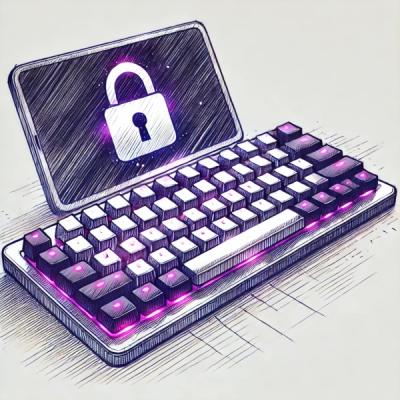
Research
Security News
Malicious npm Package Typosquats react-login-page to Deploy Keylogger
Socket researchers unpack a typosquatting package with malicious code that logs keystrokes and exfiltrates sensitive data to a remote server.
@nuxtjs/axios
Advanced tools
Package description
@nuxtjs/axios is a Nuxt.js module that integrates the Axios HTTP client with Nuxt.js applications. It simplifies making HTTP requests in a Nuxt.js project by providing a consistent API and additional features tailored for Nuxt.js.
Making GET Requests
This feature allows you to make GET requests to fetch data from an API endpoint. The example demonstrates how to use the $axios.$get method within the asyncData lifecycle hook to fetch data before rendering the page.
async asyncData({ $axios }) {
const data = await $axios.$get('https://api.example.com/data');
return { data };
}
Making POST Requests
This feature allows you to make POST requests to send data to an API endpoint. The example shows how to use the $axios.$post method to submit form data to a server.
async submitForm({ $axios }, formData) {
const response = await $axios.$post('https://api.example.com/submit', formData);
return response;
}
Setting Base URL
This feature allows you to set a base URL for all Axios requests. The example demonstrates how to configure the base URL in the Nuxt.js configuration file.
export default {
axios: {
baseURL: 'https://api.example.com'
}
}
Handling Errors
This feature allows you to handle errors that occur during HTTP requests. The example shows how to use a try-catch block to catch errors and handle them appropriately within the asyncData lifecycle hook.
async asyncData({ $axios, error }) {
try {
const data = await $axios.$get('https://api.example.com/data');
return { data };
} catch (e) {
error({ statusCode: 500, message: 'Internal Server Error' });
}
}
Axios is a promise-based HTTP client for the browser and Node.js. It provides a simple API for making HTTP requests and is the core library that @nuxtjs/axios is built upon. While @nuxtjs/axios is tailored for Nuxt.js, axios can be used in any JavaScript environment.
Vue-resource is an HTTP client for Vue.js applications. It provides services for making web requests and handling responses using a simple and easy-to-understand API. Compared to @nuxtjs/axios, vue-resource is more tightly integrated with Vue.js but lacks some of the advanced features and flexibility of axios.
Fetch is a native JavaScript API for making HTTP requests. It is built into modern browsers and provides a more low-level approach compared to axios. While fetch is more lightweight and does not require additional dependencies, it lacks some of the convenience features provided by axios, such as request and response interceptors.
Readme
Secure and Easy Axios integration with Nuxt.js.
setToken
function to $axios
so we can easily and globally set authentication tokenswithCredentials
when requesting to base URLInstall with npm:
>_ npm install @nuxtjs/axios
Install with yarn:
>_ yarn add @nuxtjs/axios
nuxt.config.js
{
modules: [
'@nuxtjs/axios',
],
axios: {
// proxyHeaders: false
}
}
asyncData
async asyncData({ app }) {
const ip = await app.$axios.$get('http://icanhazip.com')
return { ip }
}
methods
/created
/mounted
/etc
methods: {
async fetchSomething() {
const ip = await this.$axios.$get('http://icanhazip.com')
this.ip = ip
}
}
nuxtServerInit
async nuxtServerInit ({ commit }, { app }) {
const ip = await app.$axios.$get('http://icanhazip.com')
commit('SET_IP', ip)
}
(Needs Nuxt >= 1.0.0-RC8)
// In store
{
actions: {
async getIP ({ commit }) {
const ip = await this.$axios.$get('http://icanhazip.com')
commit('SET_IP', ip)
}
}
}
You can pass options using module options or axios
section in nuxt.config.js
baseURL
http://[HOST]:[PORT]/api
Base URL is required for requests in server-side & SSR and prepended to all requests with relative path.
You can also use environment variable API_URL
which overrides baseURL
.
browserBaseURL
/api
Base URL which is used in client side prepended to all requests with relative path.
You can also use environment variable API_URL_BROWSER
which overrides browserBaseURL
.
browserBaseURL
is not provided it defaults to baseURL
value.
browserbaseURL
are equal to nuxt server, it defaults to relative part of baseURL
.
So if your nuxt application is being accessed under a different domain, requests go to same origin and prevents Cross-Origin problems.credentials
true
Adds an interceptor to automatically set withCredentials
config of axios when requesting to baseUrl
which allows passing authentication headers to backend.
debug
false
Adds interceptors to log all responses and requests
proxyHeaders
true
In SSR context, sets client request header as axios default request headers. This is useful for making requests which need cookie based auth on server side. Also helps making consistent requests in both SSR and Client Side code.
NOTE: If directing requests at a url protected by CloudFlare's CDN you should set this to false to prevent CloudFlare from mistakenly detecting a reverse proxy loop and returning a 403 error.
proxyHeadersIgnore
['host', 'accept']
Only efficient when proxyHeaders
is set to true. Removes unwanted request headers to the API backend in SSR.
redirectError
{}
This option is a map from specific error codes to page which they should be redirect.
For example if you want redirecting all 401
errors to /login
use:
axios: {
redirectError: {
401: '/login'
}
}
requestInterceptor
null
Function for manipulating axios requests. Useful for setting custom headers, for example based on the store state. The second argument is the nuxt context.
requestInterceptor: (config, { store }) => {
if (store.state.token) {
config.headers.common['Authorization'] = store.state.token
}
return config
}
responseInterceptor
null
responseInterceptor: (response, ctx) => {
return response
}
Function for manipulating axios responses.
init
null
Function init(axios, ctx)
to do additional things with axios. Example:
axios: {
init(axios, ctx) {
axios.defaults.xsrfHeaderName = 'X-CSRF-TOKEN'
}
}
disableDefaultErrorHandler
false
If you want to disable the default error handler for some reason, you can do it so
by setting the option disableDefaultErrorHandler
to true.
errorHandler
Function for custom global error handler. This example uses nuxt default error page.
If you define a custom error handler, the default error handler provided by this package will be overridden.
axios: {
errorHandler (errorReason, { error }) {
error('Request Error: ' + errorReason)
}
},
Axios plugin also supports fetch style requests with $
prefixed methods:
// Normal usage with axios
let data = (await $axios.get('...')).data
// Fetch Style
let data = await $axios.$get('...')
setHeader(name, value, scopes='common')
Axios instance has a helper to easily set any header.
Parameters:
common
meaning all types of requestsget
, post
, delete
, ...// Adds header: `Authorization: 123` to all requests
this.$axios.setHeader('Authorization', '123')
// Overrides `Authorization` header with new value
this.$axios.setHeader('Authorization', '456')
// Adds header: `Content-Type: application/x-www-form-urlencoded` to only post requests
this.$axios.setHeader('Content-Type', 'application/x-www-form-urlencoded', ['post'])
// Removes default Content-Type header from `post` scope
this.$axios.setHeader('Content-Type', false, ['post'])
setToken(token, type, scopes='common')
Axios instance has an additional helper to easily set global authentication header.
Parameters:
Bearer
).common
meaning all types of requestsget
, post
, delete
, ...// Adds header: `Authorization: 123` to all requests
this.$axios.setToken('123')
// Overrides `Authorization` header with new value
this.$axios.setToken('456')
// Adds header: `Authorization: Bearer 123` to all requests
this.$axios.setToken('123', 'Bearer')
// Adds header: `Authorization: Bearer 123` to only post and delete requests
this.$axios.setToken('123', 'Bearer', ['post', 'delete'])
// Removes default Authorization header from `common` scope (all requests)
this.$axios.setToken(false)
Please notice that, API_URL
is saved into bundle on build, CANNOT be changed
on runtime! You may use proxy module for dynamically route api requests to different backend on test/staging/production.
Example: (nuxt.config.js
)
{
modules: [
'@nuxtjs/axios',
'@nuxtjs/proxy'
],
proxy: [
['/api', { target: process.env.PROXY_API_URL || 'http://www.mocky.io', pathRewrite: { '^/api': '/v2' } }]
]
}
Start Nuxt
[AXIOS] Base URL: http://localhost:3000/api | Browser: /api
[HPM] Proxy created: /api -> http://www.mocky.io
[HPM] Proxy rewrite rule created: "^/api" ~> "/v2"
Now you can make requests to backend: (Works fine in both SSR and Browser)
async asyncData({ app }) {
// Magically makes request to http://www.mocky.io/v2/59388bb4120000dc00a672e2
const nuxt = await app.$axios.$get('59388bb4120000dc00a672e2')
return {
nuxt // -> { nuxt: 'Works!' }
}
}
Details
'@nuxtjs/axios'
http://[host]:[port]/api
which is http://localhost:3000/api
'/api': 'http://www.mocky.io/v2'
/api
to http://www.mocky.io/v2
pathRewrite
to remove /api
from starting of requests and change it to /v2
Copyright (c) 2017 Nuxt Community
FAQs
Unknown package
The npm package @nuxtjs/axios receives a total of 103,873 weekly downloads. As such, @nuxtjs/axios popularity was classified as popular.
We found that @nuxtjs/axios demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers unpack a typosquatting package with malicious code that logs keystrokes and exfiltrates sensitive data to a remote server.
Security News
The JavaScript community has launched the e18e initiative to improve ecosystem performance by cleaning up dependency trees, speeding up critical parts of the ecosystem, and documenting lighter alternatives to established tools.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.