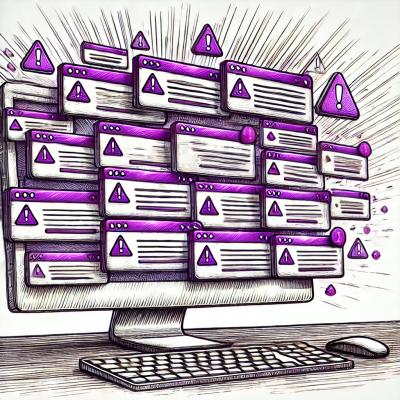
Security News
Combatting Alert Fatigue by Prioritizing Malicious Intent
In 2023, data breaches surged 78% from zero-day and supply chain attacks, but developers are still buried under alerts that are unable to prevent these threats.
@nestjs/mongoose
Advanced tools
@nestjs/mongoose is an integration package for NestJS that allows you to use Mongoose, a popular MongoDB object modeling tool, within your NestJS applications. It provides decorators and utilities to easily define and manage MongoDB schemas and models, and integrates seamlessly with NestJS's dependency injection system.
Schema Definition
This feature allows you to define MongoDB schemas using Mongoose. The code sample demonstrates how to define a simple schema for a 'Cat' model with fields for name, age, and breed.
const mongoose = require('mongoose');
const { Schema } = mongoose;
const CatSchema = new Schema({
name: String,
age: Number,
breed: String
});
module.exports = mongoose.model('Cat', CatSchema);
Model Injection
This feature allows you to inject Mongoose models into your NestJS services and controllers. The code sample shows how to create a CatsModule that imports the Cat model and makes it available for dependency injection.
import { Module } from '@nestjs/common';
import { MongooseModule } from '@nestjs/mongoose';
import { CatSchema } from './schemas/cat.schema';
import { CatsService } from './cats.service';
import { CatsController } from './cats.controller';
@Module({
imports: [MongooseModule.forFeature([{ name: 'Cat', schema: CatSchema }])],
controllers: [CatsController],
providers: [CatsService],
})
export class CatsModule {}
CRUD Operations
This feature allows you to perform CRUD operations on your MongoDB collections. The code sample demonstrates how to create a CatsService that can create and find all Cat documents in the MongoDB collection.
import { Injectable } from '@nestjs/common';
import { InjectModel } from '@nestjs/mongoose';
import { Model } from 'mongoose';
import { Cat } from './interfaces/cat.interface';
import { CreateCatDto } from './dto/create-cat.dto';
@Injectable()
export class CatsService {
constructor(@InjectModel('Cat') private readonly catModel: Model<Cat>) {}
async create(createCatDto: CreateCatDto): Promise<Cat> {
const createdCat = new this.catModel(createCatDto);
return createdCat.save();
}
async findAll(): Promise<Cat[]> {
return this.catModel.find().exec();
}
}
Mongoose is a MongoDB object modeling tool designed to work in an asynchronous environment. It provides a straightforward, schema-based solution to model your application data. While @nestjs/mongoose integrates Mongoose with NestJS, Mongoose itself can be used independently or with other frameworks.
Typegoose is a library that makes it easier to use Mongoose with TypeScript by defining Mongoose models using TypeScript classes. It provides type safety and integrates well with TypeScript's type system. Compared to @nestjs/mongoose, Typegoose focuses more on TypeScript integration rather than NestJS-specific features.
NestJS-Typegoose is a NestJS module for Typegoose, combining the benefits of Typegoose with the structure and features of NestJS. It provides decorators and utilities to define and manage Typegoose models within a NestJS application, similar to how @nestjs/mongoose works with Mongoose.
A progressive Node.js framework for building efficient and scalable server-side applications.
$ npm i --save @nestjs/mongoose mongoose
Nest is an MIT-licensed open source project. It can grow thanks to the sponsors and support by the amazing backers. If you'd like to join them, please read more here.
Nest is MIT licensed.
FAQs
Nest - modern, fast, powerful node.js web framework (@mongoose)
The npm package @nestjs/mongoose receives a total of 338,549 weekly downloads. As such, @nestjs/mongoose popularity was classified as popular.
We found that @nestjs/mongoose demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
In 2023, data breaches surged 78% from zero-day and supply chain attacks, but developers are still buried under alerts that are unable to prevent these threats.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.