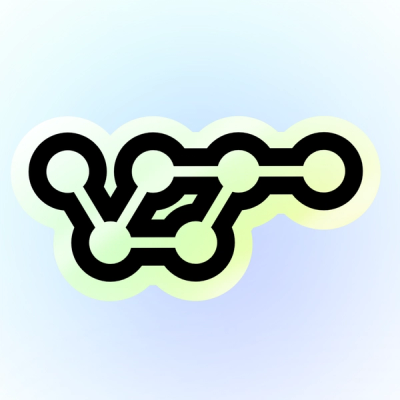
Security News
vlt Debuts New JavaScript Package Manager and Serverless Registry at NodeConf EU
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
@opentelemetry/instrumentation-http
Advanced tools
OpenTelemetry instrumentation for `node:http` and `node:https` http client and server modules
The @opentelemetry/instrumentation-http package is part of the OpenTelemetry project, which provides a collection of tools, APIs, and SDKs to instrument, generate, collect, and export telemetry data (metrics, logs, and traces) to help you analyze your software's performance and behavior. This specific package provides automatic instrumentation for HTTP and HTTPS requests in Node.js applications, allowing developers to capture detailed information about incoming and outgoing requests.
Automatic Tracing of HTTP Requests
This feature automatically traces all HTTP and HTTPS requests made or received by your application. The code sample initializes a NodeTracerProvider, registers it, and then enables the HTTP instrumentation to start tracing HTTP requests.
const { NodeTracerProvider } = require('@opentelemetry/node');
const { HttpInstrumentation } = require('@opentelemetry/instrumentation-http');
const provider = new NodeTracerProvider();
provider.register();
const httpInstrumentation = new HttpInstrumentation();
httpInstrumentation.enable();
Configurable Instrumentation
This feature allows developers to configure which requests should be ignored by the instrumentation. In the code sample, the instrumentation is configured to ignore incoming requests to '/healthz' and outgoing requests to 'example.com'.
const { HttpInstrumentation } = require('@opentelemetry/instrumentation-http');
const httpInstrumentation = new HttpInstrumentation({
ignoreIncomingPaths: [ /healthz/ ],
ignoreOutgoingUrls: [ /example.com/ ]
});
httpInstrumentation.enable();
dd-trace is a DataDog tracing library that also provides automatic instrumentation for HTTP requests among other integrations. It is similar to @opentelemetry/instrumentation-http but is specifically tailored for integration with DataDog's monitoring and analytics platform, whereas OpenTelemetry provides vendor-neutral instrumentation.
Note: This is an experimental package under active development. New releases may include breaking changes.
This module provides automatic instrumentation for http
and https
.
For automatic instrumentation see the @opentelemetry/sdk-trace-node package.
npm install --save @opentelemetry/instrumentation-http
>=14
OpenTelemetry HTTP Instrumentation allows the user to automatically collect trace data and export them to their backend of choice, to give observability to distributed systems.
To load a specific instrumentation (HTTP in this case), specify it in the Node Tracer's configuration.
const { HttpInstrumentation } = require('@opentelemetry/instrumentation-http');
const {
ConsoleSpanExporter,
NodeTracerProvider,
SimpleSpanProcessor,
} = require('@opentelemetry/sdk-trace-node');
const { registerInstrumentations } = require('@opentelemetry/instrumentation');
const provider = new NodeTracerProvider();
provider.addSpanProcessor(new SimpleSpanProcessor(new ConsoleSpanExporter()));
provider.register();
registerInstrumentations({
instrumentations: [new HttpInstrumentation()],
});
See examples/http for a short example.
Http instrumentation has few options available to choose from. You can set the following:
Options | Type | Description |
---|---|---|
applyCustomAttributesOnSpan | HttpCustomAttributeFunction | Function for adding custom attributes |
requestHook | HttpRequestCustomAttributeFunction | Function for adding custom attributes before request is handled |
responseHook | HttpResponseCustomAttributeFunction | Function for adding custom attributes before response is handled |
startIncomingSpanHook | StartIncomingSpanCustomAttributeFunction | Function for adding custom attributes before a span is started in incomingRequest |
startOutgoingSpanHook | StartOutgoingSpanCustomAttributeFunction | Function for adding custom attributes before a span is started in outgoingRequest |
ignoreIncomingRequestHook | IgnoreIncomingRequestFunction | Http instrumentation will not trace all incoming requests that matched with custom function |
ignoreOutgoingRequestHook | IgnoreOutgoingRequestFunction | Http instrumentation will not trace all outgoing requests that matched with custom function |
disableOutgoingRequestInstrumentation | boolean | Set to true to avoid instrumenting outgoing requests at all. This can be helpful when another instrumentation handles outgoing requests. |
disableIncomingRequestInstrumentation | boolean | Set to true to avoid instrumenting incoming requests at all. This can be helpful when another instrumentation handles incoming requests. |
serverName | string | The primary server name of the matched virtual host. |
requireParentforOutgoingSpans | Boolean | Require that is a parent span to create new span for outgoing requests. |
requireParentforIncomingSpans | Boolean | Require that is a parent span to create new span for incoming requests. |
headersToSpanAttributes | object | List of case insensitive HTTP headers to convert to span attributes. Client (outgoing requests, incoming responses) and server (incoming requests, outgoing responses) headers will be converted to span attributes in the form of http.{request|response}.header.header_name , e.g. http.response.header.content_length |
Prior to version 0.54
, this instrumentation created spans targeting an experimental semantic convention Version 1.7.0.
This package is capable of emitting both Semantic Convention Version 1.7.0 and Version 1.27.0.
It is controlled using the environment variable OTEL_SEMCONV_STABILITY_OPT_IN
, which is a comma separated list of values.
The values http
and http/dup
control this instrumentation.
See details for the behavior of each of these values below.
If neither http
or http/dup
is included in OTEL_SEMCONV_STABILITY_OPT_IN
, the old experimental semantic conventions will be used by default.
Enabled when OTEL_SEMCONV_STABILITY_OPT_IN
contains http
OR http/dup
.
This is the recommended configuration, and will soon become the default behavior.
Follow all requirements and recommendations of HTTP Client and Server Semantic Conventions Version 1.27.0 for spans and metrics, including all required and recommended attributes.
Metrics Exported:
When upgrading to the new semantic conventions, it is recommended to do so in the following order:
@opentelemetry/instrumentation-http
to the latest versionOTEL_SEMCONV_STABILITY_OPT_IN=http/dup
to emit both old and new semantic conventionsOTEL_SEMCONV_STABILITY_OPT_IN=http
to emit only the new semantic conventionsThis will cause both the old and new semantic conventions to be emitted during the transition period.
Enabled when OTEL_SEMCONV_STABILITY_OPT_IN
contains http/dup
or DOES NOT CONTAIN http
.
This is the current default behavior.
Create HTTP client spans which implement Semantic Convention Version 1.7.0.
When OTEL_SEMCONV_STABILITY_OPT_IN
is not set or includes http/dup
, this module implements Semantic Convention Version 1.7.0.
Attributes collected:
Attribute | Short Description |
---|---|
ip_tcp | Transport protocol used |
ip_udp | Transport protocol used |
http.client_ip | The IP address of the original client behind all proxies, if known |
http.flavor | Kind of HTTP protocol used |
http.host | The value of the HTTP host header |
http.method | HTTP request method |
http.request_content_length | The size of the request payload body in bytes |
http.request_content_length_uncompressed | The size of the uncompressed request payload body after transport decoding |
http.response_content_length | The size of the response payload body in bytes |
http.response_content_length_uncompressed | The size of the uncompressed response payload body after transport decoding |
http.route | The matched route (path template). |
http.scheme | The URI scheme identifying the used protocol |
http.server_name | The primary server name of the matched virtual host |
http.status_code | HTTP response status code |
http.target | The full request target as passed in a HTTP request line or equivalent |
http.url | Full HTTP request URL in the form scheme://host[:port]/path?query[#fragment] |
http.user_agent | Value of the HTTP User-Agent header sent by the client |
net.host.ip | Like net.peer.ip but for the host IP. Useful in case of a multi-IP host |
net.host.name | Local hostname or similar |
net.host.port | Like net.peer.port but for the host port |
net.peer.ip. | Remote address of the peer (dotted decimal for IPv4 or RFC5952 for IPv6) |
net.peer.name | Remote hostname or similar |
net.peer.port | Remote port number |
net.transport | Transport protocol used |
Apache 2.0 - See LICENSE for more information.
FAQs
OpenTelemetry instrumentation for `node:http` and `node:https` http client and server modules
The npm package @opentelemetry/instrumentation-http receives a total of 2,519,166 weekly downloads. As such, @opentelemetry/instrumentation-http popularity was classified as popular.
We found that @opentelemetry/instrumentation-http demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Security News
Research
The Socket Research Team uncovered a malicious Python package typosquatting the popular 'fabric' SSH library, silently exfiltrating AWS credentials from unsuspecting developers.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.