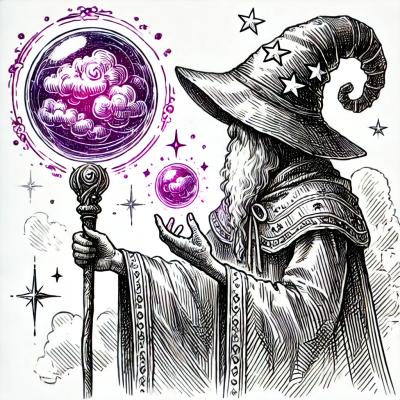
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
@netlify/cache-utils
Advanced tools
Utility for caching files in Netlify Build.
// Restore file/directory cached in previous builds.
// Does not do anything if:
// - the file/directory already exists locally
// - the file/directory has not been cached yet
export const onPreBuild = async function ({ utils }) {
await utils.cache.restore('./path/to/file')
}
// Cache file/directory for future builds.
// Does not do anything if:
// - the file/directory does not exist locally
export const onPostBuild = async function ({ utils }) {
await utils.cache.save('./path/to/file')
}
// Restore/cache several files/directories
export const onPreBuild = async function ({ utils }) {
await utils.cache.restore(['./path/to/file', './path/to/other'])
}
export const onPostBuild = async function ({ utils }) {
await utils.cache.save(['./path/to/file', './path/to/other'])
}
path
: string
options
: object
Returns: Promise<Boolean>
Cache a file/directory.
Skipped if the file/directory does not exist locally.
Returns false
if the file/directory does not exist. Returns true
otherwise.
Type: number
(in seconds)
Default: undefined
Only cache the file/directory for a specific amount of time.
// Only cache the following file/directory for 1 hour
export const onPreBuild = async function ({ utils }) {
await utils.cache.restore('./path/to/file')
}
export const onPostBuild = async function ({ utils }) {
const ttl = 3600
await utils.cache.save('./path/to/file', { ttl })
}
Type: string[]
Default: []
Paths to lock files or manifest files that can be used to check if the directory to cache has changed. Using this option speeds up caching.
// If that directory has a lockfile or a manifest file, use it to check if its
// contents has changed. This will speed up cache saving.
// For example, `package-lock.json` and `yarn.lock` are digest files for the
// `node_modules` directory.
export const onPreBuild = async function ({ utils }) {
await utils.cache.restore('node_modules')
}
export const onPostBuild = async function ({ utils }) {
await utils.cache.save('node_modules', {
digests: ['package-lock.json', 'yarn.lock'],
})
}
Type: string
Default: process.cwd()
Current directory used to resolve relative paths.
path
: string
options
: object
Returns: Promise<Boolean>
Restore a file/directory previously cached. Skipped if the file/directory already exists locally or if it has not been cached yet.
Please be careful: this overwrites the local file/directory if any exists.
Returns false
if the file/directory was not cached yet. Returns true
otherwise.
Type: string
Default: process.cwd()
Current directory used to resolve relative paths.
path
: string
Returns: Promise<Boolean>
Remove a file/directory from the cache. Useful for cache invalidation.
Returns false
if the file/directory was not cached yet. Returns true
otherwise.
export const onPostBuild = async function ({ utils }) {
await utils.cache.remove('./path/to/file')
}
Type: string
Default: process.cwd()
Current directory used to resolve relative paths.
path
: string
Returns: Promise<Boolean>
Returns whether a file/directory is currently cached.
// Conditional logic can be applied depending on whether the file has been
// previously cached or not
const path = './path/to/file'
export const onPreBuild = async function ({ utils }) {
if (!(await utils.cache.has(path))) {
console.log(`File ${path} not cached`)
return
}
console.log(`About to restore cached file ${path}...`)
if (await utils.cache.restore('./path/to/file')) {
console.log(`Restored cached file ${path}`)
}
}
export const onPostBuild = async function ({ utils }) {
if (await utils.cache.save('./path/to/file')) {
console.log(`Saved cached file ${path}`)
}
}
Type: string
Default: process.cwd()
Current directory used to resolve relative paths.
Returns: Promise<string[]>
Returns the absolute paths of the files currently cached. Those are the paths of the files before being saved (or after being restored), not while being cached.
export const onPreBuild = async function ({ utils }) {
const files = await utils.cache.list()
console.log('Cached files', files)
}
Type: string
Default: process.cwd()
Current directory used to resolve relative paths.
Type: number
Default: 1
Number of subdirectories to include. 0
means only top-level directories will be included.
FAQs
Utility for caching files in Netlify Build
The npm package @netlify/cache-utils receives a total of 136,655 weekly downloads. As such, @netlify/cache-utils popularity was classified as popular.
We found that @netlify/cache-utils demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.