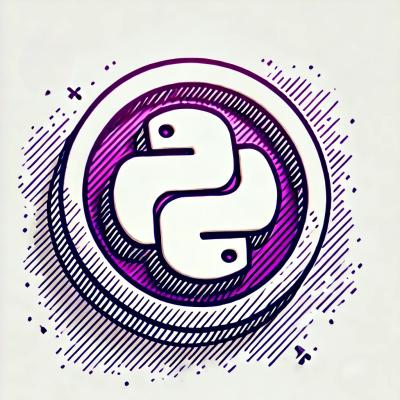
Security News
Introducing the Socket Python SDK
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
@opentelemetry/instrumentation-mongodb
Advanced tools
OpenTelemetry mongodb automatic instrumentation package.
@opentelemetry/instrumentation-mongodb is an npm package that provides automatic tracing for MongoDB operations using the OpenTelemetry framework. It helps in monitoring and collecting performance metrics and distributed traces for MongoDB operations, which can be useful for debugging and optimizing database interactions.
Automatic Tracing
This feature allows you to automatically trace MongoDB operations. The code sample shows how to set up the NodeTracerProvider and register the MongoDB instrumentation to start collecting traces.
const { NodeTracerProvider } = require('@opentelemetry/node');
const { registerInstrumentations } = require('@opentelemetry/instrumentation');
const { MongoDBInstrumentation } = require('@opentelemetry/instrumentation-mongodb');
const provider = new NodeTracerProvider();
provider.register();
registerInstrumentations({
instrumentations: [
new MongoDBInstrumentation(),
],
});
Custom Span Attributes
This feature allows you to add custom attributes to the spans created for MongoDB operations. The code sample demonstrates how to use the `responseHook` to add the MongoDB operation result as a span attribute.
const { MongoDBInstrumentation } = require('@opentelemetry/instrumentation-mongodb');
const mongoInstrumentation = new MongoDBInstrumentation({
responseHook: (span, result) => {
span.setAttribute('mongodb.result', JSON.stringify(result));
},
});
Error Handling
This feature allows you to handle errors in MongoDB operations and set the span status accordingly. The code sample shows how to use the `responseHook` to check if the result is an error and set the span status.
const { MongoDBInstrumentation } = require('@opentelemetry/instrumentation-mongodb');
const mongoInstrumentation = new MongoDBInstrumentation({
responseHook: (span, result) => {
if (result instanceof Error) {
span.setStatus({ code: 2, message: result.message });
}
},
});
The `mongodb` package is the official MongoDB driver for Node.js. While it does not provide automatic tracing, it is often used in conjunction with tracing libraries like OpenTelemetry to manually instrument MongoDB operations.
Mongoose is an ODM (Object Data Modeling) library for MongoDB and Node.js. It provides a higher-level abstraction for MongoDB operations but does not include built-in tracing capabilities. However, it can be instrumented using OpenTelemetry for tracing.
This package provides automatic tracing for HTTP requests. While it is not specific to MongoDB, it can be used alongside @opentelemetry/instrumentation-mongodb to provide a more comprehensive tracing solution for applications that interact with MongoDB over HTTP.
This module provides automatic instrumentation for the mongodb
module, which may be loaded using the @opentelemetry/sdk-trace-node
package and is included in the @opentelemetry/auto-instrumentations-node
bundle.
If total installation size is not constrained, it is recommended to use the @opentelemetry/auto-instrumentations-node
bundle with @opentelemetry/sdk-node for the most seamless instrumentation experience.
Compatible with OpenTelemetry JS API and SDK 1.0+
.
npm install --save @opentelemetry/instrumentation-mongodb
>=3.3 <7
OpenTelemetry MongoDB Instrumentation allows the user to automatically collect trace data and export them to their backend of choice, to give observability to distributed systems.
To load a specific instrumentation (mongodb in this case), specify it in the Node Tracer's configuration.
const { MongoDBInstrumentation } = require('@opentelemetry/instrumentation-mongodb');
const { NodeTracerProvider } = require('@opentelemetry/sdk-trace-node');
const { registerInstrumentations } = require('@opentelemetry/instrumentation');
const provider = new NodeTracerProvider();
provider.register();
registerInstrumentations({
instrumentations: [
new MongoDBInstrumentation({
// see under for available configuration
}),
],
});
See examples/mongodb
for a short example.
Mongodb instrumentation has few options available to choose from. You can set the following:
Options | Type | Description |
---|---|---|
enhancedDatabaseReporting | string | If true, additional information about query parameters and results will be attached (as attributes ) to spans representing database operations |
responseHook | MongoDBInstrumentationExecutionResponseHook (function) | Function for adding custom attributes from db response |
dbStatementSerializer | DbStatementSerializer (function) | Custom serializer function for the db.statement tag |
Apache 2.0 - See LICENSE for more information.
FAQs
OpenTelemetry instrumentation for `mongodb` database client for MongoDB
The npm package @opentelemetry/instrumentation-mongodb receives a total of 2,065,001 weekly downloads. As such, @opentelemetry/instrumentation-mongodb popularity was classified as popular.
We found that @opentelemetry/instrumentation-mongodb demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.
Security News
A new Rust RFC proposes "Trusted Publishing" for Crates.io, introducing short-lived access tokens via OIDC to improve security and reduce risks associated with long-lived API tokens.