What is @nestjs/websockets?
@nestjs/websockets is a module for the NestJS framework that provides WebSocket capabilities. It allows developers to create real-time, event-driven applications using WebSockets, which are essential for applications requiring instant data updates, such as chat applications, live notifications, and online gaming.
What are @nestjs/websockets's main functionalities?
WebSocket Gateway
This feature allows you to create a WebSocket gateway that listens for incoming WebSocket connections and messages. The `@WebSocketGateway` decorator is used to define a WebSocket gateway, and the `@SubscribeMessage` decorator is used to handle specific message events.
```typescript
import { WebSocketGateway, WebSocketServer, SubscribeMessage, MessageBody } from '@nestjs/websockets';
import { Server } from 'socket.io';
@WebSocketGateway()
export class ChatGateway {
@WebSocketServer()
server: Server;
@SubscribeMessage('message')
handleMessage(@MessageBody() message: string): void {
this.server.emit('message', message);
}
}
```
WebSocket Client
This feature allows you to create a WebSocket client that can connect to a WebSocket server. The `@WebSocketClient` decorator is used to define a WebSocket client, and the `OnGatewayInit`, `OnGatewayConnection`, and `OnGatewayDisconnect` interfaces are used to handle client lifecycle events.
```typescript
import { Injectable } from '@nestjs/common';
import { WebSocketClient, OnGatewayInit, OnGatewayConnection, OnGatewayDisconnect } from '@nestjs/websockets';
import { Socket } from 'socket.io-client';
@Injectable()
@WebSocketClient()
export class ChatClient implements OnGatewayInit, OnGatewayConnection, OnGatewayDisconnect {
private client: Socket;
afterInit(client: Socket) {
this.client = client;
console.log('WebSocket client initialized');
}
handleConnection(client: Socket) {
console.log('Client connected:', client.id);
}
handleDisconnect(client: Socket) {
console.log('Client disconnected:', client.id);
}
}
```
Custom WebSocket Server
This feature allows you to create a custom WebSocket server using the `ws` library. The `@WebSocketGateway` decorator can accept a custom server instance, and you can handle connection and message events directly.
```typescript
import { WebSocketGateway, WebSocketServer } from '@nestjs/websockets';
import { Server } from 'ws';
@WebSocketGateway({ server: new Server({ port: 8080 }) })
export class CustomGateway {
@WebSocketServer()
server: Server;
constructor() {
this.server.on('connection', (socket) => {
socket.on('message', (message) => {
console.log('Received message:', message);
});
});
}
}
```
Other packages similar to @nestjs/websockets
socket.io
Socket.IO is a popular library for real-time web applications. It enables real-time, bidirectional, and event-based communication between web clients and servers. Compared to @nestjs/websockets, Socket.IO is more general-purpose and can be used with various frameworks, while @nestjs/websockets is specifically designed for use with the NestJS framework.
ws
The `ws` package is a simple and fast WebSocket library for Node.js. It provides a bare-bones WebSocket server and client implementation. Compared to @nestjs/websockets, `ws` is lower-level and requires more manual setup, whereas @nestjs/websockets provides a more integrated and higher-level API within the NestJS framework.
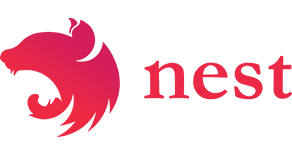
Modern, powerful web application framework for Node.js.

Description
Nest is a powerful web framework for Node.js, which helps you effortlessly build efficient, scalable applications. It uses modern JavaScript, is built with TypeScript and combines best concepts of both OOP (Object Oriented Progamming) and FP (Functional Programming).
It is not just another framework. You do not have to wait for a large community, because Nest is built with awesome, popular well-known libraries - Express and socket.io! It means, that you could quickly start using framework without worrying about a third party plugins.
Installation
Git:
$ git clone https://github.com/kamilmysliwiec/nest-typescript-starter.git project
$ cd project
$ npm install
$ npm run start
NPM:
$ npm i --save @nestjs/core @nestjs/common @nestjs/microservices @nestjs/websockets @nestjs/testing reflect-metadata rxjs redis
Philosophy
JavaScript is awesome. This language is no longer just a trash to create simple animations in the browser. Right now, the front end world is rich in variety of tools. We have a lot of amazing frameworks / libraries such as Angular, React or Vue, which improves our development process and makes our applications fast and flexible.
Node.js gave us a possibility to use this language also on the server side. There are a lot of superb libraries, helpers and tools for node, but non of them do not solve the main problem - the architecture.
We want to create scalable, modern and easy to maintain applications. Nest helps us with it.
Features
- Easy to learn - syntax is similar to Angular
- Compatible with both TypeScript and ES6 (I strongly recommend to use TypeScript)
- Based on well-known libraries (Express / socket.io) so you could share your experience
- Supremely useful Dependency Injection, built-in Inversion of Control container
- Hierarchical injector - increase abstraction in your application by creating reusable modules with type injection
- Own modularity system (split your system into reusable modules)
- WebSockets module (based on socket.io)
- Reactive microservices support with messages patterns (transport via TCP / Redis)
- Exceptions handler layer
- Testing utilities
Documentation & Quick Start
Documentation & Tutorial
Starter repos
Modules
Examples
People
License
MIT