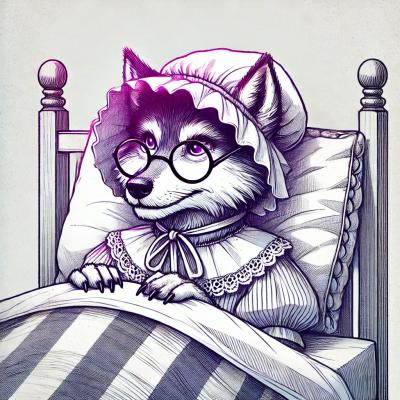
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@opentelemetry/sdk-trace-node
Advanced tools
OpenTelemetry Node SDK provides automatic telemetry (tracing, metrics, etc) for Node.js applications
The @opentelemetry/sdk-trace-node package is part of the OpenTelemetry JavaScript ecosystem and provides a Node.js SDK for tracing. It allows developers to collect and export trace data in their Node.js applications to analyze application performance and troubleshoot issues. The SDK supports various exporters and can be configured to send trace data to different backends for monitoring.
Initializing Tracing
This code initializes the Node.js tracing provider and sets up a simple span processor with a console exporter, which will print the trace data to the console.
const { NodeTracerProvider } = require('@opentelemetry/sdk-trace-node');
const { SimpleSpanProcessor } = require('@opentelemetry/tracing');
const { ConsoleSpanExporter } = require('@opentelemetry/exporter-console');
const provider = new NodeTracerProvider();
provider.addSpanProcessor(new SimpleSpanProcessor(new ConsoleSpanExporter()));
provider.register();
Configuring Exporters
This code snippet demonstrates how to configure the NodeTracerProvider to use the Jaeger exporter, which sends trace data to a Jaeger backend for analysis.
const { JaegerExporter } = require('@opentelemetry/exporter-jaeger');
const provider = new NodeTracerProvider();
const jaegerExporter = new JaegerExporter({
serviceName: 'my-service',
});
provider.addSpanProcessor(new SimpleSpanProcessor(jaegerExporter));
provider.register();
Automatic Instrumentation
This code sets up automatic instrumentation for Node.js applications, which automatically collects trace data from supported libraries without manual instrumentation.
const { NodeTracerProvider } = require('@opentelemetry/sdk-trace-node');
const { getNodeAutoInstrumentations } = require('@opentelemetry/auto-instrumentations-node');
const provider = new NodeTracerProvider();
provider.register();
require('@opentelemetry/instrumentation').registerInstrumentations({
instrumentations: [getNodeAutoInstrumentations()],
});
The dd-trace package is Datadog's APM tracing library for Node.js. It provides automatic instrumentation for popular frameworks and libraries. Compared to @opentelemetry/sdk-trace-node, dd-trace is more tightly integrated with Datadog's monitoring services but less vendor-neutral.
Elastic APM Node.js Agent is Elastic's solution for tracing and monitoring Node.js applications. It automatically instruments the code and captures errors and transactions. Similar to @opentelemetry/sdk-trace-node, it provides detailed performance insights but is designed to work seamlessly with the Elastic Stack.
New Relic's APM Agent for Node.js is a monitoring and tracing tool that provides deep insights into application performance. It differs from @opentelemetry/sdk-trace-node in that it is part of New Relic's proprietary APM platform, offering extensive integration with their suite of monitoring tools.
This module provides automated instrumentation and tracing for Node.js applications.
For manual instrumentation see the @opentelemetry/sdk-trace-base package.
This package exposes a NodeTracerProvider
.
For loading instrumentations please use registerInstrumentations
function from opentelemetry-instrumentation
OpenTelemetry comes with a growing number of instrumentation plugins for well known modules (see supported modules) and an API to create custom instrumentation (see the instrumentation developer guide).
Please note: This module does not bundle any plugins. They need to be installed separately.
This is done by wrapping all tracing-relevant functions.
This instrumentation code will automatically
Additionally to automated instrumentation, NodeTracerProvider
exposes the same API as @opentelemetry/sdk-trace-base, allowing creating custom spans if needed.
npm install --save @opentelemetry/api
npm install --save @opentelemetry/sdk-trace-node
# Install instrumentation plugins
npm install --save @opentelemetry/instrumentation-http
# and for example one additional
npm install --save instrumentation-graphql
The following code will configure the NodeTracerProvider
to instrument http
(and any other installed supported
modules)
using @opentelemetry/plugin-http
.
const { registerInstrumentations } = require('@opentelemetry/instrumentation');
const { NodeTracerProvider } = require('@opentelemetry/sdk-trace-node');
// Create and configure NodeTracerProvider
const provider = new NodeTracerProvider();
// Initialize the provider
provider.register();
// register and load instrumentation and old plugins - old plugins will be loaded automatically as previously
// but instrumentations needs to be added
registerInstrumentations({
});
// Your application code - http will automatically be instrumented if
// @opentelemetry/plugin-http is present
const http = require('http');
In the following example:
requestHook
const { registerInstrumentations } = require('@opentelemetry/instrumentation');
const { HttpInstrumentation } = require('@opentelemetry/instrumentation-http');
const { ExpressInstrumentation } = require('@opentelemetry/instrumentation-express');
const provider = new NodeTracerProvider();
provider.register();
// register and load instrumentation and old plugins - old plugins will be loaded automatically as previously
// but instrumentations needs to be added
registerInstrumentations({
instrumentations: [
new ExpressInstrumentation(),
new HttpInstrumentation({
requestHook: (span, request) => {
span.setAttribute("custom request hook attribute", "request");
},
}),
],
});
See how to automatically instrument http and gRPC / grpc-js using node-sdk.
Apache 2.0 - See LICENSE for more information.
1.1.1
FAQs
OpenTelemetry Node SDK provides automatic telemetry (tracing, metrics, etc) for Node.js applications
The npm package @opentelemetry/sdk-trace-node receives a total of 1,501,512 weekly downloads. As such, @opentelemetry/sdk-trace-node popularity was classified as popular.
We found that @opentelemetry/sdk-trace-node demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.