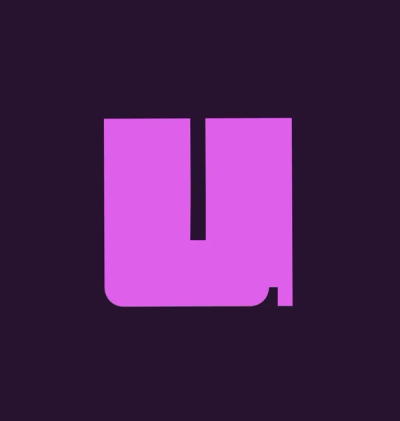
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
@monteway/codeshift
Advanced tools
Internal jscodeshift helpers used in @monteway/app for easier codemod implementation.
npm i -D @monteway/codeshift
appendImports
Adds new import inside imports group. You can specify where the import should go, by selecting an existing import inside source file and set if the new one should go before of after it.
Let's have index.tsx
file with following content:
import ReactDOM from 'react-dom';
// some more content that is irrelevant
Now write a codemod file codeshift.mjs
that will be run using jscodeshift.
In this example we want to add a React default import before react-dom
import.
import { appendImports } from '@monteway/codeshift';
export default function codemod(file, api, options) {
const jscodeshift = api.jscodeshift;
let source = jscodeshift(file.source);
source = appendImports(
jscodeshift,
source,
'BEFORE_FIRST',
/^react-dom$/,
`import React from 'react';`,
);
return source.toSource();
}
The output of running codeshift.mjs
for index.tsx
content would be:
+ import React from 'react';
import ReactDOM from 'react-dom';
// some more content that is irrelevant
wrapDefaultExport
Finds a default export and wraps it with a call experssion.
Let's have a index.tsx
file with the following content:
import React from 'react';
function Component() {
return <>Hello, world!</>;
}
export default Component;
Now write a codemod file codeshift.mjs
that will be run using jscodeshift.
In this example we want to wrap the Component
with memo
.
import { wrapDefaultExport } from '@monteway/codeshift';
export default function codemod(file, api, options) {
const jscodeshift = api.jscodeshift;
let source = jscodeshift(file.source);
source = wrapDefaultExport(jscodeshift, source, 'memo');
return source.toSource();
}
The output of running codeshift.mjs
for index.tsx
content would be:
import React from 'react';
function Component() {
return <>Hello, world!</>;
}
- export default Component;
+ export default memo(Component);
Notice that for this code to run we would need to add import for
memo
. We could solve it by usingReact.memo
instead ofmemo
, sinceReact
is already imported, but right now, thewrapDefaultExport
does not accept anything other than identifier node, so we could not useReact.memo
, since this a member experssion, not an identifier.
FAQs
Helpers for writing codemods
We found that @monteway/codeshift demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.