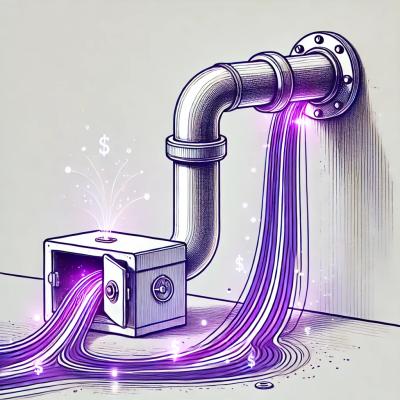
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
@nomicfoundation/ethereumjs-common
Advanced tools
Resources common to all Ethereum implementations
@nomicfoundation/ethereumjs-common is a library that provides common Ethereum constants, parameters, and functions for various Ethereum networks. It is useful for developers working on Ethereum-based projects who need to handle different network configurations and parameters.
Network Configuration
This feature allows you to create a custom network configuration. The code sample demonstrates how to create a custom network configuration for a network with a specific networkId and chainId.
const Common = require('@nomicfoundation/ethereumjs-common').default;
const mainnetCommon = Common.forCustomChain('mainnet', {
name: 'custom-network',
networkId: 1234,
chainId: 1234,
}, 'istanbul');
console.log(mainnetCommon.chainName());
Hardfork Support
This feature allows you to specify and work with different Ethereum hardforks. The code sample shows how to create a Common instance for the mainnet with the Berlin hardfork.
const Common = require('@nomicfoundation/ethereumjs-common').default;
const common = new Common({ chain: 'mainnet', hardfork: 'berlin' });
console.log(common.hardfork());
Chain Parameters
This feature provides access to various chain parameters such as genesis block information. The code sample demonstrates how to retrieve the genesis block parameters for the Ropsten test network.
const Common = require('@nomicfoundation/ethereumjs-common').default;
const common = new Common({ chain: 'ropsten' });
console.log(common.genesis());
web3 is a comprehensive library for interacting with the Ethereum blockchain. It provides functionalities for sending transactions, interacting with smart contracts, and more. While it offers some network configuration capabilities, it is more focused on general blockchain interactions compared to the specialized network configuration focus of @nomicfoundation/ethereumjs-common.
ethers is another popular library for interacting with the Ethereum blockchain. It provides utilities for sending transactions, interacting with smart contracts, and managing wallets. Similar to web3, it includes some network configuration features but is more broadly focused on Ethereum interactions rather than the specific network configuration and parameter management provided by @nomicfoundation/ethereumjs-common.
ethereumjs-tx is a library for creating and signing Ethereum transactions. It is part of the ethereumjs suite of libraries and focuses on transaction creation and signing. While it can be used in conjunction with @nomicfoundation/ethereumjs-common, it does not provide the same level of network configuration and parameter management.
Resources common to all EthereumJS implementations. |
---|
Note: this README
reflects the state of the library from v2.0.0
onwards. See README
from the standalone repository for an introduction on the last preceding release.
To obtain the latest version, simply require the project using npm
:
npm install @ethereumjs/common
import (ESM, TypeScript):
import { Chain, Common, Hardfork } from '@ethereumjs/common'
require (CommonJS, Node.js):
const { Common, Chain, Hardfork } = require('@ethereumjs/common')
All parameters can be accessed through the Common
class, instantiated with an object containing either the chain
(e.g. 'Chain.Mainnet') or the chain
together with a specific hardfork
provided:
// ./examples/common.ts#L1-L7
import { Chain, Common, Hardfork } from '@ethereumjs/common'
// With enums:
const commonWithEnums = new Common({ chain: Chain.Mainnet, hardfork: Hardfork.London })
// (also possible with directly passing in strings:)
const commonWithStrings = new Common({ chain: 'mainnet', hardfork: 'london' })
If no hardfork is provided, the common is initialized with the default hardfork.
Current DEFAULT_HARDFORK
: Hardfork.Shanghai
Here are some simple usage examples:
// ./examples/common.ts#L9-L23
// Instantiate with the chain (and the default hardfork)
let c = new Common({ chain: Chain.Mainnet })
console.log(`The gas price for ecAdd is ${c.param('gasPrices', 'ecAddGas')}`) // 500
// Chain and hardfork provided
c = new Common({ chain: Chain.Mainnet, hardfork: Hardfork.Byzantium })
console.log(`The miner reward under PoW on Byzantium us ${c.param('pow', 'minerReward')}`) // 3000000000000000000
// Get bootstrap nodes for chain/network
console.log('Below are the known bootstrap nodes')
console.log(c.bootstrapNodes()) // Array with current nodes
// Instantiate with an EIP activated
c = new Common({ chain: Chain.Mainnet, eips: [4844] })
console.log(`EIP 4844 is active -- ${c.isActivatedEIP(4844)}`)
With the breaking release round in Summer 2023 we have added hybrid ESM/CJS builds for all our libraries (see section below) and have eliminated many of the caveats which had previously prevented a frictionless browser usage.
It is now easily possible to run a browser build of one of the EthereumJS libraries within a modern browser using the provided ESM build. For a setup example see ./examples/browser.html.
See the API documentation for a full list of functions for accessing specific chain and
depending hardfork parameters. There are also additional helper functions like
paramByBlock (topic, name, blockNumber)
or hardforkIsActiveOnBlock (hardfork, blockNumber)
to ease blockNumber
based access to parameters.
Generated TypeDoc API Documentation
With the breaking releases from Summer 2023 we have started to ship our libraries with both CommonJS (cjs
folder) and ESM builds (esm
folder), see package.json
for the detailed setup.
If you use an ES6-style import
in your code files from the ESM build will be used:
import { EthereumJSClass } from '@ethereumjs/[PACKAGE_NAME]'
If you use Node.js specific require
, the CJS build will be used:
const { EthereumJSClass } = require('@ethereumjs/[PACKAGE_NAME]')
Using ESM will give you additional advantages over CJS beyond browser usage like static code analysis / Tree Shaking which CJS can not provide.
With the breaking releases from Summer 2023 we have removed all Node.js specific Buffer
usages from our libraries and replace these with Uint8Array representations, which are available both in Node.js and the browser (Buffer
is a subclass of Uint8Array
).
We have converted existing Buffer conversion methods to Uint8Array conversion methods in the @ethereumjs/util bytes
module, see the respective README section for guidance.
Starting with v4 the usage of BN.js for big numbers has been removed from the library and replaced with the usage of the native JS BigInt data type (introduced in ES2020
).
Please note that number-related API signatures have changed along with this version update and the minimal build target has been updated to ES2020
.
The Common
class has a public property events
which contains an EventEmitter
. Following events are emitted on which you can react within your code:
Event | Description |
---|---|
hardforkChanged | Emitted when a hardfork change occurs in the Common object |
The chain
can be set in the constructor like this:
const c = new Common({ chain: Chain.Mainnet })
Supported chains:
mainnet
(Chain.Mainnet
)goerli
(Chain.Goerli
)sepolia
(Chain.Sepolia
) (v2.6.1
+)holesky
(Chain.Holesky
) (v4.1.0
+)The following chain-specific parameters are provided:
name
chainId
networkId
consensusType
(e.g. pow
or poa
)consensusAlgorithm
(e.g. ethash
or clique
)consensusConfig
(depends on consensusAlgorithm
, e.g. period
and epoch
for clique
)genesis
block header valueshardforks
block numbersbootstrapNodes
listdnsNetworks
list (EIP-1459-compliant list of DNS networks for peer discovery)To get an overview of the different parameters have a look at one of the chain configurations in the chains.ts
configuration
file, or to the Chain
type in ./src/types.ts.
There are two distinct APIs available for setting up custom(ized) chains.
There is a dedicated Common.custom()
static constructor which allows for an easy instantiation of a Common instance with somewhat adopted chain parameters, with the main use case to adopt on instantiating with a deviating chain ID (you can use this to adopt other chain parameters as well though). Instantiating a custom common instance with its own chain ID and inheriting all other parameters from mainnet
can now be as easily done as:
// ./examples/common.ts#L25-L27
// Instantiate common with custom chainID
const commonWithCustomChainId = Common.custom({ chainId: 1234 })
console.log(`The current chain ID is ${commonWithCustomChainId.chainId}`)
The custom()
method also takes a string as a first input (instead of a dictionary). This can be used in combination with the CustomChain
enum dict which allows for the selection of predefined supported custom chains for an easier Common
setup of these supported chains:
const common = Common.custom(CustomChain.ArbitrumRinkebyTestnet)
The following custom chains are currently supported:
PolygonMainnet
PolygonMumbai
ArbitrumRinkebyTestnet
xDaiChain
OptimisticKovan
OptimisticEthereum
Common
instances created with this simplified custom()
constructor can't be used in all usage contexts (the HF configuration is very likely not matching the actual chain) but can be useful for specific use cases, e.g. for sending a tx with @ethereumjs/tx
to an L2 network (see the Tx
library README for a complete usage example).
If you want to initialize a Common
instance with a single custom chain which is then directly activated
you can pass a dictionary - conforming to the parameter format described above - with your custom chain
values to the constructor using the chain
parameter or the setChain()
method, here is some example:
// ./examples/customChain.ts
import { Common } from '@ethereumjs/common'
import myCustomChain1 from './genesisData/testnet.json'
// Add custom chain config
const common1 = new Common({ chain: myCustomChain1 })
console.log(`Common is instantiated with custom chain parameters - ${common1.chainName()}`)
A second way for custom chain initialization is to use the customChains
constructor option. This
option comes with more flexibility and allows for an arbitrary number of custom chains to be initialized on
a common instance in addition to the already supported ones. It also allows for an activation-independent
initialization, so you can add your chains by adding to the customChains
array and either directly
use the chain
option to activate one of the custom chains passed or activate a build in chain
(e.g. mainnet
) and switch to other chains - including the custom ones - by using Common.setChain()
.
// ./examples/customChains.ts
import { Common } from '@ethereumjs/common'
import myCustomChain1 from './genesisData/testnet.json'
import myCustomChain2 from './genesisData/testnet2.json'
// Add two custom chains, initial mainnet activation
const common1 = new Common({ chain: 'mainnet', customChains: [myCustomChain1, myCustomChain2] })
console.log(`Common is instantiated with mainnet parameters - ${common1.chainName()}`)
common1.setChain('testnet1')
console.log(`Common is set to use testnet parameters - ${common1.chainName()}`)
// Add two custom chains, activate customChain1
const common2 = new Common({
chain: 'testnet2',
customChains: [myCustomChain1, myCustomChain2],
})
console.log(`Common is instantiated with testnet2 parameters - ${common1.chainName()}`)
Starting with v3 custom genesis states should be passed to the Blockchain library directly.
For lots of custom chains (for e.g. devnets and testnets), you might come across a genesis json config which has both config specification for the chain as well as the genesis state specification. You can derive the common from such configuration in the following manner:
// ./examples/fromGeth.ts
import { Common } from '@ethereumjs/common'
import { hexToBytes } from '@ethereumjs/util'
import genesisJson from './genesisData/post-merge.json'
const genesisHash = hexToBytes('0x3b8fb240d288781d4aac94d3fd16809ee413bc99294a085798a589dae51ddd4a')
// Load geth genesis json file into lets say `genesisJson` and optional `chain` and `genesisHash`
const common = Common.fromGethGenesis(genesisJson, { chain: 'customChain', genesisHash })
// If you don't have `genesisHash` while initiating common, you can later configure common (for e.g.
// after calculating it via `blockchain`)
common.setForkHashes(genesisHash)
console.log(`The London forkhash for this custom chain is ${common.forkHash('london')}`)
The hardfork
can be set in constructor like this:
// ./examples/common.ts#L1-L4
import { Chain, Common, Hardfork } from '@ethereumjs/common'
// With enums:
const commonWithEnums = new Common({ chain: Chain.Mainnet, hardfork: Hardfork.London })
There are currently parameter changes by the following past and future hardfork by the library supported:
chainstart
(Hardfork.Chainstart
)homestead
(Hardfork.Homestead
)dao
(Hardfork.Dao
)tangerineWhistle
(Hardfork.TangerineWhistle
)spuriousDragon
(Hardfork.SpuriousDragon
)byzantium
(Hardfork.Byzantium
)constantinople
(Hardfork.Constantinople
)petersburg
(Hardfork.Petersburg
) (aka constantinopleFix
, apply together with constantinople
)istanbul
(Hardfork.Instanbul
)muirGlacier
(Hardfork.MuirGlacier
)berlin
(Hardfork.Berlin
) (since v2.2.0
)london
(Hardfork.London
) (since v2.4.0
)merge
(Hardfork.Merge
) (DEFAULT_HARDFORK
) (since v2.5.0
)shanghai
(Hardfork.Shanghai
) (since v3.1.0
)cancun
(Hardfork.Cancun
) (since v4.0.0
)The next upcoming HF Hardfork.Prague
is currently not yet supported by this library.
For hardfork-specific parameter access with the param()
and paramByBlock()
functions
you can use the following topics
:
gasConfig
gasPrices
vm
pow
sharding
See one of the hardfork configurations in the hardforks.ts
file
for an overview. For consistency, the chain start (chainstart
) is considered an own
hardfork.
The hardfork configurations above chainstart
only contain the deltas from chainstart
and
shouldn't be accessed directly until you have a specific reason for it.
Starting with the v2.0.0
release of the library, EIPs are now native citizens within the library
and can be activated like this:
const c = new Common({ chain: Chain.Mainnet, eips: [4844] })
The following EIPs are currently supported:
outdated
)outdated
)outdated
)experimental
)You can use common.bootstrapNodes()
function to get nodes for a specific chain/network.
See our organizational documentation for an introduction to EthereumJS
as well as information on current standards and best practices. If you want to join for work or carry out improvements on the libraries, please review our contribution guidelines first.
FAQs
Resources common to all Ethereum implementations
The npm package @nomicfoundation/ethereumjs-common receives a total of 133,077 weekly downloads. As such, @nomicfoundation/ethereumjs-common popularity was classified as popular.
We found that @nomicfoundation/ethereumjs-common demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.