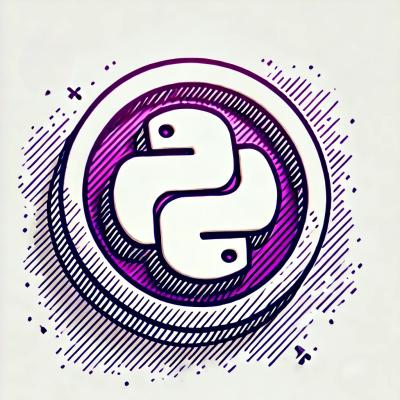
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
@nouance/payload-better-fields-plugin
Advanced tools
A Payload plugin that aims to provide improved fields for the admin panel
This plugin aims to provide you with very specific and improved fields for the admin panel.
We've tried to keep styling as consistent as possible with the existing admin UI, however if there are any issues please report them!
Every field will come with its own usage instructions and structure. These are subject to change!
yarn add @nouance/payload-better-fields-plugin
# OR
npm i @nouance/payload-better-fields-plugin
We've tried to re-use the internal components of Payload as much as possible. Where we can't we will re-use the CSS variables used in the core theme to ensure as much compatibility with user customisations.
If you want to further customise the CSS of these components, every component comes with a unique class wrapper in the format of bf<field-name>Wrapper
, eg bfPatternFieldWrapper
, to help you target these inputs consistently.
import { CollectionConfig } from 'payload/types'
import { SlugField } from '@nouance/payload-better-fields-plugin'
const Examples: CollectionConfig = {
slug: 'examples',
admin: {
useAsTitle: 'title',
},
fields: [
{
name: 'title',
type: 'text',
},
...SlugField(['title'], undefined, {
admin: {
position: 'sidebar',
},
}),
],
}
The SlugField
accepts the following parameters
fieldToUse
- string[]
defaults to ['title']
slugifyOptions
- Options to be passed to the slugify function
@default
{ lower: true, remove: /[*+~.()'"!?#\.,:@]/g }
slugOverrides
- TextField
Slug field overrides, use this to rename the machine name or label of the field
enableEditSlug
- boolean
defaults to true
| Enable or disable the checkbox field
editSlugOverrides
- CheckboxField
| Checkbox field overrides
import { CollectionConfig } from 'payload/types'
import { ComboField } from '@nouance/payload-better-fields-plugin'
const Examples: CollectionConfig = {
slug: 'examples',
admin: {
useAsTitle: 'fullName',
},
fields: [
{
type: 'row',
fields: [
{
name: 'firstName',
type: 'text',
},
{
name: 'lastName',
type: 'text',
},
],
},
...ComboField(['firstName', 'lastName'], { name: 'fullName' }),
],
}
The ComboField
accepts the following parameters
fieldToUse
- string[]
required
overrides
- TextField
required for name attribute
options
separator
- string
defaults to ' '
initial
- string
The starting string value before all fields are concatenated
callback
- (value: string) => string
You can apply a callback to modify each field value if you want to preprocess them
source | react-number-format NumericFormat
import { CollectionConfig } from 'payload/types'
import { NumberField } from '@nouance/payload-better-fields-plugin'
const Examples: CollectionConfig = {
slug: 'examples',
admin: {
useAsTitle: 'fullName',
},
fields: [
{
name: 'title',
type: 'text',
},
...NumberField(
{
prefix: '$ ',
thousandSeparator: ',',
decimalScale: 2,
fixedDecimalScale: true,
},
{
name: 'price',
required: true,
},
),
],
}
The NumberField
accepts the following parameters
format
- NumericFormatProps
required, accepts props for NumericFormat
callback
- you can override the internal callback on the value, the value
will be a string so you need to handle the conversion to an int or float yourself via parseFloat// example
callback: (value) => parseFloat(value) + 20,
overrides
- NumberField
required for name attribute
source | react-number-format PatternFormat
import { CollectionConfig } from 'payload/types'
import { PatternField } from '@nouance/payload-better-fields-plugin'
const Examples: CollectionConfig = {
slug: 'examples',
admin: {
useAsTitle: 'fullName',
},
fields: [
{
name: 'title',
type: 'text',
},
...PatternField(
{
format: '+1 (###) #### ###',
prefix: '% ',
allowEmptyFormatting: true,
mask: '_',
},
{
name: 'telephone',
type: 'text',
required: false,
admin: {
placeholder: '% 20',
},
},
),
],
}
The PatternField
accepts the following parameters
format
- PatternFormatProps
required, accepts props for PatternFormat
format
required, input for the pattern to be applied
callback
- you can override the internal callback on the value, the value
will be a string so you need to handle the conversion to an int or float yourself via parseFloat
// example
callback: (value) => value + 'ID',
overrides
- TextField
required for name attribute
We recommend using a text field in Payload.
import { CollectionConfig } from 'payload/types'
import { RangeField } from '@nouance/payload-better-fields-plugin'
const Examples: CollectionConfig = {
slug: 'examples',
admin: {
useAsTitle: 'title',
},
fields: [
{
name: 'title',
type: 'text',
},
...RangeField(
{ min: 5, max: 200, step: 5 },
{
name: 'groups',
},
),
],
}
export default Examples
The RangeField
accepts the following parameters
config
- required
min
- number
defaults to 1
max
- number
defaults to 100
step
- number
defaults to 1
showPreview
- boolean
defaults to false, shows a preview box of the underlying selected value, n/a
for null
markers
- NumberMarker[]
array of markers to be visually set, accepts an optional label
{ value: number, label?: string}[]
overrides
- NumberField
required for name attribute
import { CollectionConfig } from 'payload/types'
import { ColourTextField } from '@nouance/payload-better-fields-plugin'
const Examples: CollectionConfig = {
slug: 'examples',
admin: {
useAsTitle: 'title',
},
fields: [
{
name: 'title',
type: 'text',
},
...ColourTextField({
name: 'colour',
}),
],
}
export default Examples
The ColourTextField
accepts the following parameters
overrides
- TextField
required for name attributesource | react-phone-number-input
import { CollectionConfig } from 'payload/types'
import { TelephoneField } from '@nouance/payload-better-fields-plugin'
const Examples: CollectionConfig = {
slug: 'examples',
admin: {
useAsTitle: 'title',
},
fields: [
{
name: 'title',
type: 'text',
},
...TelephoneField({
name: 'telephone',
admin: {
placeholder: '+1 2133 734 253',
},
}),
],
}
export default Examples
The TelephoneField
accepts the following parameters
overrides
- TextField
required for name
attribute
config
- Config
optional, allows for overriding attributes in the phone field
international
defaults to true
| Forces international formatting
defaultCountry
accepts a 2-letter country code eg. 'US'
| If defaultCountry is specified then the phone number can be input both in "international" format and "national" format
country
accepts a 2-letter country code eg. 'US'
| If country is specified then the phone number can only be input in "national" (not "international") format
initialValueFormat
If an initial value is passed, and initialValueFormat is "national", then the initial value is formatted in national format
withCountryCallingCode
If country is specified and international property is true then the phone number can only be input in "international" format for that country
countryCallingCodeEditable
smartCaret
When the user attempts to insert a digit somewhere in the middle of a phone number, the caret position is moved right before the next available digit skipping any punctuation in between
useNationalFormatForDefaultCountryValue
When defaultCountry is defined and the initial value corresponds to defaultCountry, then the value will be formatted as a national phone number by default
countrySelectProps
unicodeFlags
defaults to false
| Set to true
to render Unicode flag icons instead of SVG imagesFor development purposes, there is a full working example of how this plugin might be used in the dev directory of this repo.
git clone git@github.com:NouanceLabs/payload-better-fields-plugin.git \
cd payload-better-fields-plugin && yarn \
cd demo && yarn \
cp .env.example .env \
vim .env \ # add your payload details
yarn dev
FAQs
A Payload plugin that aims to provide improved fields for the admin panel
The npm package @nouance/payload-better-fields-plugin receives a total of 1,830 weekly downloads. As such, @nouance/payload-better-fields-plugin popularity was classified as popular.
We found that @nouance/payload-better-fields-plugin demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.