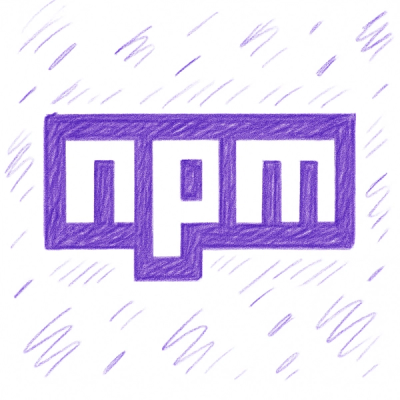
Security News
npm Adopts OIDC for Trusted Publishing in CI/CD Workflows
npm now supports Trusted Publishing with OIDC, enabling secure package publishing directly from CI/CD workflows without relying on long-lived tokens.
@notifee/react-native
Advanced tools
Notifee - a feature rich notifications library for React Native.
@notifee/react-native is a comprehensive library for managing local notifications in React Native applications. It provides a wide range of features for creating, displaying, and handling notifications, including support for custom sounds, actions, and scheduling.
Displaying a Simple Notification
This feature allows you to display a simple notification with a title and body content.
import notifee from '@notifee/react-native';
async function displayNotification() {
await notifee.displayNotification({
title: 'Notification Title',
body: 'Main body content of the notification',
});
}
Scheduling a Notification
This feature allows you to schedule a notification to be displayed at a specific time in the future.
import notifee, { TimestampTrigger, TriggerType } from '@notifee/react-native';
async function scheduleNotification() {
const date = new Date(Date.now());
date.setMinutes(date.getMinutes() + 5);
const trigger = {
type: TriggerType.TIMESTAMP,
timestamp: date.getTime(),
};
await notifee.createTriggerNotification(
{
title: 'Scheduled Notification',
body: 'This notification was scheduled to appear 5 minutes later',
},
trigger
);
}
Custom Notification Sounds
This feature allows you to set a custom sound for your notifications.
import notifee from '@notifee/react-native';
async function customSoundNotification() {
await notifee.displayNotification({
title: 'Custom Sound Notification',
body: 'This notification has a custom sound',
android: {
sound: 'custom_sound',
},
});
}
Notification Actions
This feature allows you to add actions to your notifications, enabling users to interact with them directly.
import notifee, { AndroidAction } from '@notifee/react-native';
async function notificationWithActions() {
await notifee.displayNotification({
title: 'Notification with Actions',
body: 'This notification has actions',
android: {
actions: [
{
title: 'Action 1',
pressAction: { id: 'action1' },
},
{
title: 'Action 2',
pressAction: { id: 'action2' },
},
],
},
});
}
react-native-push-notification is another popular library for handling local and remote notifications in React Native. It offers similar functionalities such as scheduling notifications, custom sounds, and actions. However, @notifee/react-native provides a more modern API and better support for advanced features.
react-native-notifications is a library focused on providing a unified API for handling notifications across iOS and Android. It offers features like scheduling, custom sounds, and actions. Compared to @notifee/react-native, it has a more straightforward API but may lack some of the advanced customization options.
expo-notifications is part of the Expo ecosystem and provides a simple way to handle notifications in Expo and React Native projects. It supports scheduling, custom sounds, and actions. While it is easy to use and integrates well with Expo, it may not offer the same level of customization and advanced features as @notifee/react-native.
A feature rich Android & iOS notifications library for React Native.
yarn add @notifee/react-native
The APIs for Android allow for creating rich, styled and highly interactive notifications. Below you'll find guides that cover the supported Android features.
Topic | |
---|---|
Appearance | Change the appearance of a notification; icons, colors, visibility etc. |
Behaviour | Customize how a notification behaves when it is delivered to a device; sound, vibration, lights etc. |
Channels & Groups | Organize your notifications into channels & groups to allow users to control how notifications are handled on their device |
Foreground Service | Long running background tasks can take advantage of a Android Foreground Services to display an on-going, prominent notification. |
Grouping & Sorting | Group and sort related notifications in a single notification pane. |
Interaction | Allow users to interact with your application directly from the notification with actions. |
Progress Indicators | Show users a progress indicator of an on-going background task, and learn how to keep it updated. |
Styles | Style notifications to show richer content, such as expandable images/text, or message conversations. |
Timers | Display counting timers on your notification, useful for on-going tasks such as a phone call, or event time remaining. |
Below you'll find guides that cover the supported iOS features.
Topic | |
---|---|
Appearance | Change now the notification is displayed to your users. |
Behaviour | Control how notifications behave when they are displayed to a device; sound, crtitial alerts etc. |
Categories | Create & assign categories to notifications. |
Interaction | Handle user interaction with your notifications. |
Permissions | Request permission from your application users to display notifications. |
To run jest tests after integrating this module, you will need to mock out the native parts of Notifee or you will get an error that looks like:
● Test suite failed to run
Notifee native module not found.
59 | this._nativeModule = NativeModules[this._moduleConfig.nativeModuleName];
60 | if (this._nativeModule == null) {
> 61 | throw new Error('Notifee native module not found.');
| ^
62 | }
63 |
64 | return this._nativeModule;
Add this to a setup file in your project e.g. jest.setup.js
:
If you don't already have a Jest setup file configured, please add the following to your Jest configuration file and create the new jest.setup.js file in project root:
setupFiles: ['<rootDir>/jest.setup.js'],
You can then add the following line to that setup file to mock notifee
:
jest.mock('@notifee/react-native', () => require('@notifee/react-native/jest-mock'))
You will also need to add @notifee
to transformIgnorePatterns
in your config file (jest.config.js
):
transformIgnorePatterns: [
'node_modules/(?!(jest-)?react-native|@react-native|@notifee)'
]
To utilise Detox's functionality to mock a local notification and trigger notifee's event handlers, you will need a payload with a key __notifee_notification
:
{
title: 'test',
body: 'Body',
payload: {
__notifee_notification: {
ios: {
foregroundPresentationOptions: {
banner: true,
list: true,
},
},
data: {}
},
},
}
The important part is to make sure you have a __notifee_notification
object under payload
with the default properties.
Built and maintained with 💛 by Invertase.
FAQs
Notifee - a feature rich notifications library for React Native.
The npm package @notifee/react-native receives a total of 170,382 weekly downloads. As such, @notifee/react-native popularity was classified as popular.
We found that @notifee/react-native demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
npm now supports Trusted Publishing with OIDC, enabling secure package publishing directly from CI/CD workflows without relying on long-lived tokens.
Research
/Security News
A RubyGems malware campaign used 60 malicious packages posing as automation tools to steal credentials from social media and marketing tool users.
Security News
The CNA Scorecard ranks CVE issuers by data completeness, revealing major gaps in patch info and software identifiers across thousands of vulnerabilities.