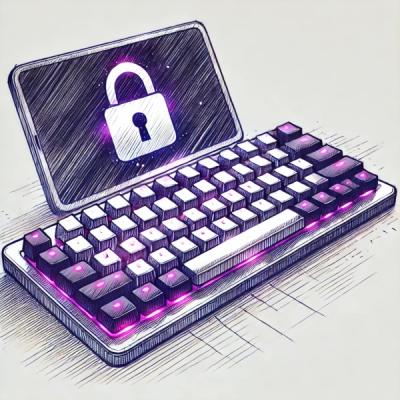
Research
Security News
Malicious npm Package Typosquats react-login-page to Deploy Keylogger
Socket researchers unpack a typosquatting package with malicious code that logs keystrokes and exfiltrates sensitive data to a remote server.
@nestjs/cqrs
Advanced tools
Package description
@nestjs/cqrs is a package for the NestJS framework that provides tools to implement the Command Query Responsibility Segregation (CQRS) pattern. This pattern separates read and write operations to simplify complex business logic and improve performance. The package also supports event sourcing, which allows you to store the state of an application as a sequence of events.
Commands
Commands are used to encapsulate a request to perform an action. The `CreateUserCommand` class represents a command to create a user, and the `CreateUserHandler` class handles the execution of this command.
```typescript
import { CommandHandler, ICommand, ICommandHandler } from '@nestjs/cqrs';
export class CreateUserCommand implements ICommand {
constructor(public readonly name: string) {}
}
@CommandHandler(CreateUserCommand)
export class CreateUserHandler implements ICommandHandler<CreateUserCommand> {
async execute(command: CreateUserCommand) {
console.log(`Creating user with name: ${command.name}`);
// Add your business logic here
}
}
```
Queries
Queries are used to encapsulate a request to retrieve data. The `GetUserQuery` class represents a query to get a user by ID, and the `GetUserHandler` class handles the execution of this query.
```typescript
import { IQuery, IQueryHandler, QueryHandler } from '@nestjs/cqrs';
export class GetUserQuery implements IQuery {
constructor(public readonly id: string) {}
}
@QueryHandler(GetUserQuery)
export class GetUserHandler implements IQueryHandler<GetUserQuery> {
async execute(query: GetUserQuery) {
console.log(`Fetching user with id: ${query.id}`);
// Add your business logic here
}
}
```
Events
Events are used to notify the system that something has happened. The `UserCreatedEvent` class represents an event that a user has been created, and the `UserCreatedHandler` class handles this event.
```typescript
import { EventsHandler, IEvent, IEventHandler } from '@nestjs/cqrs';
export class UserCreatedEvent implements IEvent {
constructor(public readonly userId: string) {}
}
@EventsHandler(UserCreatedEvent)
export class UserCreatedHandler implements IEventHandler<UserCreatedEvent> {
handle(event: UserCreatedEvent) {
console.log(`User created with id: ${event.userId}`);
// Add your business logic here
}
}
```
Sagas
Sagas are used to manage long-running transactions and orchestrate multiple events and commands. The `UserSagas` class listens for `UserCreatedEvent` and triggers a `CreateUserCommand` in response.
```typescript
import { Injectable } from '@nestjs/common';
import { Saga } from '@nestjs/cqrs';
import { Observable } from 'rxjs';
import { map } from 'rxjs/operators';
import { UserCreatedEvent } from './events/user-created.event';
import { CreateUserCommand } from './commands/create-user.command';
@Injectable()
export class UserSagas {
@Saga()
userCreated = (events$: Observable<any>): Observable<ICommand> => {
return events$.ofType(UserCreatedEvent).pipe(
map(event => new CreateUserCommand(event.userId))
);
};
}
```
Redux is a predictable state container for JavaScript apps, commonly used with React. It follows a similar pattern to CQRS by separating actions (commands) and reducers (handlers). However, Redux is more focused on client-side state management, whereas @nestjs/cqrs is designed for server-side applications.
EventEmitter2 is an implementation of the EventEmitter found in Node.js. It provides a way to handle events and listeners, similar to the event handling in @nestjs/cqrs. However, it does not provide the full CQRS pattern with commands and queries.
RxJS is a library for reactive programming using Observables, to make it easier to compose asynchronous or callback-based code. While RxJS itself is not a CQRS library, it is often used in conjunction with @nestjs/cqrs for handling asynchronous events and streams.
Readme
A progressive Node.js framework for building efficient and scalable server-side applications.
A lightweight CQRS module for Nest framework (node.js)
$ npm install --save @nestjs/cqrs
Nest is an MIT-licensed open source project. It can grow thanks to the sponsors and support by the amazing backers. If you'd like to join them, please read more here.
Nest is MIT licensed.
FAQs
Unknown package
We found that @nestjs/cqrs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers unpack a typosquatting package with malicious code that logs keystrokes and exfiltrates sensitive data to a remote server.
Security News
The JavaScript community has launched the e18e initiative to improve ecosystem performance by cleaning up dependency trees, speeding up critical parts of the ecosystem, and documenting lighter alternatives to established tools.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.