@obolnetwork/obol-sdk
Advanced tools
Comparing version 1.0.4 to 1.0.5
@@ -21,6 +21,6 @@ export declare const CONFLICT_ERROR_MSG = "Conflict"; | ||
export declare const dkg_algorithm = "default"; | ||
export declare const config_version = "v1.5.0"; | ||
export declare const SDK_VERSION = "1.0.0"; | ||
export declare const config_version = "v1.7.0"; | ||
export declare const SDK_VERSION = "1.0.3"; | ||
export declare const DEFAULT_BASE_URL = "https://api.obol.tech"; | ||
export declare const DEFAULT_CHAIN_ID = 5; | ||
//# sourceMappingURL=constants.d.ts.map |
@@ -1,2 +0,2 @@ | ||
export declare const clusterLockV1X5: { | ||
export declare const clusterLockV1X7: { | ||
cluster_definition: { | ||
@@ -31,5 +31,21 @@ name: string; | ||
public_shares: string[]; | ||
deposit_data: { | ||
pubkey: string; | ||
withdrawal_credentials: string; | ||
amount: string; | ||
signature: string; | ||
}; | ||
builder_registration: { | ||
message: { | ||
fee_recipient: string; | ||
gas_limit: number; | ||
timestamp: number; | ||
pubkey: string; | ||
}; | ||
signature: string; | ||
}; | ||
}[]; | ||
signature_aggregate: string; | ||
lock_hash: string; | ||
node_signatures: string[]; | ||
}; | ||
@@ -36,0 +52,0 @@ export declare const clusterConfig: { |
@@ -11,3 +11,3 @@ import { ContainerType, ByteVectorType, UintNumberType, ListCompositeType, ByteListType } from '@chainsafe/ssz'; | ||
export declare const clusterConfigOrDefinitionHash: (cluster: ClusterDefintion, configOnly: boolean) => string; | ||
export declare const hashClusterDefinitionV1X5: (cluster: any, configOnly: boolean) => ValueOfFields<DefinitionFieldsV1X5>; | ||
export declare const hashClusterDefinitionV1X7: (cluster: ClusterDefintion, configOnly: boolean) => ValueOfFields<DefinitionFieldsV1X7>; | ||
/** | ||
@@ -43,3 +43,3 @@ * Converts a string to a Uint8Array | ||
}>; | ||
declare type DefinitionFieldsV1X5 = { | ||
declare type DefinitionFieldsV1X7 = { | ||
uuid: ByteListType; | ||
@@ -58,3 +58,3 @@ name: ByteListType; | ||
}; | ||
export declare type DefinitionContainerTypeV1X5 = ContainerType<DefinitionFieldsV1X5>; | ||
export declare type DefinitionContainerTypeV1X7 = ContainerType<DefinitionFieldsV1X7>; | ||
/** | ||
@@ -65,4 +65,4 @@ * Returns the containerized cluster definition | ||
*/ | ||
export declare const clusterDefinitionContainerTypeV1X5: (configOnly: boolean) => DefinitionContainerTypeV1X5; | ||
export declare const clusterDefinitionContainerTypeV1X7: (configOnly: boolean) => DefinitionContainerTypeV1X7; | ||
export {}; | ||
//# sourceMappingURL=hash.d.ts.map |
import { Signer } from 'ethers'; | ||
import { Base } from './base'; | ||
import { ClusterLock, ClusterPayload } from './types'; | ||
export * from "./types"; | ||
/** | ||
* Obol sdk Client can be used for creating, managing and activating distributed validators. | ||
*/ | ||
export declare class Client extends Base { | ||
private signer; | ||
/** | ||
* @param config | ||
* @param signer ethersJS Signer | ||
* @returns Obol-SDK Client instance | ||
* | ||
* An example of how to instantiate obol-sdk Client: | ||
* [obolClient](https://github.com/ObolNetwork/obol-sdk-examples/blob/main/TS-Example/index.ts#L29) | ||
*/ | ||
constructor(config: { | ||
@@ -11,12 +23,21 @@ baseUrl?: string | undefined; | ||
/** | ||
* @param cluster The new unique cluster | ||
* @returns Invite Link with config_hash | ||
*/ | ||
* Creates a cluster definition which contains cluster configuration. | ||
* @param {ClusterPayload} newCluster - The new unique cluster. | ||
* @returns {Promise<string>} config_hash. | ||
* @throws On duplicate entries, missing or wrong cluster keys. | ||
* | ||
* An example of how to use createClusterDefinition: | ||
* [createObolCluster](https://github.com/ObolNetwork/obol-sdk-examples/blob/main/TS-Example/index.ts#L45) | ||
*/ | ||
createClusterDefinition(newCluster: ClusterPayload): Promise<string>; | ||
/** | ||
* @param configHash The config hash of the requested cluster | ||
* @returns The matched cluster details (lock) from DB | ||
*/ | ||
* @returns {Promise<ClusterLock>} The matched cluster details (lock) from DB | ||
* @throws On not found cluster definition or lock. | ||
* | ||
* An example of how to use getClusterLock: | ||
* [getObolClusterLock](https://github.com/ObolNetwork/obol-sdk-examples/blob/main/TS-Example/index.ts#L61) | ||
*/ | ||
getClusterLock(configHash: string): Promise<ClusterLock>; | ||
} | ||
//# sourceMappingURL=index.d.ts.map |
@@ -1,45 +0,137 @@ | ||
declare type ClusterOperator = { | ||
/** | ||
* Cluster Node Operator | ||
*/ | ||
export declare type ClusterOperator = { | ||
/** The operator address. */ | ||
address: string; | ||
/** The operator ethereum node record. */ | ||
enr?: string; | ||
/** The cluster fork_version. */ | ||
fork_version?: string; | ||
/** The cluster version. */ | ||
version?: string; | ||
/** The operator enr signature. */ | ||
enr_signature?: string; | ||
/** The operator configuration signature. */ | ||
config_signature?: string; | ||
}; | ||
declare type ClusterCreator = { | ||
/** | ||
* Cluster Creator | ||
*/ | ||
export declare type ClusterCreator = { | ||
/** The creator address. */ | ||
address: string; | ||
/** The cluster configuration signature. */ | ||
config_signature?: string; | ||
}; | ||
declare type ClusterValidator = { | ||
/** | ||
* Cluster Validator | ||
*/ | ||
export declare type ClusterValidator = { | ||
/** The validator fee recipient address. */ | ||
fee_recipient_address: string; | ||
/** The validator reward address. */ | ||
withdrawal_address: string; | ||
}; | ||
/** | ||
* Cluster Required Configuration | ||
*/ | ||
export interface ClusterPayload { | ||
/** The cluster name. */ | ||
name: string; | ||
/** The cluster nodes operators addresses. */ | ||
operators: ClusterOperator[]; | ||
/** The clusters validators information. */ | ||
validators: ClusterValidator[]; | ||
} | ||
/** | ||
* Cluster Definition | ||
*/ | ||
export interface ClusterDefintion extends ClusterPayload { | ||
/** The creator of the cluster. */ | ||
creator: ClusterCreator; | ||
/** The cluster configuration version. */ | ||
version: string; | ||
/** The cluster dkg algorithm. */ | ||
dkg_algorithm: string; | ||
/** The cluster fork version. */ | ||
fork_version: string; | ||
/** The cluster uuid. */ | ||
uuid: string; | ||
/** The cluster creation timestamp. */ | ||
timestamp: string; | ||
/** The cluster configuration hash. */ | ||
config_hash: string; | ||
/** The distributed validator threshold. */ | ||
threshold: number; | ||
/** The number of distributed validators in the cluster. */ | ||
num_validators: number; | ||
/** The hash of the cluster definition. */ | ||
definition_hash?: string; | ||
} | ||
declare type DistributedValidator = { | ||
/** | ||
* Unsigned DV Builder Registration Message | ||
*/ | ||
export declare type BuilderRegistrationMessage = { | ||
/** The DV fee recipient. */ | ||
fee_recipient: string; | ||
/** Default is 30000000. */ | ||
gas_limit: number; | ||
/** Timestamp when generating cluster lock file. */ | ||
timestamp: number; | ||
/** The public key of the DV. */ | ||
pubkey: string; | ||
}; | ||
/** | ||
* Pre-generated Signed Validator Builder Registration | ||
*/ | ||
export declare type BuilderRegistration = { | ||
/** Builder registration message. */ | ||
message: BuilderRegistrationMessage; | ||
/** BLS signature of the builder registration message. */ | ||
signature: string; | ||
}; | ||
/** | ||
* Deposit Data | ||
*/ | ||
export declare type DepositData = { | ||
/** The public key of the distributed validator. */ | ||
pubkey: string; | ||
/** The 0x01 withdrawal address of the DV. */ | ||
withdrawal_credentials: string; | ||
/** 32 ethers. */ | ||
amount: string; | ||
/** A checksum for DepositData fields . */ | ||
deposit_data_root: string; | ||
/** BLS signature of the deposit message. */ | ||
signature: string; | ||
}; | ||
/** | ||
* Distributed Validator | ||
*/ | ||
export declare type DistributedValidator = { | ||
/** The public key of the distributed validator. */ | ||
distributed_public_key: string; | ||
/** The public key of the node distributed validator share. */ | ||
public_shares: string[]; | ||
/** The required deposit data for activating the DV. */ | ||
deposit_data: Partial<DepositData>; | ||
/** pre-generated signed validator builder registration to be sent to builder network. */ | ||
builder_registration: BuilderRegistration; | ||
}; | ||
/** | ||
* Cluster Lock (Cluster Details after DKG is complete) | ||
*/ | ||
export interface ClusterLock { | ||
/** The cluster definition. */ | ||
cluster_definition: ClusterDefintion; | ||
/** The cluster distributed validators. */ | ||
distributed_validators: DistributedValidator[]; | ||
/** The cluster bls signature aggregate. */ | ||
signature_aggregate: string; | ||
/** The hash of the cluster lock. */ | ||
lock_hash: string; | ||
/** Node Signature for the lock hash by the node secp256k1 key. */ | ||
node_signatures: string[]; | ||
} | ||
export {}; | ||
//# sourceMappingURL=types.d.ts.map |
{ | ||
"name": "@obolnetwork/obol-sdk", | ||
"version": "1.0.4", | ||
"version": "1.0.5", | ||
"description": "A package for creating Distributed Validators using the Obol API.", | ||
@@ -20,2 +20,3 @@ "main": "dist/index.js", | ||
"ds:build": "rollup -c", | ||
"generate-typedoc": "typedoc", | ||
"ds:release:major": "npm version $(semver $npm_package_version -i major) && npm publish --tag latest", | ||
@@ -57,4 +58,5 @@ "ds:release:minor": "npm version $(semver $npm_package_version -i minor) && npm publish --tag latest", | ||
"tsup": "^6.7.0", | ||
"typedoc": "^0.24.8", | ||
"typescript": "^5.1.3" | ||
} | ||
} |
@@ -9,2 +9,2 @@ 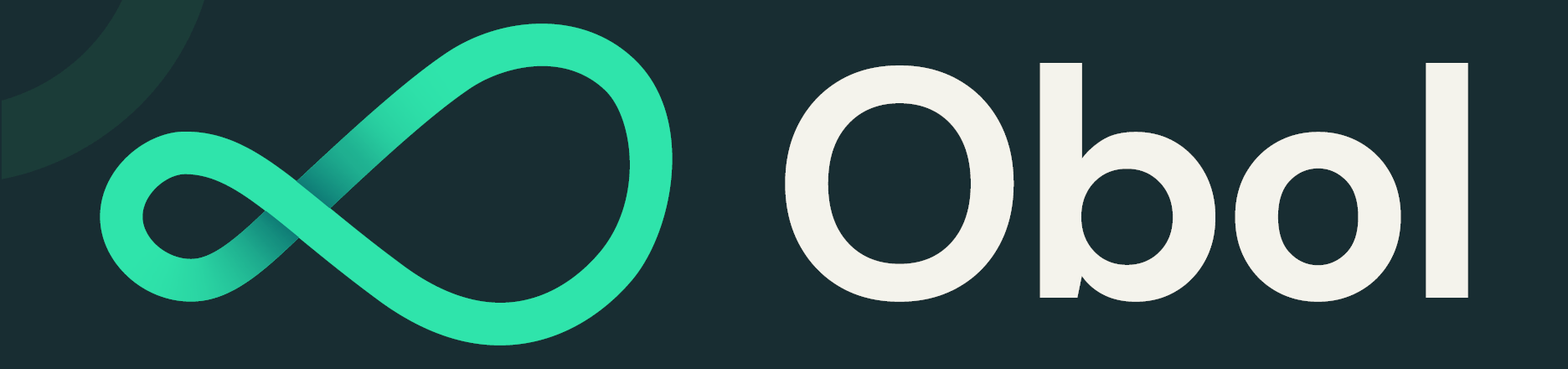 | ||
Checkout our [docs](https://docs.obol.tech/docs/int/quickstart/alone). Further guides and walkthroughs coming soon. | ||
Checkout our [docs](https://docs.obol.tech/docs/int/quickstart). Further guides and walkthroughs coming soon. |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is too big to display
Sorry, the diff of this file is too big to display
Sorry, the diff of this file is not supported yet
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
1834320
47224
6
10