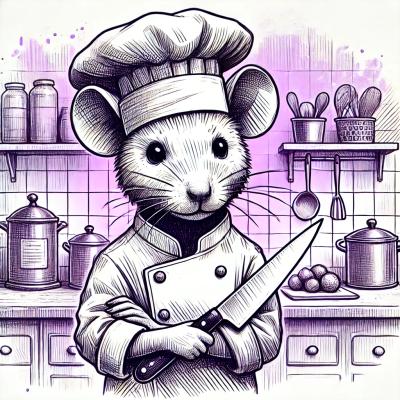
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@opacity-labs/react-native-opacity
Advanced tools
Opacity Networks library for React Native.
yarn add @opacity-labs/react-native-opacity
pod install
Module is a Turbo Module, therefore you need to enable the new arch. (RCT_ENABLE_NEW_ARCH=1 pod install)If you are pulling on the latest version cocoapods sometimes refuses to update it's main repo specs. You can force an update via:
rm -rf ~/.cocoapods/repos/trunk
pod repo update
RCT_ENABLE_NEW_ARCH=1 pod install --repo-update
First add the necessary repos to download the dependencies. On your root build.gradle
add:
allprojects {
repositories {
google()
mavenCentral()
maven { url "https://maven.mozilla.org/maven2/" }
maven { url 'https://jitpack.io' }
}
}
On your apps AndroidManifest.xml
add an activity:
<activity
android:name="com.opacitylabs.opacitycore.InAppBrowserActivity"
android:theme="@style/Theme.AppCompat.DayNight" />
// Just above the closing tag of "application"
</application>
On your main activity you need to initialize the library (kotlin snippet):
import android.os.Bundle // add this import
import com.facebook.react.ReactActivity
import com.facebook.react.ReactActivityDelegate
import com.facebook.react.defaults.DefaultNewArchitectureEntryPoint.fabricEnabled
import com.facebook.react.defaults.DefaultReactActivityDelegate
import com.opacitylabs.opacitycore.OpacityCore // add this import
class MainActivity : ReactActivity() {
override fun onCreate(savedInstanceState: Bundle?) { // add this method
super.onCreate(savedInstanceState)
OpacityCore.initialize(this)
}
// ...
}
You need to make sure react-native.config.js
is properly set up for code generation to work:
module.exports = {
project: {
android: {
packageName: 'your.package.name', // must match your android apps package name, take a look into your apps build.gradle
},
},
};
Once everything is setup you can call the init method on your JS:
import {
init,
getUberRiderPorfile,
OpacityEnvironment,
} from '@opacity-labs/react-native-opacity';
useEffect(() => {
init('Your API key', false, OpacityEnvironment.PRODUCTION);
}, []);
// Later you can call the methods
const res = getUberRiderProfile();
FAQs
Opacity Networks library for React Native
The npm package @opacity-labs/react-native-opacity receives a total of 243 weekly downloads. As such, @opacity-labs/react-native-opacity popularity was classified as not popular.
We found that @opacity-labs/react-native-opacity demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.