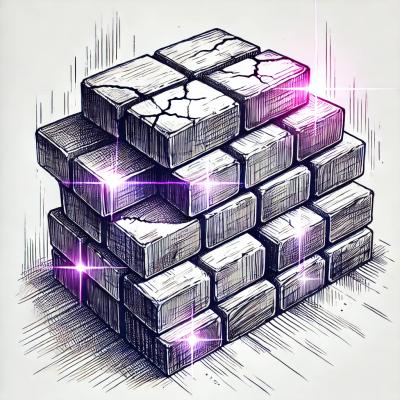
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
@miyauci/isx
Advanced tools
Collection of validation functions for JavaScript data.
This is a very small collection of validate functions. It provides a custom type guard whenever it can.
Module can be divided into two categories.
Top-type module can accept any JavaScript data. In other words, it accepts the
unknown
type, which is top-type.
Most of them can be used to identify the type by a type guard.
The module directly under namespace is it.
Sub-type modules are modules that perform type-dependent operations. It can use type-specific methods and compare values.
For example, the module under number
is a sub-type module that takes a
number
type as an argument.
Whether the input is string
or not.
import { isString } from "https://deno.land/x/isx@$VERSION/is_string.ts";
import { assertEquals } from "https://deno.land/std/testing/asserts.ts";
assertEquals(isString("hello world"), true);
assertEquals(isString(1000), false);
Whether the input is number
or not.
import { isNumber } from "https://deno.land/x/isx@$VERSION/is_number.ts";
import { assertEquals } from "https://deno.land/std/testing/asserts.ts";
assertEquals(isNumber(1000), true);
assertEquals(isNumber("hello world"), false);
Whether the input is bigint
or not.
import { isBigint } from "https://deno.land/x/isx@$VERSION/is_bigint.ts";
import { assertEquals } from "https://deno.land/std/testing/asserts.ts";
assertEquals(isBigint(1000n), true);
assertEquals(isBigint(undefined), false);
Whether the input is null
or not.
import { isNull } from "https://deno.land/x/isx@$VERSION/is_null.ts";
import { assertEquals } from "https://deno.land/std/testing/asserts.ts";
assertEquals(isNull(null), true);
assertEquals(isNull(undefined), false);
Whether the input is undefined
or not.
import { isUndefined } from "https://deno.land/x/isx@$VERSION/is_undefined.ts";
import { assertEquals } from "https://deno.land/std/testing/asserts.ts";
assertEquals(isUndefined(undefined), true);
assertEquals(isUndefined(null), false);
Whether the input is boolean
or not.
import { isBoolean } from "https://deno.land/x/isx@$VERSION/is_boolean.ts";
import { assertEquals } from "https://deno.land/std/testing/asserts.ts";
assertEquals(isBoolean(true), true);
assertEquals(isBoolean(null), false);
Whether the input is Function
or not.
import { isFunction } from "https://deno.land/x/isx@$VERSION/is_function.ts";
import { assertEquals } from "https://deno.land/std/testing/asserts.ts";
assertEquals(isFunction(() => {}), true);
assertEquals(isFunction({}), false);
Whether the input is object
or not.
import { isObject } from "https://deno.land/x/isx@$VERSION/is_object.ts";
import { assertEquals } from "https://deno.land/std/testing/asserts.ts";
assertEquals(isObject({}), true);
assertEquals(isObject(null), false);
Whether the input is symbol
or not.
import { isSymbol } from "https://deno.land/x/isx@$VERSION/is_symbol.ts";
import { assertEquals } from "https://deno.land/std/testing/asserts.ts";
assertEquals(isSymbol(Symbol("symbol")), true);
assertEquals(isSymbol(null), false);
Whether the input is null
or undefined
or not.
import { isNullable } from "https://deno.land/x/isx@$VERSION/is_nullable.ts";
import { assertEquals } from "https://deno.land/std/testing/asserts.ts";
assertEquals(isNullable(null), true);
assertEquals(isNullable(undefined), true);
assertEquals(isNullable({}), false);
Whether the input is Primitive
or not.
import { isPrimitive } from "https://deno.land/x/isx@$VERSION/is_primitive.ts";
import { assertEquals } from "https://deno.land/std/testing/asserts.ts";
assertEquals(isPrimitive(true), true);
assertEquals(isPrimitive(() => {}), false);
type Primitive =
| number
| string
| boolean
| bigint
| undefined
| null
| symbol;
Whether the input is array or not.
Use only if input contains ReadOnlyArray
. It improves type inference.
Otherwise, use Array.isArray
.
This exists only because of TypeScript bug #17002. When this is fixed, this function will no longer be provided.
import { isArray } from "https://deno.land/x/isx@$VERSION/is_array.ts";
import { assertEquals } from "https://deno.land/std/testing/asserts.ts";
assertEquals(isArray([]), true);
assertEquals(isArray({}), false);
Whether the input is Promise
or not.
import { isPromise } from "https://deno.land/x/isx@$VERSION/is_promise.ts";
import { assertEquals } from "https://deno.land/std/testing/asserts.ts";
assertEquals(isPromise(Promise.resolve()), true);
assertEquals(isPromise({}), false);
Whether the input is Date
or not.
import { isDate } from "https://deno.land/x/isx@$VERSION/is_date.ts";
import { assertEquals } from "https://deno.land/std/testing/asserts.ts";
assertEquals(isDate(new Date()), true);
assertEquals(isDate({}), false);
Whether the input is Error
or not.
import { isError } from "https://deno.land/x/isx@$VERSION/is_error.ts";
import { assertEquals } from "https://deno.land/std/testing/asserts.ts";
assertEquals(isError(Error()), true);
assertEquals(isError(new SyntaxError()), true);
assertEquals(isError(new Date()), false);
Whether the input is Iterable
or not.
import { isIterable } from "https://deno.land/x/isx@$VERSION/is_iterable.ts";
import { assertEquals } from "https://deno.land/std/testing/asserts.ts";
assertEquals(isIterable(""), true);
assertEquals(isIterable({}), false);
Whether the input is NonNullable
or not.
import { isNonNullable } from "https://deno.land/x/isx@$VERSION/is_non_nullable.ts";
import { assertEquals } from "https://deno.land/std/testing/asserts.ts";
assertEquals(isNonNullable(""), true);
assertEquals(isNonNullable(null), false);
assertEquals(isNonNullable(undefined), false);
Whether the input is AsyncIterable
or not.
import { isAsyncIterable } from "https://deno.land/x/isx@$VERSION/is_async_iterable.ts";
import { assertEquals } from "https://deno.land/std/testing/asserts.ts";
assertEquals(
isAsyncIterable({
async *[Symbol.asyncIterator]() {
yield "hello";
},
}),
true,
);
assertEquals(isAsyncIterable(() => {}), false);
Whether the input is RegExp
of not.
import { isRegExp } from "https://deno.land/x/isx@$VERSION/is_reg_exp.ts";
import { assertEquals } from "https://deno.land/std/testing/asserts.ts";
assertEquals(isRegExp(new RegExp("")), true);
assertEquals(isRegExp({}), false);
Validates a subtype of number
. All validate functions must satisfy ⊂ number
.
Whether the input is odd or not.
import { isOdd } from "https://deno.land/x/isx@$VERSION/number/is_odd.ts";
import { assertEquals } from "https://deno.land/std/testing/asserts.ts";
assertEquals(isOdd(1), true);
assertEquals(isOdd(0), false);
Whether the input is even or not.
import { isEven } from "https://deno.land/x/isx@$VERSION/number/is_even.ts";
import { assertEquals } from "https://deno.land/std/testing/asserts.ts";
assertEquals(isEven(0), true);
assertEquals(isEven(1), false);
Whether the input is positive number or not.
import { isPositiveNumber } from "https://deno.land/x/isx@$VERSION/number/is_positive_number.ts";
import { assertEquals } from "https://deno.land/std/testing/asserts.ts";
assertEquals(isPositiveNumber(1), true);
assertEquals(isPositiveNumber(0), false);
Whether the input is non-positive number or not. Non-positive number means less than or equal to zero.
import { isNonPositiveNumber } from "https://deno.land/x/isx@$VERSION/number/is_non_positive_number.ts";
import { assertEquals } from "https://deno.land/std/testing/asserts.ts";
assertEquals(isNonPositiveNumber(0), true);
assertEquals(isNonPositiveNumber(-1), true);
assertEquals(isNonPositiveNumber(1), false);
Whether the input is negative number or not.
import { isNegativeNumber } from "https://deno.land/x/isx@$VERSION/number/is_negative_number.ts";
import { assertEquals } from "https://deno.land/std/testing/asserts.ts";
assertEquals(isNegativeNumber(-1), true);
assertEquals(isNegativeNumber(0), false);
Whether the input is non-negative number or not. Non-negative number means greater than or equal to zero.
import { isNonNegativeNumber } from "https://deno.land/x/isx@$VERSION/number/is_non_negative_number.ts";
import { assertEquals } from "https://deno.land/std/testing/asserts.ts";
assertEquals(isNonNegativeNumber(0), true);
assertEquals(isNonNegativeNumber(1), true);
assertEquals(isNonNegativeNumber(-1), false);
Whether the input is non negative integer or not.
import { isNonNegativeInteger } from "https://deno.land/x/isx@$VERSION/number/is_non_negative_integer.ts";
import { assertEquals } from "https://deno.land/std/testing/asserts.ts";
assertEquals(isNonNegativeInteger(0), true);
assertEquals(isNonNegativeInteger(1.0), true);
assertEquals(isNonNegativeInteger(-1), false);
Whether the input is unit interval or not. The unit interval refers to the interval between 0 and 1 on the real number line.
import { isUnitInterval } from "https://deno.land/x/isx@$VERSION/number/is_unit_interval.ts";
import { assertEquals } from "https://deno.land/std/testing/asserts.ts";
assertEquals(isUnitInterval(0), true);
assertEquals(isUnitInterval(1.0), true);
assertEquals(isUnitInterval(-1), false);
Validates a subtype of Iterable
. All validate functions must satisfy ⊂
Iterable<unknown>
.
Wether the input is empty or not.
import { isEmpty } from "https://deno.land/x/isx@$VERSION/iterable/is_empty.ts";
import { assert } from "https://deno.land/std/testing/asserts.ts";
assert(isEmpty(""));
assert(isEmpty([]));
assert(isEmpty(new Set()));
string:
If the input is a string, it has a ""
type guard.
array:
If the input is a array, it has a []
type guard.
Whether the input is not empty or not.
import { isNotEmpty } from "https://deno.land/x/isx@$VERSION/iterable/is_not_empty.ts";
import { assert } from "https://deno.land/std/testing/asserts.ts";
assert(isNotEmpty("a"));
assert(isNotEmpty([0, 1]));
array:
If the input is a T[]
, it has a [T, ...T[]]
type guard.
Whether the input is single element or not.
import { isSingle } from "https://deno.land/x/isx@$VERSION/iterable/is_single.ts";
import { assert, assertFalse } from "https://deno.land/std/testing/asserts.ts";
assert(isSingle("a"));
assert(isSingle([0]));
assertFalse(isSingle([0, 1, 2]));
array:
If the input is a T[]
, it has a [T]
type guard.
Validates a subtype of Date
. All validate functions must satisfy ⊂ Date
.
Whether the input is valid Date
or not.
import { isValidDate } from "https://deno.land/x/isx@$VERSION/date/is_valid_date.ts";
import { assertEquals } from "https://deno.land/std/testing/asserts.ts";
assertEquals(isValidDate(new Date("2000/1/1")), true);
assertEquals(isValidDate(new Date("invalid")), false);
Bundle size is not exact. It is only a guide.
Usually, the actual bundle size is smaller than the indicated value.
There is no single entry point such as mod
.
This prevents the inclusion of many unnecessary modules.
Copyright © 2023-present Tomoki Miyauchi.
Released under the MIT license
FAQs
Collection of validation functions for JavaScript data
We found that @miyauci/isx demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.