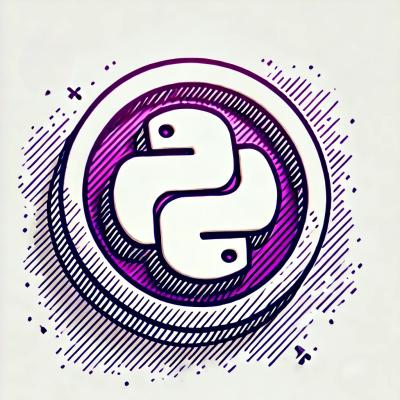
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
@orchestrator/ngx-testing
Advanced tools
Testing utilities for Angular projects
npm install -D @orchestrator/ngx-testing
Because you want to test your code and not writing testing code to test your code.
This utility library will generate a wrapping (host) component for your component
under test and bind all the @Input
s and @Output
s for you so you can
interact with component as the consumer will.
That also means that you do not need to worry about life-cycle hooks hassle because auto generated code will move this bit to Angular itself.
Library will initialize every @Output
property on host component to a mock
so you have a nice time checking if the output was triggered.
By default it is simply a NOOP function (that does nothing).
But which testing tool do you use is up to you so you have to tell us what to use by writing next code in your main test configuration file:
import { setOutputMock } from '@orchestrator/ngx-testing';
// Use this for Jasmine Spies
setOutputMock(() => jasmine.createSpy());
// Or this for Jest Mocks
setOutputMock(() => jest.fn());
// Optionally you can provide typings for mocks
declare module '@orchestrator/ngx-testing' {
// Use this for Jasmine Spies
interface OutputMock<T> extends jasmine.Spy {}
// Or this for Jest Mocks
interface OutputMock<T> extends jest.Mock {}
// <T> here represents actual type from `EventEmitter<T>`
// You can use it if you need it
}
Let's see how to test a component with this library:
import { TestBed } from '@angular/core/testing';
import { getTestingForComponent } from '@orchestrator/ngx-testing';
// This is component under test
@Component({ selector: 'my-component', template: 'Text is: {{text}}' })
class MyComponent implements OnInit, OnChanges {
@Input() text: string;
}
describe('MyComponent', () => {
// This will generate host component and module with everything required
const { testModule, createComponent } = getTestingForComponent(MyComponent);
// Configure testing module by importing generated module before
beforeEach(() => TestBed.configureTestingModule({ imports: [testModule] }));
it('should render input text', async () => {
// Now simply create host component
// It is async because it performs compilation of templates
// And returns a special Host service that contains a bunch of useful stuff
const host = await createComponent();
// To interact with your component Inputs/Outputs use `hostComponent` property
// It's an instance of generated host component that binds all Inputs and Outputs
host.hostComponent.text = 'something';
// This is an alias to fixture.detectChanges()
// Fixture can be used as well and is available as `fixture` property
host.detectChanges();
// htmlElement is simply an HTMLElement of rendered component under test
expect(host.htmlElement.textContent).toBe(`Text is: something`);
});
});
For more examples visit example-component.spec.ts.
Sometimes you will need to provide extra config for your component/directive under test so it can work in isolated unit test. You can do this like so:
import { getTestingForComponent, getTestingForDirective } from '@orchestrator/ngx-testing';
// For component
getTestingForComponent(YourComponent, { ngModule: { imports: [...], providers: [...] } });
// For directive
getTestingForDirective(YourDirective, { ngModule: { imports: [...], providers: [...] } });
NOTE: ngModule
prop in second argument is default config for @NgModule
.
Run ng build
to build the project. The build artifacts will be stored in the dist/
directory. Use the --prod
flag for a production build.
Run ng test
to execute the unit tests via Karma.
Run ng e2e
to execute the end-to-end tests via Protractor.
FAQs
Testing utilities for Angular projects
The npm package @orchestrator/ngx-testing receives a total of 1,403 weekly downloads. As such, @orchestrator/ngx-testing popularity was classified as popular.
We found that @orchestrator/ngx-testing demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.