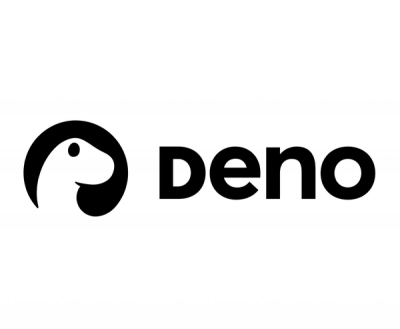
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@nft/hooks
Advanced tools
npm i @nft/hooks
Your application is relying on a web3 wallet. Please refer to your preferred web3 provider for the setup. Here are a few provider tested and supported:
LiteflowProvider
Wrap your app with the LiteflowProvider
component, passing the endpoint
of the API to it (similar to https://api.acme.com/graphql
).
function App() {
return (
<YourWalletProvider>
<LiteflowProvider endpoint="https://api.acme.com/graphql">
<YourRoutes />
</LiteflowProvider>
</YourWalletProvider>
)
}
Every component inside the LiteflowProvider
is now set up to use the Liteflow hooks.
import { useCreateOffer } from '@nft/hooks'
import { OfferType } from '@nft/hooks/dist/graphql'
import { BigNumber } from 'ethers'
import { useSigner } from 'wagmi' // or your favorite web3 wallet
function NFT() {
const { data: signer } = useSigner()
const [authenticate] = useAuthenticate()
const [createOffer] = useCreateOffer(signer)
const handleClick = async () => {
await authenticate(signer)
const offerId = await createOffer({
type: OfferType.Buy,
quantity: BigNumber.from(1),
unitPrice: BigNumber.from(1).mul(BigNumber.from(10).pow(18)), // this value is in base unit of the token. This is equivalent to 1 ETH. Use BigNumber
assetId: 'XXX',
currencyId: 'XXX',
})
alert(`Offer created: ${offerId}`)
}
return <button onClick={handleClick}>Create Offer</button>
}
Want to learn more? Check out the example to learn how to setup Liteflow and continue on reading the documentation.
FAQs
## Installation
The npm package @nft/hooks receives a total of 1 weekly downloads. As such, @nft/hooks popularity was classified as not popular.
We found that @nft/hooks demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.