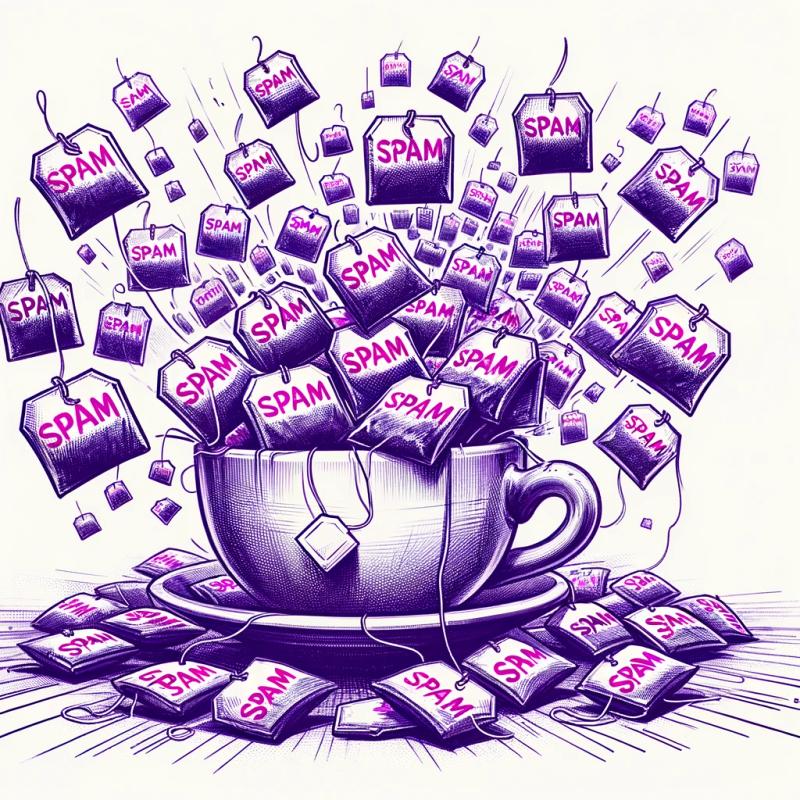
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@onirix/camera-controls-module
Advanced tools
Readme
This library is an addon to Onirix Embed SDK that allows manipulating the camera in a more intuitive and direct way to perform effects such as:
Before accessing any of its functions, the camera module must be initialized by providing an instance of the Onirix Embed SDK as shown below:
import OnirixEmbedSDK from 'https://www.unpkg.com/@onirix/embed-sdk@latest/dist/ox-embed-sdk.esm.js';
import OnirixCameraModule from 'https://www.unpkg.com/@onirix/camera-controls-module@latest/dist/ox-camera-controls-module.esm.js';
const embedSDK = new OnirixEmbedSDK();
embedSDK.connect();
const camera = new OnirixCameraModule(embedSDK);
The camera can be moved using the camera.animate(...)
function. This function receives the following parameters:
position_x
, position_y
, and position_z
: The coordinates the camera should move to. If any of them is
undefined
, the camera will stay in place.look_at_x
, look_at_y
, and look_at_z
: The coordinates the camera should look at. If any of them is undefined
,
the camera will keep looking at the point it is currently looking at.mode
: How to animate the transition to these positions. It is specified by a string with two components: an
interpolator (a component that describes the path the camera should take) and an easing function (a component that
describes the speed of the camera along said path). This string must be formated as '<interpolator> <ease function>'
.
Alternatively, any of the components can be omitted. If the mode
is optional (can be undefined
).There are two interpolators available:
linear
: The camera moves in a straight line.spherical
: The camera moves following and arc around a center.There are seven easing functions available:
linear-in-out
: The camera moves always at the same speed.ease-in
: The camera starts slower.ease-out
: The camera reduces its speed as it reaches its destination.ease-in-out
: The camera starts accelerates at the begining and decelerates as it reaches the end.bounce-in
: The camera anticipates the movement by going in the opposite direction for a short period of time.bounce-out
: The camera overshoots the destination for a short period of time.bounce-in-out
: The camera anticipates the movement by going in the opposite direction and overshoots the
destination for a short period of time.Some examples of valid modes are 'linear'
, 'ease-in-out'
, 'spherical bounce-out'
.
time
: Length of the animation in seconds. If not provided it is assumed to be 0
.center_x
, center_y
, and center_z
: When using a spherical interpolator, the center of the arc. Otherwise it is
ignored.The camera controls are the components that describe how user interactions (touches, mouse clicks, key presses...) are translated to camera movements around the scene. Camera controls are optional and you may use none if the camera cannot be controlled by the user.
Currently, the camera module supports the following camera controls:
camera.enableOrbitControls()
.The function camera.disableControls()
can be used to disable the current camera controls.
Some functions allow setting limits to the transformations that the user can perform:
camera.setOrbitControlsPanRange(min_x, max_x, min_y, max_y, min_z, max_z)
allows setting a minimum and maximum pan
value for each of the coordinate axes.camera.setOrbitControlsRotateRange(min_x, max_x, min_y, max_y, min_z, max_z)
allows setting a minimum and maximum
rotation value for each of the coordiante axes. This rotation is measured as the angle from the camera to the axis.camera.setOrbitControlsZoomRange(min, max)
allows setting a minimum and maximum zoom value.Also, there is a function that allows setting the orbit target: camera.setOrbitControlsTarget(x, y, z)
.
import OnirixEmbedSDK from 'https://www.unpkg.com/@onirix/embed-sdk@latest/dist/ox-embed-sdk.esm.js';
import OnirixCameraModule from 'https://www.unpkg.com/@onirix/camera-controls-module@latest/dist/ox-camera-controls-module.esm.js';
const embedSDK = new OnirixEmbedSDK();
embedSDK.connect();
const camera = new OnirixCameraModule(embedSDK);
camera.enableOrbitControls();
embedSDK.subscribe(OnirixEmbedSDK.Events.SCENE_LOAD_END, () => {
camera.animateTo(
-1, 0, 1,
1, 0, -1,
'spherical ease-in-out',
5
);
});
import OnirixEmbedSDK from 'https://www.unpkg.com/@onirix/embed-sdk@latest/dist/ox-embed-sdk.esm.js';
import OnirixCameraModule from 'https://www.unpkg.com/@onirix/camera-controls-module@latest/dist/ox-camera-controls-module.esm.js';
const embedSDK = new OnirixEmbedSDK();
embedSDK.connect();
const camera = new OnirixCameraModule(embedSDK);
camera.enableOrbitControls();
embedSDK.subscribe(OnirixEmbedSDK.Events.SCENE_LOAD_END, async () => {
await camera.animateTo(
0, 0.5, -5,
0, 0.5, 0
);
await camera.animateTo(
0, 0.5, 5,
0, 0.5, 0,
'spherical ease-in-out',
2,
0, 0.5, 0
);
await camera.animateTo(
0, 0.5, 2,
0, 0.5, 0,
'linear ease-in-out',
2,
0, 0.5, 0
);
});
FAQs
Onirix helper library for camera controls.
The npm package @onirix/camera-controls-module receives a total of 45 weekly downloads. As such, @onirix/camera-controls-module popularity was classified as not popular.
We found that @onirix/camera-controls-module demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.