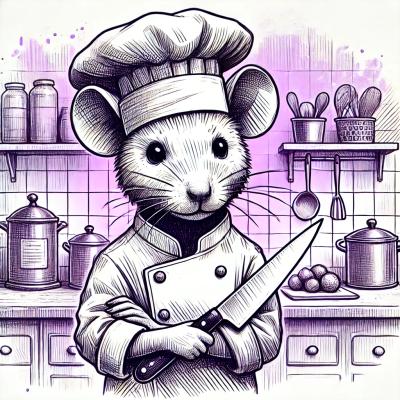
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@nimbusforwork/nimbus-ui
Advanced tools
UI components for React Native and React Native Web, built with Typescript Documentation: https://nimbus-ui.netlify.com/
Import theme and custom Font
import * as Font from 'expo-font'
import { ThemeProvider } from 'styled-components/native'
import { theme, FontFamily } from '@nimbusforwork/nimbus-ui'
...
const initFont = async () => {
await Font.loadAsync({
body_bold: FontFamily.FontInterBold,
body_base: FontFamily.FontInterRegular,
body_medium: FontFamily.FontInterMedium
})
}
render() {
return (
<ThemeProvider theme={theme}>
{!loading && <AppContainer />}
</ThemeProvider>
)
}
...
.babelrc
{
"presets": ["next/babel"],
"plugins": [["react-native-web", { "commonjs": true }]]
}
app.json
{
"name": "with-react-native-web",
"displayName": "with-react-native-web"
}
next.config.js
const path = require('path')
const withTM = require('next-transpile-modules')
const withFonts = require('next-fonts')
const withCSS = require('@zeit/next-css')
const nextConf = {
env: {
API_PATH: process.env.API_PATH,
API_KEY: process.env.API_KEY
},
transpileModules: ['react-native', 'styled-components', 'styled-components/native', '@nimbusforwork/nimbus-ui'],
webpack: config => {
config.module.rules.push({
test: /\.ttf$/,
loader: 'url-loader',
include: path.resolve(__dirname, 'node_modules/react-native-vector-icons')
})
config.module.rules.push({
test: /\.svg$/,
use: ['@svgr/webpack']
})
return {
...config,
resolve: {
...config.resolve,
extensions: [
'.web.ts',
'.web.tsx',
'.ts',
'.tsx',
'.web.js',
'.web.jsx',
'.js',
'.jsx',
...config.resolve.extensions
],
alias: {
...config.resolve.alias,
'react-native$': 'react-native-web'
}
}
}
},
cssModules: true
}
module.exports = withTM(withFonts(withCSS(nextConf)))
Default fontFamily in nimbus-ui theme
theme.fontFamily = 'body'
fonts type
body_base: Inter-Regular
body_bold: Inter-Bold
body_medium: Inter-Medium
Custom fonts
@font-face {
font-family: 'body_base';
src: url('./fonts/Inter-Regular.ttf');
}
@font-face {
font-family: 'body_bold';
src: url('./fonts/Inter-Bold.ttf');
}
@font-face {
font-family: 'body_medium';
src: url('./fonts/Inter-Medium.ttf');
}
FAQs
Nimbus UI Components
We found that @nimbusforwork/nimbus-ui demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.