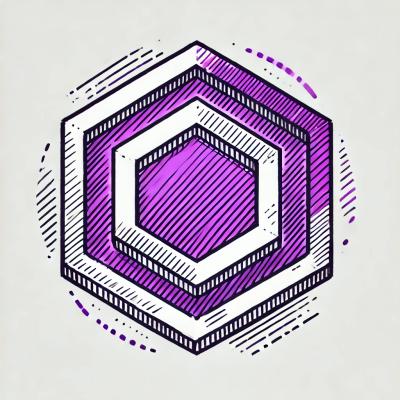
Security News
ESLint is Now Language-Agnostic: Linting JSON, Markdown, and Beyond
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
@ngx-pwa/local-storage
Advanced tools
Efficient local storage module for Angular apps and PWA: simple API based on native localStorage API, but internally stored via the asynchronous IndexedDB API for performance, and wrapped in RxJS observables to be homogeneous with other Angular modules.
Efficient local storage module for Angular apps and Progressive Wep apps (PWA):
localStorage
API and automatic JSON stringify/parse,IndexedDB
API,Observables
,You could also be interested by @ngx-pwa/offline.
The author of this library organizes Angular courses (based in Paris, France, but open to travel). You can find my bio here (in English) and course details here (in French).
For now, Angular does not provide a local storage module, and almost every app needs some local storage. There are 2 native JavaScript APIs available:
The localStorage
API is simple to use but synchronous, so if you use it too often,
your app will soon begin to freeze.
The IndexedDB
API is asynchronous and efficient, but it's a mess to use:
you'll soon be caught by the callback hell, as it does not support Promises yet.
Mozilla has done a very great job with the localForage library :
a simple API based on native localStorage
,
but internally stored via the asynchronous IndexedDB
for performance.
But it's built in ES5 old school way and then it's a mess to include into Angular.
This module is based on the same idea as localForage, but in ES6/ES2015 and additionally wrapped into RxJS Observables to be homogeneous with other Angular modules.
If you already use the previous angular-async-local-storage
package, see the migration guide.
Install the same version as your Angular one via npm:
# For Angular 5 (latest):
npm install @ngx-pwa/local-storage
# For Angular 4 (and TypeScript >= 2.3):
npm install @ngx-pwa/local-storage@4
# For Angular 6 (next):
npm install @ngx-pwa/local-storage@next
Then, for versions 4 & 5 only, include the LocalStorageModule
in your app root module (just once, do NOT re-import it in your submodules). Since version 6, this step is no longer required and LocalStorageModule
is removed.
import { LocalStorageModule } from '@ngx-pwa/local-storage';
@NgModule({
imports: [
BrowserModule,
LocalStorageModule,
...
]
...
})
export class AppModule {}
Now you just have to inject the service where you need it:
import { LocalStorage } from '@ngx-pwa/local-storage';
@Injectable()
export class YourService {
constructor(protected localStorage: LocalStorage) {}
}
The API follows the native localStorage API, except it's asynchronous via RxJS Observables.
let user: User = { firstName: 'Henri', lastName: 'Bergson' };
this.localStorage.setItem('user', user).subscribe(() => {});
You can store any value, without worrying about stringifying.
To delete one item:
this.localStorage.removeItem('user').subscribe(() => {});
To delete all items:
this.localStorage.clear().subscribe(() => {});
this.localStorage.getItem<User>('user').subscribe((user) => {
user.firstName; // should be 'Henri'
});
As any data can be stored, you can type your data.
Not finding an item is not an error, it succeeds but returns null
.
this.localStorage.getItem('notexisting').subscribe((data) => {
data; // null
});
Don't forget it's client-side storage: always check the data, as it could have been forged or deleted. Starting with version 5, you can use a JSON Schema to validate the data.
import { JSONSchema } from '@ngx-pwa/local-storage';
const schema: JSONSchema = {
properties: {
firstName: { type: 'string' },
lastName: { type: 'string' }
},
required: ['firstName', 'lastName']
};
this.localStorage.getItem<User>('user', { schema }).subscribe((user) => {
// Called if data is valid or null
}, (error) => {
// Called if data is invalid
});
Note: last draft of JSON Schema is used (draft 7 at this time), but we don't support all validation features yet. Just follow the interface. Contributions are welcome to add more features.
Note: as the goal is validation, types are enforced: each value MUST have either type
or properties
or items
.
You DO NOT need to unsubscribe: the observable autocompletes (like in the HttpClient
service).
But you DO need to subscribe, even if you don't have something specific to do after writing in local storage (because it's how RxJS Observables work).
Since version 5.2, you can use these methods to auto-subscribe:
this.localStorage.setItemSubscribe('user', user);
this.localStorage.removeItemSubscribe('user');
this.localStorage.clearSubscribe();
Use these methods only if these conditions are fulfilled:
this.localStorage.setItem('color', 'red').subscribe(() => {
// Done
}, () => {
// Error
});
This lib major version is aligned to the major version of Angular. Meaning for Angular 4 you need version 4, for Angular 5 you need version 5, for Angular 6 you need version 6, and so on.
We follow Angular LTS support, meaning we support Angular 4 minimum, until October 2018.
This module supports AoT pre-compiling.
This module supports Universal server-side rendering via a mock storage.
All browsers supporting IndexedDB, ie. all current browsers : Firefox, Chrome, Opera, Safari, Edge, and IE10+.
Local storage is required only for apps, and given that you won't do an app in older browsers, current browsers support is far enough.
Even so, IE9 is supported but use native localStorage as a fallback, so internal operations are synchronous (the public API remains asynchronous-like).
This module is not impacted by IE/Edge missing IndexedDB features.
It also works in tools based on browser engines (like Electron) but not in non-browser tools (like NativeScript, see #11).
Be aware that local storage is limited in browsers when in private / incognito modes. Most browsers will delete the data when the private browsing session ends. It's not a real issue as local storage is useful for apps, and apps should not be in private mode.
In IE / Edge, indexedDB
is null
when in private mode. The lib fallbacks to (synchronous) localStorage
.
In Firefox, indexedDB
API is available in code but throwing error on usage. It's a bug in the browser, this lib can't handle it, see
#26.
Starting with version 5, you can easily add your own storage:
import { LocalDatabase } from '@ngx-pwa/local-storage';
export class MyDatabase implements LocalDatabase {
/* Implement the methods required by the LocalDatabase class */
}
@NgModule({
providers: [{ provide: LocalDatabase, useClass: MyDatabase }]
})
export class AppModule {}
Be sure to be compatible with Universal by checking the current platform before using any browser-specific API.
You can also extend the JSON validator, but if you do so, please contribute so everyone can enjoy.
MIT
FAQs
Efficient local storage module for Angular: simple API based on native localStorage API, but internally stored via the asynchronous IndexedDB API for performance, and wrapped in RxJS observables to be homogeneous with other Angular modules.
The npm package @ngx-pwa/local-storage receives a total of 14,849 weekly downloads. As such, @ngx-pwa/local-storage popularity was classified as popular.
We found that @ngx-pwa/local-storage demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
Security News
Members Hub is conducting large-scale campaigns to artificially boost Discord server metrics, undermining community trust and platform integrity.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.