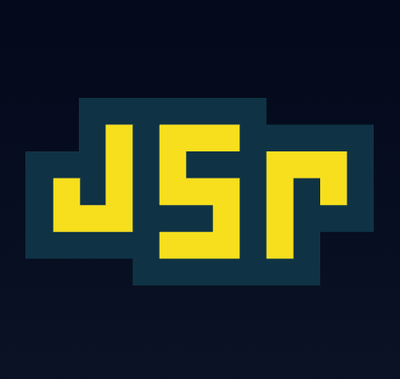
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@orca-so/sdk
Advanced tools
The Orca SDK contains a set of simple to use APIs to allow developers to integrate with the Orca exchange platform.
Learn more Orca here.
Supported Orca Pools
Features Coming Soon
Use your environment's package manager to install @orca-so/sdk and other related packages into your project.
yarn add @orca.so/sdk @solana/web3.js decimal.js
npm install @orca.so/sdk @solana/web3.js decimal.js
import { Connection, Keypair } from "@solana/web3.js";
import { getOrca, OrcaPoolConfig, OrcaU64 } from "@orca-so/sdk";
try {
const connection = new Connection(url, "singleGossip");
const orca = getOrca(connection);
const owner: Keypair = getKeyPair();
// Get an instance of the ETH-USDC orca pool
let pool = orca.getPool(OrcaPoolConfig.ETH_USDC);
// Get the number of ETH-USDC LP tokens in your wallet
let ethUsdcLPBalance = await pool.getLPBalance(owner.publicKey);
// Get the total supply of ETH-USDC LP tokens
let ethUsdcLPSupply = await pool.getLPSupply();
// Get a quote of exchanging 1.1 ETH to USDC with a slippage tolerance of 0.1%
// From the quote, you can get the current rate, fees, expected output amount and minimum output amount
let usdcToken = pool.getTokenB();
let tradeValue = new Decimal(1.1);
let quote = await pool.getQuote(usdcToken, tradeValue, new Decimal(0.1));
// Perform a swap for 1USDC to the quoted minimum amount of ETH
const txId = await pool.swap(owner, usdcToken, tradeValue, quote.getMinOutputAmount());
} catch (err) {
// Handle errors
}
Decimals & OrcaU64 The SDK relies on the use of Decimal for number inputs and Decimal/OrcaU64 for token-value inputs. If a Decimal instance is provided for a token-value input, it will be automatically transformed to the token's scale.
Funding Associated Token Addresses The swap() function assumes the owner keypair address has already created & initialized the associated token addresses for the trading pair tokens. The swap will fail if this is not the case.
Integration Questions
Have problems integrating with the SDK? Pop by over to our Discord #integrations channel and chat with one of our engineers.
Issues / Bugs
If you found a bug, open up an issue on github with the prefix [ISSUE]. To help us be more effective in resolving the problem, be specific in the steps it took to reproduce the problem (ex. when did the issue occur, code samples, debug logs etc).
Feedback
Got ideas on how to improve the system? Open up an issue on github with the prefix [FEEDBACK] and let's brainstorm more about it together!
FAQs
Typescript SDK for the Orca protocol.
The npm package @orca-so/sdk receives a total of 2,304 weekly downloads. As such, @orca-so/sdk popularity was classified as popular.
We found that @orca-so/sdk demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.