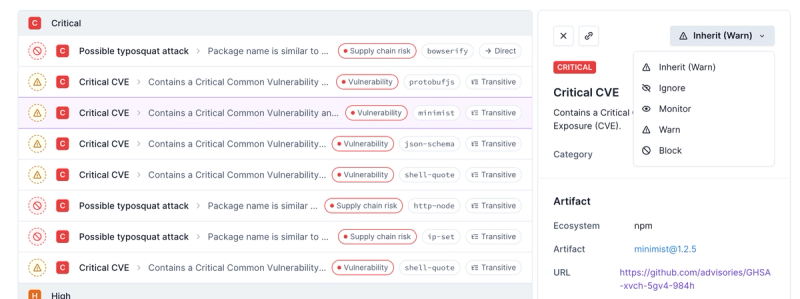
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
@owja/ioc
Advanced tools
Readme
This library implements dependency injection for javascript. It is currently work in progress and not jet released.
No package delivered jet!
npm install --save-dev <unreleased>
Create a folder ioc and add the new file ioc/types.ts:
export const TYPE = {
"MyService" = Symbol.for("MyService"),
"MyOtherService" = Symbol.for("MyOtherService"),
};
Next need a container to bind your dependencies to. Lets create the file ioc/container.ts
import {Container, inject} from <unreleased>;
import {TYPE} from "./types";
import {IMyService, MyService} from "./service/my-service";
import {IMyOtherService, MyOtherService} from "./service/my-other-service";
const container = new Container();
container.bind<IMyService>(TYPE.MyService).to(MyService);
container.bind<IMyOtherService>(TYPE.MyOtherService).to(MyOtherService);
export {container, TYPE, inject};
Lets create a example.ts file in your source root:
import {container, TYPE, inject} from "./ioc/container";
import {IMyService} from "./service/my-service";
class Example {
@inject(TYPE.MyService)
readonly myService!: IMyService;
@inject(TYPE.MyOtherSerice)
readonly myOtherService!: IMyOtherService;
}
const example = new Example();
console.log(example.myService);
console.log(example.myOtherSerice);
If you run this example you should see the content of your example services.
You can snapshot and restore a container for unit testing. You can make multiple snapshots in a row, but that is not recommend.
beforeEach(() => {
container.snapshot();
});
afterEach(() => {
container.restore();
}
test("can snapshot and restore the registry", () => {
container.rebind<MyServiceMock>(TYPE.MySerice).to(MyServiceMock);
// ...
});
I am working on the first release. Current state can you see in the Github Project.
This library is highly inspired by InversifyJS but has other goals:
License under Creative Commons Attribution 4.0 International
Copyright © 2019 Hauke Broer
FAQs
dependency injection for javascript
The npm package @owja/ioc receives a total of 1,275 weekly downloads. As such, @owja/ioc popularity was classified as popular.
We found that @owja/ioc demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.