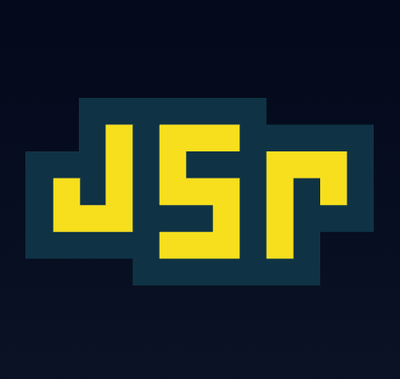
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@ovotech/laminar-cli
Advanced tools
A CLI for the Open Api implementation for the laminar http server.
yarn add @ovotech/laminar-cli
Given a OpenAPI config file:
---
openapi: 3.0.0
info:
title: Test
version: 1.0.0
servers:
- url: http://localhost:3333
paths:
'/test':
post:
requestBody:
required: true
content:
application/json:
schema: { $ref: '#/components/schemas/User' }
responses:
'200':
description: A Test Object
content:
application/json:
schema: { $ref: '#/components/schemas/Test' }
get:
responses:
'200':
description: A Test Object
content:
application/json:
schema: { $ref: '#/components/schemas/Test' }
components:
schemas:
User:
additionalProperties: false
properties:
email:
type: string
scopes:
type: array
items:
type: string
required:
- email
Test:
properties:
text:
type: string
user:
$ref: '#/components/schemas/User'
required:
- text
We can run:
yarn laminar api --file examples/api.yaml --output examples/__generated__/api.yaml.ts
Which would convert a given api.yaml
file to a api.yaml.ts
. Any external urls, referenced in it would be downloaded, and any local file references would be loaded as well.
Then you can load the types like this:
import { HttpService, init, jsonOk } from '@ovotech/laminar';
import { join } from 'path';
import { openApiTyped } from './__generated__/api.yaml';
const main = async () => {
const listener = await openApiTyped({
api: join(__dirname, 'api.yaml'),
paths: {
'/test': {
post: async ({ body }) => jsonOk({ text: 'ok', user: body }),
get: async () => jsonOk({ text: 'ok', user: { email: 'me@example.com' } }),
},
},
});
const server = new HttpService({ listener });
await init({ initOrder: [server], logger: console });
};
main();
You can also watch for changes and regenerate the typescript types with the --watch
flag
yarn laminar --watch --file examples/api.yaml --output examples/__generated__/api.yaml.ts
When you update the source yaml file, or any of the local files it references, laminar
would rebuild the typescript files.
Then given this OpenApi file:
---
openapi: 3.0.0
info:
title: Test
version: 1.0.0
servers:
- url: https://simple.example.com
paths:
'/test/{id}':
parameters:
- name: id
in: path
schema:
type: string
required: true
post:
requestBody:
required: true
content:
application/json:
schema: { $ref: '#/components/schemas/User' }
responses:
'200':
description: A Test Object
content:
application/json:
schema: { $ref: '#/components/schemas/Test' }
get:
responses:
'200':
description: A Test Object
content:
application/json:
schema: { $ref: '#/components/schemas/Test' }
components:
schemas:
User:
additionalProperties: false
properties:
email:
type: string
scopes:
type: array
items:
type: string
required:
- email
Test:
properties:
text:
type: string
user:
$ref: '#/components/schemas/User'
required:
- text
You can run
yarn laminar axios --file examples/axios.yaml --output examples/__generated__/axios.yaml.ts
And would then have:
import { AxiosRequestConfig, AxiosInstance, AxiosResponse } from 'axios';
/**
* Test
*
* Version: 1.0.0
*/
export const axiosOapi = (api: AxiosInstance): AxiosOapiInstance => ({
'POST /test/{id}': (id, data, config) => api.post<Test>(`/test/${id}`, data, config),
'GET /test/{id}': (id, config) => api.get<Test>(`/test/${id}`, config),
api: api,
});
export interface User {
email: string;
scopes?: string[];
}
export interface Test {
text: string;
user?: User;
[key: string]: unknown;
}
export interface AxiosOapiInstance {
'POST /test/{id}': (id: string, data: User, config?: AxiosRequestConfig) => Promise<AxiosResponse<Test>>;
'GET /test/{id}': (id: string, config?: AxiosRequestConfig) => Promise<AxiosResponse<Test>>;
api: AxiosInstance;
}
And you can then use it like this:
import axios from 'axios';
import { axiosOapi } from './__generated__/axios.yaml';
import * as nock from 'nock';
/**
* Mock the simple rest api so we can test it out
*/
nock('http://simple.example.com')
.get('/test/20')
.reply(200, { text: 'test' })
.post('/test/30')
.reply(200, { text: 'test', user: { email: 'test@example.com' } });
/**
* Wrap an axios instance. This will add typed functions with the name of the paths
*/
const simple = axiosOapi(axios.create({ baseURL: 'http://simple.example.com' }));
simple['GET /test/{id}']('20').then(({ data }) => console.log(data));
simple['POST /test/{id}']('30', { email: 'test2example.com' }).then(({ data }) => console.log(data));
If we use swagger's petstore.json example, we can generate the petstore types and use them like this:
import { axiosOapi } from './__generated__/petstore.json';
import axios from 'axios';
const petstore = axiosOapi(axios.create({ baseURL: 'https://petstore.swagger.io/v2' }));
const main = async () => {
const { data: pixel } = await petstore['PUT /pet']({
name: 'Pixel',
photoUrls: ['https://placekitten.com/g/200/300'],
tags: [{ name: 'axios-oapi-cli' }],
});
console.log('SAVED', pixel);
const { data: retrievedPixel } = await petstore['GET /pet/{petId}'](pixel.id!);
console.log('RETRIEVED', retrievedPixel);
// Use the underlying api to perform custom requests
const { data: inventory } = await petstore.api.get('/store/inventory');
console.log('INVENTORY', inventory);
};
main();
If you don't include "output" the cli will output to stdout. You can use this to chain with other processors like prettier
yarn laminar axios --file examples/simple.yaml | prettier --stdin-filepath examples/simple.types.ts > examples/simple.types.ts
If you omit the "file" option, stdin will be used. This can be used to pipe the file contents from somewhere else (like curl). By default it will asume the content to be json.
curl http://example.com/simple.json | yarn laminar axios --output examples/simple.types.ts
You can specify that the openapi content is yaml too:
curl http://example.com/simple.yaml | yarn laminar axios --output examples/simple.types.ts --stdin-type yaml
You can run the tests with:
yarn test
Style is maintained with prettier and eslint
yarn lint
Deployment is preferment by yarn automatically on merge / push to main, but you'll need to bump the package version numbers yourself. Only updated packages with newer versions will be pushed to the npm registry.
Have a bug? File an issue with a simple example that reproduces this so we can take a look & confirm.
Want to make a change? Submit a PR, explain why it's useful, and make sure you've updated the docs (this file) and the tests (see test folder).
This project is licensed under Apache 2 - see the LICENSE file for details
FAQs
CLI generating typescript types for @ovotech/laminar
We found that @ovotech/laminar-cli demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 36 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.