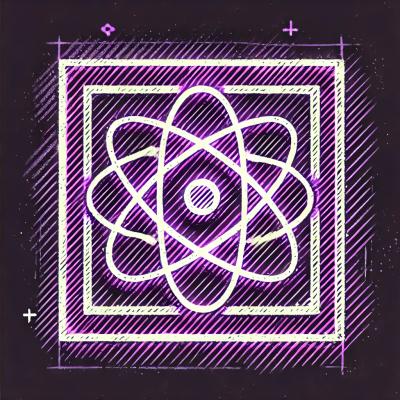
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
@ozdemirburak/morse-code-translator
Advanced tools
Morse code translator helps you convert text to Morse code and vice versa, with the option to play Morse code audio.
Morse code encoder and Morse decoder with no dependencies. It supports Latin, Cyrillic, Greek, Hebrew, Arabic, Persian, Japanese, Korean, and Thai, with audio-generation functionality using the Web Audio API.
$ npm install @ozdemirburak/morse-code-translator --save
import morse from '@ozdemirburak/morse-code-translator';
const encoded = morse.encode('SOS'); // ... --- ...
const decoded = morse.decode('... --- ...'); // SOS
const characters = morse.characters(); // {'1': {'A': '.-', ...}, ..., '11': {'ㄱ': '.-..', ...}}
const audio = morse.audio('SOS');
audio.play(); // play audio
audio.stop(); // stop audio (cannot resume)
audio.exportWave(); // download audio wave file (promise)
const url = await audio.getWaveUrl(); // get audio wave url (promise)
const blob = await audio.getWaveBlob(); // get audio wave blob (promise)
You can customize the dash, dot, or space characters and specify the alphabet with the priority option for an accurate encoding and decoding.
The priority option gives direction to the plugin to start searching for the given character set first.
Set the priority option according to the list below.
const cyrillic = morse.encode('Ленинград', { priority: 5 }); // .-.. . -. .. -. --. .-. .- -..
const greek = morse.decode('... .- --. .- .--. .--', { priority: 6 }); // ΣΑΓΑΠΩ
const hebrew = morse.decode('.. ––– . –––', { dash: '–', dot: '.', priority: 7 }); // יהוה
const japanese = morse.encode('NEWS', { priority: 10, dash: '-', dot: '・', separator: ' ' }); // -・ ・ ・-- ・・・
const characters = morse.characters({ dash: '–', dot: '•' }); // {'1': {'A': '•–', ...}, ..., '11': {'ㄱ': '•–••', ...}}
const arabicAudio = morse.audio('البراق', { // generates the Morse .- .-.. -... .-. .- --.- then generates the audio from it
wpm: 18, // sets the wpm based on the Paris method, note that setting this will override and set the unit and fwUnits accordingly
unit: 0.1, // period of one unit, in seconds, 1.2 / c where c is speed of transmission, in words per minute
fwUnit: 0.1, // period of one Farnsworth unit to control intercharacter and interword gaps
volume: 100, // the volume in percent (0-100)
oscillator: {
type: 'sine', // sine, square, sawtooth, triangle
frequency: 500, // value in hertz
onended: function () { // event that fires when the tone stops playing
console.log('ended');
}
}
});
const oscillator = arabicAudio.oscillator; // OscillatorNode
const context = arabicAudio.context; // AudioContext;
const gainNode = arabicAudio.gainNode; // GainNode
arabicAudio.play(); // will start playing Morse audio
arabicAudio.stop(); // will stop playing Morse audio
morse-code-translator has been developed with extensive feedback and contributions from numerous developers.
Special thanks to Chris Jones, who added many great features.
Please consider referencing the website of this project, if you find this package useful for your work.
<a href="https://morsecodetranslator.com">Morse Code Translator</a>
The MIT License (MIT). Please see License File for more information.
FAQs
Morse code translator helps you convert text to Morse code and vice versa, with the option to play Morse code audio.
The npm package @ozdemirburak/morse-code-translator receives a total of 35 weekly downloads. As such, @ozdemirburak/morse-code-translator popularity was classified as not popular.
We found that @ozdemirburak/morse-code-translator demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.