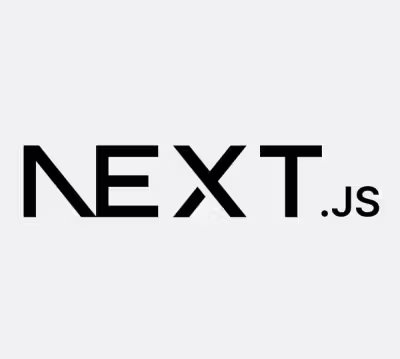
Security News
Next.js Patches Critical Middleware Vulnerability (CVE-2025-29927)
Next.js has patched a critical vulnerability (CVE-2025-29927) that allowed attackers to bypass middleware-based authorization checks in self-hosted apps.
@opentelemetry/sdk-trace-web
Advanced tools
@opentelemetry/sdk-trace-web is a package that provides OpenTelemetry tracing capabilities specifically for web applications. It allows developers to capture and export trace data from web applications, which can be used for performance monitoring, debugging, and observability.
Initialize Tracer
This code initializes a tracer provider for web applications, sets up a span processor to export spans to the console, and registers the provider. The tracer can then be used to create and manage spans.
const { WebTracerProvider } = require('@opentelemetry/sdk-trace-web');
const { ConsoleSpanExporter, SimpleSpanProcessor } = require('@opentelemetry/sdk-trace-base');
const provider = new WebTracerProvider();
provider.addSpanProcessor(new SimpleSpanProcessor(new ConsoleSpanExporter()));
provider.register();
const tracer = provider.getTracer('example-tracer-web');
Create and End a Span
This code demonstrates how to create a span, simulate some work with a timeout, and then end the span. Spans represent units of work and are used to measure the time taken for operations.
const span = tracer.startSpan('example-span');
// Simulate some work
setTimeout(() => {
span.end();
}, 1000);
Add Attributes to a Span
This code shows how to add attributes to a span. Attributes are key-value pairs that provide additional context about the span.
const span = tracer.startSpan('example-span');
span.setAttribute('key', 'value');
span.end();
Add Events to a Span
This code demonstrates how to add events to a span. Events are time-stamped annotations that provide additional information about the span.
const span = tracer.startSpan('example-span');
span.addEvent('event-name', { 'key': 'value' });
span.end();
jaeger-client is a library for instrumenting applications with Jaeger tracing. It provides similar capabilities to @opentelemetry/sdk-trace-web, enabling developers to capture and export trace data. Like zipkin-js, jaeger-client is specific to the Jaeger tracing system, while @opentelemetry/sdk-trace-web is part of the more versatile OpenTelemetry project.
elastic-apm-js-base is a library for instrumenting web applications with Elastic APM tracing. It offers similar functionality to @opentelemetry/sdk-trace-web, allowing developers to capture and export trace data to Elastic APM. Unlike @opentelemetry/sdk-trace-web, which is part of the OpenTelemetry project, elastic-apm-js-base is specific to the Elastic APM ecosystem.
This module provides automated instrumentation and tracing for Web applications.
For manual instrumentation see the @opentelemetry/sdk-trace-base package.
This package exposes a class WebTracerProvider
that will be able to automatically trace things in Browser only.
See the example how to use it.
OpenTelemetry comes with a growing number of instrumentations for well know modules (see supported modules) and an API to create custom instrumentations (see the instrumentation developer guide).
Web Tracer currently supports one plugin for document load.
Unlike Node Tracer (NodeTracerProvider
), the plugins needs to be initialized and passed in configuration.
The reason is to give user full control over which plugin will be bundled into web page.
You can choose to use the ZoneContextManager
if you want to trace asynchronous operations. Please note that the ZoneContextManager
does not work with JS code targeting ES2017+
. In order to use the ZoneContextManager
, please transpile back to ES2015
.
npm install --save @opentelemetry/sdk-trace-web
import {
ConsoleSpanExporter,
SimpleSpanProcessor,
WebTracerProvider,
} from '@opentelemetry/sdk-trace-web';
import { DocumentLoad } from '@opentelemetry/plugin-document-load';
import { ZoneContextManager } from '@opentelemetry/context-zone';
import { registerInstrumentations } from '@opentelemetry/instrumentation';
const provider = new WebTracerProvider({
spanProcessors: [new SimpleSpanProcessor(new ConsoleSpanExporter())]
});
provider.register({
// Changing default contextManager to use ZoneContextManager - supports asynchronous operations - optional
contextManager: new ZoneContextManager(),
});
// Registering instrumentations / plugins
registerInstrumentations({
instrumentations: [
new DocumentLoad(),
],
});
Apache 2.0 - See LICENSE for more information.
2.0.0
^18.19.0 || >=20.6.0
. This means that support for Node.js 14 and 16 has been dropped.0.200.0
are compatible with this releaseparentSpanContext
and remove parentSpanId
from Span
and ReadableSpan
#5450 @JacksonWeber
Span
s parentSpanId
was replaced by parentSpanContext
, to migrate to the new property, please replace span.parentSpanId
-> span.parentSpanContext?.spanId
type
field on MetricDescriptor
#5291 @chancancodeInstrumentDescriptor
type; use MetricDescriptor
instead #5277 @chancancode@opentelemetry/api
peer dependency to 1.9.0 #5254 @chancancodeSpanAttributes
with Attributes
#5009 @david-lunaResourceAttributes
with Attributes
#5016 @david-lunaView
and Aggregation
in favor of ViewOptions
and AggregationOption
#4931 @pichlermarcnew Span
constructor in favor of Tracer.startSpan
API #5048 @david-lunaBasicTracerProvider.addSpanProcessor
API in favor of constructor options. #5134 @david-lunaresource
property private in BasicTracerProvider
and remove getActiveSpanProcessor
API. #5192 @david-lunaIMetricReader
interface and use it over abstract class #5311
MeterProviderOptions
now provides the more general IMetricReader
type over MetricReader
MetricReader
in your public interface, consider accepting the more general IMetricReader
instead to avoid unintentional breaking changesBasicTracerProvider
, the class offered multiple methods to facilitate the creation of exporters and auto-pairing with SpanProcessor
s.
SpanProcessor
s to the base class constructor when extending_registeredExporters
has been removed_getSpanExporter
has been removed_buildExporterFromEnv
has been removedIdGenerator
and RandomIdGenerator
#5309 @pichlermarcInstrumentationLibrary
#5308 @pichlermarc
InstrumentationScope
insteadInstrumentationLibrary
with InstrumentationScope
#5308 @pichlermarc
Tracer.instrumentationLibrary
-> Tracer.instrumentationScope
ReadableSpan.instrumentationLibrary
-> ReadableSpan.instrumentationScope
ReadableSpan
ReadableSpan.instrumentationScope
over ReadableSpan.instrumentationLibrary
#5308 @pichlermarcReadableSpan.instrumentationScope
over ReadableSpan.instrumentationLibrary
#5308 @pichlermarc5.0.4
#5145 @david-luna
typescript@<5.0.4
typescript
in minor releases. We will only drop support for versions that are older than 2 years.AlwaysOnSampler
has moved to @opentelemetry/sdk-trace-base
AlwaysOffSampler
has moved to @opentelemetry/sdk-trace-base
ParentBasedSampler
has moved to @opentelemetry/sdk-trace-base
TraceIdRatioSampler
has moved to @opentelemetry/sdk-trace-base
attributes
objectDetectedResource
plain objects instead of new Resource()
BasicTracerProvider#register()
.
OTEL_PROPAGATORS
or window.OTEL_PROPAGATORS
anymore, please pass the propagator to NodeTracerProvider#register()
instead.BasicTracerProvider#register()
will now fall back to defaults (tracecontext
and baggage
)NodeTracerProvider#register()
.
OTEL_PROPAGATORS
anymore, please pass the propagator to NodeTracerProvider#register()
instead.NodeTracerProvider#register()
will now fall back to the defaults (tracecontext
and baggage
)NodeSDK
from @opentelemetry/sdk-node
.WebTracerProvider#register()
.
window.OTEL_PROPAGATORS
anymore, please pass the propagator to WebTracerProvider#register()
instead.WebTracerProvider#register()
will now fall back to defaults (tracecontext
and baggage
)EXPORTER_FACTORY
is not used anymore and has been removedPROPAGATOR_FACTORY
is not used anymore and has been removedForceFlushState
was intended for internal use and has been removedTracer
class was unintentionally exported and has been removed
Tracer
, please use BasicTracerProvider#getTracer()
, NodeTracerProvider#getTracer()
or WebTracerProvider#getTracer()
Tracer
, please use the Tracer
type from @opentelemetry/api
^18.19.0 || >=20.6.0
. Support for Node.js 14, 16, and early minor versions of 18 and 20 have been dropped. This applies to all packages except the 'api' and 'semantic-conventions' packages. #5395 @trentm@opentelemetry/resource
in favor of @opentelemetry/opentelemetry-browser-detector
#5420VERSION
was an internal constant that was unintentionally exported. It has been removed without replacement.isWrapped
has been removed in favor of isWrapped
from @opentelemetry/instrumentation
ShimWrapped
has been removed in favor of ShimWrapped
from @opentelemetry/instrumentation
hexToBase64
was a utility function that is not used by the SDK anymore. It has been removed without replacement.hexToBinary
was a utility function that now internal to @opentelemetry/otlp-tranformer
. It has been removed without replacement.baggageUtils.getKeyParis
was an internal utility function that was unintentionally exported. It has been removed without replacement.baggageUtils.serializeKeyPairs
was an internal utility function that was unintentionally exported. It has been removed without replacement.baggageUtils.parseKeyPairsIntoRecord,
has been removed in favor of parseKeyPairsIntoRecord
baggageUtils.parsePairKeyValue
was an internal utility function that was unintentionally exported. It has been removed without replacement.TimeOriginLegacy
has been removed without replacement.isAttributeKey
was an internal utility function that was unintentionally exported. It has been removed without replacement.window.OTEL_*
is now not supported anymore, please pass configuration options to constructors instead.window.OTEL_*
is now not supported anymore, please pass configuration options to constructors instead.Resource
class to ResourceImpl
and makes it package-privateIResource
interface to Resource
resourceFromAttributes
to create a Resource
from a DetectedAttributes
objectdefaultResource
to create a default resource #5467 @pichlermarcemptyResource
to create an empty resource #5467 @pichlermarcwindow.OTEL_*
is now not supported anymore
envDetector
in browser environments, please migrate to manually creating a resource.ParentBasedAlwaysOnSampler
over AlwaysOnSampler
when bogus data is supplied to OTEL_TRACES_SAMPLER
getEnv()
, getEnvWithoutDefaults()
#5481 @pichlermarc
getEnv()
has been replaced by getStringFromEnv()
, getNumberFromEnv()
, getBooleanFromEnv()
, getStringListFromEnv()
getStringFromEnv("OTEL_FOO") ?? "my-default"
)getEnvWithoutDefaults()
has been replaced by getStringFromEnv()
, getNumberFromEnv()
, getBooleanFromEnv()
, getStringListFromEnv()
DEFAULT_ENVIRONMENT
has been removed, please inline any defaults from now on
ENVIRONMENT
has been removed without replacementRAW_ENVIRONMENT
has been removed without replacementparseEnvironment
has been removed without replacementBasicTracerProvider#register()
to improve tree-shaking #5503 @pichlermarc
BasicTracerProvider#register()
has been removed
propagation.setGlobalPropagator()
from @opentelemetry/api
context.setGlobalContextManager()
from @opentelemetry/api
@opentelemetry/api
, @opentelemetry/api-logs
, @opentelemetry/api-events
, and @opentelemetry/semantic-conventions
#5456 @david-luna
DEFAULT_ATTRIBUTE_VALUE_LENTGHT_LIMIT
has been removed, please use Infinity
insteadDEFAULT_ATTRIBUTE_VALUE_COUNT_LIMIT
has been removed, please use 128
insteadDEFAULT_SPAN_ATTRIBUTE_PER_EVENT_COUNT_LIMIT
has been removed, please use 128
insteadDEFAULT_SPAN_ATTRIBUTE_PER_LINK_COUNT_LIMIT
has been removed, please use 128
insteadWebTracerProvider
constructor now does not throw anymore when contextManager
or propagator
are passed as extra options to the constructordiagLogLevelFromString
utility #5475 @pichlermarcurl.parse
from node:url
#5390 @chancancodePeriodicExportingMetricReader
when there are no metrics to export. #5288 @jacksonweberInstrumentDescriptor
type now extends MetricDescriptor
; moved public InstrumentType
type enum into ./src/export/MetricData.ts
#5277Gauge
and MetricAdvice
workaround types in favor of the upstream @opentelemetry/api
types #5254 @chancancodeBasicTracerProvider._registeredSpanProcessors
private property. #5134 @david-lunaBasicTracerProvider.activeSpanProcessor
private property. #5211 @david-lunamodule
compiler option to node16
. #5347 @david-lunasemver
package with internal semantic versioning check implementation to get rid of semver
package initialization overhead especially in the AWS Lambda environment during coldstart #5305 @serkan-ozal@opentelemetry/semantic-conventions
dep to allow better de-duplication in installs #5439 @trentmFAQs
OpenTelemetry Web Tracer
The npm package @opentelemetry/sdk-trace-web receives a total of 689,073 weekly downloads. As such, @opentelemetry/sdk-trace-web popularity was classified as popular.
We found that @opentelemetry/sdk-trace-web demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Next.js has patched a critical vulnerability (CVE-2025-29927) that allowed attackers to bypass middleware-based authorization checks in self-hosted apps.
Security News
A survey of 500 cybersecurity pros reveals high pay isn't enough—lack of growth and flexibility is driving attrition and risking organizational security.
Product
Socket, the leader in open source security, is now available on Google Cloud Marketplace for simplified procurement and enhanced protection against supply chain attacks.