSigningAuthorityWalletSecretKey);
let txHash = await connection.sendRawTransaction(tx.serialize(), {skipPreflight: false});
console.log("Tx Hash: ", txHash);
See example here
This transaction add the sol in the user deposit pool from the distribution pool
let tx = await bankSharedPoolLib.createDistributeSolTransaction(bankName, amount, solSigningAuthorityWalletKeypair.publicKey,
userKeypair.publicKey, feePayerWalletKeypair.publicKey, SystemProgram.programId, SYSVAR_RENT_PUBKEY);
bankSharedPoolLib.signTransaction(tx, feePayerWalletSecretKey);
bankSharedPoolLib.signTransaction(tx, userSecretKey);
bankSharedPoolLib.signTransaction(tx, solSigningAuthorityWalletSecretKey);
let txHash = await connection.sendRawTransaction(tx.serialize(), {skipPreflight: false});
console.log("Tx Hash: ", txHash);
See example here
This transaction add the sol in the user deposit pool from the distribution pool without user
let tx = await bankSharedPoolLib.createDistributeSolWithoutUserTransaction(bankName, amount, solSigningAuthorityWalletKeypair.publicKey,
userKeypair.publicKey, feePayerWalletKeypair.publicKey, SystemProgram.programId, SYSVAR_RENT_PUBKEY);
bankSharedPoolLib.signTransaction(tx, feePayerWalletSecretKey);
bankSharedPoolLib.signTransaction(tx, solSigningAuthorityWalletSecretKey);
let txHash = await connection.sendRawTransaction(tx.serialize(), {skipPreflight: false});
console.log("Tx Hash: ", txHash);
See example here
This transaction the creates the token config for the bank for a token mint account
const isMaxWithdrawEnable = false;
const isMinWithdrawEnable = false;
const maxWithdraw = 0.1 * LAMPORTS_PER_SOL;
const minWithdraw = 3 * LAMPORTS_PER_SOL;
let tx = await bankSharedPoolLib.createTokenConfigTransaction(tokenMintAccount, bankName, isMaxWithdrawEnable, isMinWithdrawEnable,
maxWithdraw, minWithdraw, incomeAccount, tokenSigningAuthorityWalletKeypair.publicKey, bankSigningAuthorityWalletKeypair.publicKey,
feePayerWalletKeypair.publicKey,
TOKEN_PROGRAM_ID, ASSOCIATED_TOKEN_PROGRAM_ID, SystemProgram.programId, SYSVAR_RENT_PUBKEY);
bankSharedPoolLib.signTransaction(tx, feePayerWalletSecretKey);
bankSharedPoolLib.signTransaction(tx, bankSigningAuthorityWalletSecretKey);
bankSharedPoolLib.signTransaction(tx, tokenSigningAuthorityWalletSecretKey);
let txHash = await connection.sendRawTransaction(tx.serialize(), {skipPreflight: false});
console.log("Tx Hash: ", txHash);
See example here
this transaction update the token config, only token signing authority can call this transaction
const isMaxWithdrawEnable = false;
const isMinWithdrawEnable = false;
const maxWithdraw = 0.1 * LAMPORTS_PER_SOL;
const minWithdraw = 3 * LAMPORTS_PER_SOL;
let tx = await bankSharedPoolLib.createUpdateTokenConfigTransaction(tokenMintAccount, bankName, isMaxWithdrawEnable, isMinWithdrawEnable,
maxWithdraw, minWithdraw, incomeAccount, tokenSigningAuthorityWalletKeypair.publicKey,
feePayerWalletKeypair.publicKey,
TOKEN_PROGRAM_ID, ASSOCIATED_TOKEN_PROGRAM_ID, SystemProgram.programId, SYSVAR_RENT_PUBKEY);
bankSharedPoolLib.signTransaction(tx, feePayerWalletSecretKey);
bankSharedPoolLib.signTransaction(tx, tokenSigningAuthorityWalletSecretKey);
let txHash = await connection.sendRawTransaction(tx.serialize(), {skipPreflight: false});
console.log("Tx Hash: ", txHash);
See example here
This transaction add the token in the distribution pool.
let tx = await bankSharedPoolLib.createAddTokenDistributionSupplyTransaction(tokenMintAccount, bankName, amount,
bankSigningAuthorityWalletKeypair.publicKey, tokenSigningAuthorityWalletKeypair.publicKey, feePayerWalletKeypair.publicKey,
TOKEN_PROGRAM_ID, ASSOCIATED_TOKEN_PROGRAM_ID, SystemProgram.programId, SYSVAR_RENT_PUBKEY);
bankSharedPoolLib.signTransaction(tx, feePayerWalletSecretKey);
bankSharedPoolLib.signTransaction(tx, tokenSigningAuthorityWalletSecretKey);
bankSharedPoolLib.signTransaction(tx, bankSigningAuthorityWalletSecretKey);
let txHash = await connection.sendRawTransaction(tx.serialize(), {skipPreflight: false});
console.log("Tx Hash: ", txHash);
See example here
This transaction add the token in the deposit pool.
let tx = await bankSharedPoolLib.createAddTokenDepositSupplyTransaction(tokenMintAccount, bankName, amount,
bankSigningAuthorityWalletKeypair.publicKey, tokenSigningAuthorityWalletKeypair.publicKey, feePayerWalletKeypair.publicKey,
TOKEN_PROGRAM_ID, ASSOCIATED_TOKEN_PROGRAM_ID, SystemProgram.programId, SYSVAR_RENT_PUBKEY);
bankSharedPoolLib.signTransaction(tx, feePayerWalletSecretKey);
bankSharedPoolLib.signTransaction(tx, tokenSigningAuthorityWalletSecretKey);
bankSharedPoolLib.signTransaction(tx, bankSigningAuthorityWalletSecretKey);
let txHash = await connection.sendRawTransaction(tx.serialize(), {skipPreflight: false});
console.log("Tx Hash: ", txHash);
See example here
This transaction create user token config if it is not created and add token in the deposit pool.
let tx = await bankSharedPoolLib.createDepositTokenTransaction(tokenMintAccount, bankName, amount,
userKeypair.publicKey, userKeypair.publicKey, tokenSigningAuthorityWalletKeypair.publicKey, feePayerWalletKeypair.publicKey,
TOKEN_PROGRAM_ID, ASSOCIATED_TOKEN_PROGRAM_ID, SystemProgram.programId, SYSVAR_RENT_PUBKEY);
bankSharedPoolLib.signTransaction(tx, feePayerWalletSecretKey);
bankSharedPoolLib.signTransaction(tx, userSecretKey);
bankSharedPoolLib.signTransaction(tx, tokenSigningAuthorityWalletSecretKey);
let txHash = await connection.sendRawTransaction(tx.serialize(), {skipPreflight: false});
console.log("Tx Hash: ", txHash);
See example here
This transaction withdraw token from the deposit pool for the user.
let tx = await bankSharedPoolLib.createWithdrawTokenTransaction(tokenMintAccount, bankName, amount,
userKeypair.publicKey, userKeypair.publicKey, tokenSigningAuthorityWalletKeypair.publicKey, feePayerWalletKeypair.publicKey,
TOKEN_PROGRAM_ID, ASSOCIATED_TOKEN_PROGRAM_ID, SystemProgram.programId, SYSVAR_RENT_PUBKEY);
bankSharedPoolLib.signTransaction(tx, feePayerWalletSecretKey);
bankSharedPoolLib.signTransaction(tx, userSecretKey);
bankSharedPoolLib.signTransaction(tx, tokenSigningAuthorityWalletSecretKey);
let txHash = await connection.sendRawTransaction(tx.serialize(), {skipPreflight: false});
console.log("Tx Hash: ", txHash);
See example here
This transaction withdraw token from the deposit pool for the user without user
let tx = await bankSharedPoolLib.createWithdrawTokenWithoutUserTransaction(tokenMintAccount, bankName, amount,
userKeypair.publicKey, userKeypair.publicKey, tokenSigningAuthorityWalletKeypair.publicKey, feePayerWalletKeypair.publicKey,
TOKEN_PROGRAM_ID, ASSOCIATED_TOKEN_PROGRAM_ID, SystemProgram.programId, SYSVAR_RENT_PUBKEY);
bankSharedPoolLib.signTransaction(tx, feePayerWalletSecretKey);
bankSharedPoolLib.signTransaction(tx, tokenSigningAuthorityWalletSecretKey);
let txHash = await connection.sendRawTransaction(tx.serialize(), {skipPreflight: false});
console.log("Tx Hash: ", txHash);
See example here
This transaction spend token from the user deposit pool and added in the income account.
let tx = await bankSharedPoolLib.createSpendTokenTransaction(tokenMintAccount, bankName, amount,
incomeAccount, tokenSigningAuthorityWalletKeypair.publicKey, userKeypair.publicKey, feePayerWalletKeypair.publicKey,
TOKEN_PROGRAM_ID, ASSOCIATED_TOKEN_PROGRAM_ID, SystemProgram.programId, SYSVAR_RENT_PUBKEY);
bankSharedPoolLib.signTransaction(tx, feePayerWalletSecretKey);
bankSharedPoolLib.signTransaction(tx, userSecretKey);
bankSharedPoolLib.signTransaction(tx, tokenSigningAuthorityWalletSecretKey);
let txHash = await connection.sendRawTransaction(tx.serialize(), {skipPreflight: false});
console.log("Tx Hash: ", txHash);
See example here
This transaction spend token from the user deposit pool and added in the income account without user.
let tx = await bankSharedPoolLib.createSpendTokenWithoutUserTransaction(tokenMintAccount, bankName, amount,
incomeAccount, tokenSigningAuthorityWalletKeypair.publicKey, userKeypair.publicKey, feePayerWalletKeypair.publicKey,
TOKEN_PROGRAM_ID, ASSOCIATED_TOKEN_PROGRAM_ID, SystemProgram.programId, SYSVAR_RENT_PUBKEY);
bankSharedPoolLib.signTransaction(tx, feePayerWalletSecretKey);
bankSharedPoolLib.signTransaction(tx, tokenSigningAuthorityWalletSecretKey);
let txHash = await connection.sendRawTransaction(tx.serialize(), {skipPreflight: false});
console.log("Tx Hash: ", txHash);
See example here
This transaction add the token in the user deposit pool from the distribution pool
let tx = await bankSharedPoolLib.createDistributeTokenTransaction(tokenMintAccount, bankName, amount,
tokenSigningAuthorityWalletKeypair.publicKey, userKeypair.publicKey, feePayerWalletKeypair.publicKey,
TOKEN_PROGRAM_ID, ASSOCIATED_TOKEN_PROGRAM_ID, SystemProgram.programId, SYSVAR_RENT_PUBKEY);
bankSharedPoolLib.signTransaction(tx, feePayerWalletSecretKey);
bankSharedPoolLib.signTransaction(tx, userSecretKey);
bankSharedPoolLib.signTransaction(tx, tokenSigningAuthorityWalletSecretKey);
let txHash = await connection.sendRawTransaction(tx.serialize(), {skipPreflight: false});
console.log("Tx Hash: ", txHash);
See example here
This transaction add the token in the user deposit pool from the distribution pool without user
let tx = await bankSharedPoolLib.createDistributeTokenWithoutUserTransaction(tokenMintAccount, bankName, amount,
tokenSigningAuthorityWalletKeypair.publicKey, userKeypair.publicKey, feePayerWalletKeypair.publicKey,
TOKEN_PROGRAM_ID, ASSOCIATED_TOKEN_PROGRAM_ID, SystemProgram.programId, SYSVAR_RENT_PUBKEY);
bankSharedPoolLib.signTransaction(tx, feePayerWalletSecretKey);
bankSharedPoolLib.signTransaction(tx, tokenSigningAuthorityWalletSecretKey);
let txHash = await connection.sendRawTransaction(tx.serialize(), {skipPreflight: false});
console.log("Tx Hash: ", txHash);
See example here
Name | Description |
---|---|
Main Config | Program config for the main pda account |
Bank Config | Program config for the bank pda account |
Sol Config | Program config for the sol pda account |
Token Config | Program config for the token pda account |
User Token Config | User config related to the token pda account |
User Sol Config | User config related to the sol pda account |
Deposit Pool | Deposit Pool pda account |
Deposit Pool Token Account | Deposit Pool pda token account |
Distribution Pool | Distribution Pool pda account |
Distribution Pool Token Account | Distribution Pool pda token account |
FAQs
bank shared pool SDK
The npm package @mirrorworld/library.banksharedpool.new receives a total of 2 weekly downloads. As such, @mirrorworld/library.banksharedpool.new popularity was classified as not popular.
We found that @mirrorworld/library.banksharedpool.new demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.