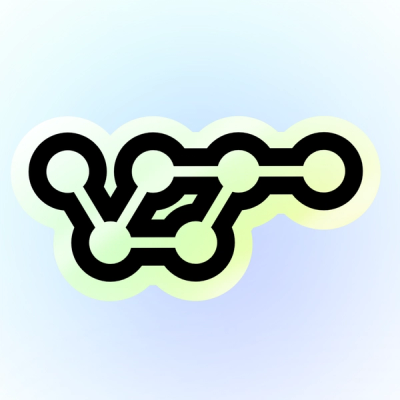
Security News
vlt Debuts New JavaScript Package Manager and Serverless Registry at NodeConf EU
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
@panora/sdk-typescript
Advanced tools
The Typescript SDK for PanoraSDK.
The Panora API description
npm install @panora/sdk-typescript
To see whether an endpoint needs a specific type of authentication check the endpoint's documentation.
The PanoraSDK API uses access tokens as a form of authentication. You can set the access token when initializing the SDK through the constructor:
const sdk = new PanoraSDK('YOUR_ACCESS_TOKEN')
Or through the setAccessToken
method:
const sdk = new PanoraSDK()
sdk.setAccessToken('YOUR_ACCESS_TOKEN')
You can also set it for each service individually:
const sdk = new PanoraSDK()
sdk.main.setAccessToken('YOUR_ACCESS_TOKEN')
Here is a simple program demonstrating usage of this SDK. It can also be found in the examples/src/index.ts
file in this directory.
When running the sample make sure to use npm install
to install all the dependencies.
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.main
.getHello();
console.log(result);
})();
A list of all services and services methods.
Services
Method | Description |
---|---|
getHello |
Method | Description |
---|---|
getHealth |
Method | Description |
---|---|
getHelloProtected |
Method | Description |
---|---|
signUp | Register |
signIn | Log In |
getUsers | Get users |
getApiKeys | Retrieve API Keys |
generateApiKey | Create API Key |
Method | Description |
---|---|
handleOAuthCallback | Capture oAuth callback |
getConnections | List Connections |
Method | Description |
---|---|
createWebhookMetadata | Add webhook metadata |
getWebhooksMetadata | Retrieve webhooks metadata |
updateWebhookStatus | Update webhook status |
Method | Description |
---|---|
addLinkedUser | Add Linked User |
getLinkedUsers | Retrieve Linked Users |
getLinkedUser | Retrieve a Linked User |
Method | Description |
---|---|
getOrganisations | Retrieve Organisations |
createOrganisation | Create an Organisation |
Method | Description |
---|---|
getProjects | Retrieve projects |
createProject | Create a project |
Method | Description |
---|---|
getFieldMappingsEntities | Retrieve field mapping entities |
getFieldMappings | Retrieve field mappings |
getFieldMappingValues | Retrieve field mappings values |
defineTargetField | Define target Field |
mapField | Map Custom Field |
getCustomProviderProperties | Retrieve Custom Properties |
Method | Description |
---|---|
getEvents | Retrieve Events |
Method | Description |
---|---|
createMagicLink | Create a Magic Link |
getMagicLinks | Retrieve Magic Links |
getMagicLink | Retrieve a Magic Link |
Method | Description |
---|---|
passthroughRequest | Make a passthrough request |
Method | Description |
---|---|
addContact | Create CRM Contact |
getContacts | List a batch of CRM Contacts |
updateContact | Update a CRM Contact |
getContact | Retrieve a CRM Contact |
addContacts | Add a batch of CRM Contacts |
Method | Description |
---|---|
addDeal | Create a Deal |
getDeals | List a batch of Deals |
getDeal | Retrieve a Deal |
updateDeal | Update a Deal |
addDeals | Add a batch of Deals |
Method | Description |
---|---|
addNote | Create a Note |
getNotes | List a batch of Notes |
getNote | Retrieve a Note |
addNotes | Add a batch of Notes |
Method | Description |
---|---|
addCompany | Create a Company |
getCompanies | List a batch of Companies |
updateCompany | Update a Company |
getCompany | Retrieve a Company |
addCompanies | Add a batch of Companies |
Method | Description |
---|---|
addEngagement | Create a Engagement |
getEngagements | List a batch of Engagements |
updateEngagement | Update a Engagement |
getEngagement | Retrieve a Engagement |
addEngagements | Add a batch of Engagements |
Method | Description |
---|---|
getStages | List a batch of Stages |
getStage | Retrieve a Stage |
Method | Description |
---|---|
addTask | Create a Task |
getTasks | List a batch of Tasks |
updateTask | Update a Task |
getTask | Retrieve a Task |
addTasks | Add a batch of Tasks |
Method | Description |
---|---|
getUsers | List a batch of Users |
getUser | Retrieve a User |
Method | Description |
---|---|
addTicket | Create a Ticket |
getTickets | List a batch of Tickets |
updateTicket | Update a Ticket |
getTicket | Retrieve a Ticket |
addTickets | Add a batch of Tickets |
Method | Description |
---|---|
addComment | Create a Comment |
getComments | List a batch of Comments |
getComment | Retrieve a Comment |
addComments | Add a batch of Comments |
Method | Description |
---|---|
getUsers | List a batch of Users |
getUser | Retrieve a User |
Method | Description |
---|---|
addAttachment | Create a Attachment |
getAttachments | List a batch of Attachments |
getAttachment | Retrieve a Attachment |
downloadAttachment | Download a Attachment |
addAttachments | Add a batch of Attachments |
Method | Description |
---|---|
getContacts | List a batch of Contacts |
getContact | Retrieve a Contact |
Method | Description |
---|---|
getAccounts | List a batch of Accounts |
getAccount | Retrieve an Account |
Method | Description |
---|---|
getTags | List a batch of Tags |
getTag | Retrieve a Tag |
Method | Description |
---|---|
getTeams | List a batch of Teams |
getTeam | Retrieve a Team |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.main.getHello();
console.log(result);
})();
Return Type
Returns a dict object.
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.health.getHealth();
console.log(result);
})();
Return Type
Returns a dict object.
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.protected.getHelloProtected();
console.log(result);
})();
Register
Required Parameters
| input | object | Request body. |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const input = {
email: 'email',
first_name: 'first_name',
id_organisation: 'id_organisation',
last_name: 'last_name',
password_hash: 'password_hash',
};
const result = await sdk.auth.signUp(input);
console.log(result);
})();
Log In
Required Parameters
| input | object | Request body. |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const input = { email: 'email', id_user: 'id_user', password_hash: 'password_hash' };
const result = await sdk.auth.signIn(input);
console.log(result);
})();
Get users
Return Type
Returns a dict object.
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.auth.getUsers();
console.log(result);
})();
Retrieve API Keys
Return Type
Returns a dict object.
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.auth.getApiKeys();
console.log(result);
})();
Create API Key
Required Parameters
| input | object | Request body. |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const input = { keyName: 'keyName', projectId: 'projectId', userId: 'userId' };
const result = await sdk.auth.generateApiKey(input);
console.log(result);
})();
Capture oAuth callback
Required Parameters
Name | Type | Description |
---|---|---|
state | string | |
code | string | |
location | string |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.connections.handleOAuthCallback('state', 'code', 'location');
console.log(result);
})();
List Connections
Return Type
Returns a dict object.
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.connections.getConnections();
console.log(result);
})();
Add webhook metadata
Required Parameters
| input | object | Request body. |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const input = {
description: 'description',
id_project: 'id_project',
scope: ['sit cupidatat ut', 'magna reprehenderit'],
url: 'url',
};
const result = await sdk.webhook.createWebhookMetadata(input);
console.log(result);
})();
Retrieve webhooks metadata
Return Type
Returns a dict object.
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.webhook.getWebhooksMetadata();
console.log(result);
})();
Update webhook status
Required Parameters
Name | Type | Description |
---|---|---|
id | string |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.webhook.updateWebhookStatus('id');
console.log(result);
})();
Add Linked User
Required Parameters
| input | object | Request body. |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const input = {
alias: 'alias',
id_project: 'id_project',
linked_user_origin_id: 'linked_user_origin_id',
};
const result = await sdk.linkedUsers.addLinkedUser(input);
console.log(result);
})();
Retrieve Linked Users
Return Type
Returns a dict object.
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.linkedUsers.getLinkedUsers();
console.log(result);
})();
Retrieve a Linked User
Required Parameters
Name | Type | Description |
---|---|---|
originId | string |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.linkedUsers.getLinkedUser('originId');
console.log(result);
})();
Retrieve Organisations
Return Type
Returns a dict object.
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.organisations.getOrganisations();
console.log(result);
})();
Create an Organisation
Required Parameters
| input | object | Request body. |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const input = { name: 'name', stripe_customer_id: 'stripe_customer_id' };
const result = await sdk.organisations.createOrganisation(input);
console.log(result);
})();
Retrieve projects
Return Type
Returns a dict object.
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.projects.getProjects();
console.log(result);
})();
Create a project
Required Parameters
| input | object | Request body. |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const input = { id_organization: 'id_organization', name: 'name' };
const result = await sdk.projects.createProject(input);
console.log(result);
})();
Retrieve field mapping entities
Return Type
Returns a dict object.
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.fieldMapping.getFieldMappingsEntities();
console.log(result);
})();
Retrieve field mappings
Return Type
Returns a dict object.
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.fieldMapping.getFieldMappings();
console.log(result);
})();
Retrieve field mappings values
Return Type
Returns a dict object.
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.fieldMapping.getFieldMappingValues();
console.log(result);
})();
Define target Field
Required Parameters
| input | object | Request body. |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const input = {
data_type: 'data_type',
description: 'description',
name: 'name',
object_type_owner: 'object_type_owner',
};
const result = await sdk.fieldMapping.defineTargetField(input);
console.log(result);
})();
Map Custom Field
Required Parameters
| input | object | Request body. |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const input = {
attributeId: 'attributeId',
linked_user_id: 'linked_user_id',
source_custom_field_id: 'source_custom_field_id',
source_provider: 'source_provider',
};
const result = await sdk.fieldMapping.mapField(input);
console.log(result);
})();
Retrieve Custom Properties
Required Parameters
Name | Type | Description |
---|---|---|
linkedUserId | string | |
providerId | string |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.fieldMapping.getCustomProviderProperties('linkedUserId', 'providerId');
console.log(result);
})();
Retrieve Events
Return Type
Returns a dict object.
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.events.getEvents();
console.log(result);
})();
Create a Magic Link
Required Parameters
| input | object | Request body. |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const input = {
alias: 'alias',
email: 'email',
id_project: 'id_project',
linked_user_origin_id: 'linked_user_origin_id',
};
const result = await sdk.magicLink.createMagicLink(input);
console.log(result);
})();
Retrieve Magic Links
Return Type
Returns a dict object.
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.magicLink.getMagicLinks();
console.log(result);
})();
Retrieve a Magic Link
Required Parameters
Name | Type | Description |
---|---|---|
id | string |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.magicLink.getMagicLink('id');
console.log(result);
})();
Make a passthrough request
Required Parameters
Name | Type | Description |
---|---|---|
integrationId | string | |
linkedUserId | string | |
input | object | Request body. |
Return Type
PassThroughResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const input = { data: {}, headers_: {}, method: 'PATCH', path: 'path' };
const result = await sdk.passthrough.passthroughRequest(input, 'integrationId', 'linkedUserId');
console.log(result);
})();
Create CRM Contact
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
input | object | Request body. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original CRM software. |
Return Type
AddContactResponse UnifiedContactOutput
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const input = {
addresses: [],
email_addresses: [],
field_mappings: {},
first_name: 'first_name',
last_name: 'last_name',
phone_numbers: [],
user_id: 'user_id',
};
const result = await sdk.crmContacts.addContact(input, 'connection_token', { remoteData: true });
console.log(result);
})();
List a batch of CRM Contacts
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original CRM software. |
Return Type
GetContactsResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.crmContacts.getContacts('connection_token', { remoteData: true });
console.log(result);
})();
Update a CRM Contact
Required Parameters
Name | Type | Description |
---|---|---|
id | string |
Return Type
UnifiedContactOutput
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.crmContacts.updateContact('id');
console.log(result);
})();
Retrieve a CRM Contact
Required Parameters
Name | Type | Description |
---|---|---|
id | string | id of the contact you want to retrive. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original CRM software. |
Return Type
GetContactResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.crmContacts.getContact('id', { remoteData: true });
console.log(result);
})();
Add a batch of CRM Contacts
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
input | object | Request body. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original CRM software. |
Return Type
AddContactsResponse CrmContactsAddContactsResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const input = [{}, {}];
const result = await sdk.crmContacts.addContacts(input, 'connection_token', { remoteData: true });
console.log(result);
})();
Create a Deal
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
input | object | Request body. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Crm software. |
Return Type
AddDealResponse UnifiedDealOutput
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const input = { field_mappings: {} };
const result = await sdk.crmDeals.addDeal(input, 'connection_token', { remoteData: true });
console.log(result);
})();
List a batch of Deals
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Crm software. |
Return Type
GetDealsResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.crmDeals.getDeals('connection_token', { remoteData: true });
console.log(result);
})();
Retrieve a Deal
Required Parameters
Name | Type | Description |
---|---|---|
id | string | id of the deal you want to retrieve. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Crm software. |
Return Type
GetDealResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.crmDeals.getDeal('id', { remoteData: true });
console.log(result);
})();
Update a Deal
Required Parameters
Name | Type | Description |
---|---|---|
id | string |
Return Type
UpdateDealResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.crmDeals.updateDeal('id');
console.log(result);
})();
Add a batch of Deals
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
input | object | Request body. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Crm software. |
Return Type
AddDealsResponse CrmDealsAddDealsResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const input = [{}, {}];
const result = await sdk.crmDeals.addDeals(input, 'connection_token', { remoteData: true });
console.log(result);
})();
Create a Note
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
input | object | Request body. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Crm software. |
Return Type
AddNoteResponse UnifiedNoteOutput
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const input = { field_mappings: {} };
const result = await sdk.crmNotes.addNote(input, 'connection_token', { remoteData: true });
console.log(result);
})();
List a batch of Notes
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Crm software. |
Return Type
GetNotesResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.crmNotes.getNotes('connection_token', { remoteData: true });
console.log(result);
})();
Retrieve a Note
Required Parameters
Name | Type | Description |
---|---|---|
id | string | id of the note you want to retrieve. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Crm software. |
Return Type
GetNoteResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.crmNotes.getNote('id', { remoteData: true });
console.log(result);
})();
Add a batch of Notes
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
input | object | Request body. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Crm software. |
Return Type
AddNotesResponse CrmNotesAddNotesResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const input = [{}, {}];
const result = await sdk.crmNotes.addNotes(input, 'connection_token', { remoteData: true });
console.log(result);
})();
Create a Company
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
input | object | Request body. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Crm software. |
Return Type
AddCompanyResponse UnifiedCompanyOutput
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const input = { field_mappings: {} };
const result = await sdk.crmCompanies.addCompany(input, 'connection_token', { remoteData: true });
console.log(result);
})();
List a batch of Companies
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Crm software. |
Return Type
GetCompaniesResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.crmCompanies.getCompanies('connection_token', { remoteData: true });
console.log(result);
})();
Update a Company
Required Parameters
Name | Type | Description |
---|---|---|
id | string |
Return Type
UpdateCompanyResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.crmCompanies.updateCompany('id');
console.log(result);
})();
Retrieve a Company
Required Parameters
Name | Type | Description |
---|---|---|
id | string | id of the company you want to retrieve. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Crm software. |
Return Type
GetCompanyResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.crmCompanies.getCompany('id', { remoteData: true });
console.log(result);
})();
Add a batch of Companies
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
input | object | Request body. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Crm software. |
Return Type
AddCompaniesResponse CrmCompaniesAddCompaniesResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const input = [{}, {}];
const result = await sdk.crmCompanies.addCompanies(input, 'connection_token', {
remoteData: true,
});
console.log(result);
})();
Create a Engagement
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
input | object | Request body. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Crm software. |
Return Type
AddEngagementResponse UnifiedEngagementOutput
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const input = { field_mappings: {} };
const result = await sdk.crmEngagements.addEngagement(input, 'connection_token', {
remoteData: true,
});
console.log(result);
})();
List a batch of Engagements
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Crm software. |
Return Type
GetEngagementsResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.crmEngagements.getEngagements('connection_token', { remoteData: true });
console.log(result);
})();
Update a Engagement
Required Parameters
Name | Type | Description |
---|---|---|
id | string |
Return Type
UpdateEngagementResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.crmEngagements.updateEngagement('id');
console.log(result);
})();
Retrieve a Engagement
Required Parameters
Name | Type | Description |
---|---|---|
id | string | id of the engagement you want to retrieve. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Crm software. |
Return Type
GetEngagementResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.crmEngagements.getEngagement('id', { remoteData: true });
console.log(result);
})();
Add a batch of Engagements
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
input | object | Request body. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Crm software. |
Return Type
AddEngagementsResponse CrmEngagementsAddEngagementsResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const input = [{}, {}];
const result = await sdk.crmEngagements.addEngagements(input, 'connection_token', {
remoteData: true,
});
console.log(result);
})();
List a batch of Stages
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Crm software. |
Return Type
GetStagesResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.crmStages.getStages('connection_token', { remoteData: true });
console.log(result);
})();
Retrieve a Stage
Required Parameters
Name | Type | Description |
---|---|---|
id | string | id of the stage you want to retrieve. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Crm software. |
Return Type
GetStageResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.crmStages.getStage('id', { remoteData: true });
console.log(result);
})();
Create a Task
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
input | object | Request body. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Crm software. |
Return Type
AddTaskResponse UnifiedTaskOutput
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const input = { field_mappings: {} };
const result = await sdk.crmTasks.addTask(input, 'connection_token', { remoteData: true });
console.log(result);
})();
List a batch of Tasks
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Crm software. |
Return Type
GetTasksResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.crmTasks.getTasks('connection_token', { remoteData: true });
console.log(result);
})();
Update a Task
Required Parameters
Name | Type | Description |
---|---|---|
id | string |
Return Type
UpdateTaskResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.crmTasks.updateTask('id');
console.log(result);
})();
Retrieve a Task
Required Parameters
Name | Type | Description |
---|---|---|
id | string | id of the task you want to retrieve. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Crm software. |
Return Type
GetTaskResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.crmTasks.getTask('id', { remoteData: true });
console.log(result);
})();
Add a batch of Tasks
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
input | object | Request body. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Crm software. |
Return Type
AddTasksResponse CrmTasksAddTasksResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const input = [{}, {}];
const result = await sdk.crmTasks.addTasks(input, 'connection_token', { remoteData: true });
console.log(result);
})();
List a batch of Users
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Crm software. |
Return Type
GetUsersResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.crmUsers.getUsers('connection_token', { remoteData: true });
console.log(result);
})();
Retrieve a User
Required Parameters
Name | Type | Description |
---|---|---|
id | string | id of the user you want to retrieve. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Crm software. |
Return Type
GetUserResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.crmUsers.getUser('id', { remoteData: true });
console.log(result);
})();
Create a Ticket
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
input | object | Request body. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Ticketing software. |
Return Type
AddTicketResponse UnifiedTicketOutput
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const input = {
account_id: 'account_id',
assigned_to: ['aliquip', 'enim'],
comment: ['pariatur nulla deserunt', 'irure incididunt Duis minim'],
completed_at: '1912-09-29T15:29:41.0Z',
contact_id: 'contact_id',
description: ['Duis nostrud in', 'magna adipisicing'],
due_date: '1945-12-08T15:08:52.0Z',
field_mappings: {},
name: 'name',
parent_ticket: 'parent_ticket',
priority: 'priority',
status: 'status',
tags: ['cillum', 'officia dolore'],
type_: 'type',
};
const result = await sdk.ticketingTickets.addTicket(input, 'connection_token', {
remoteData: true,
});
console.log(result);
})();
List a batch of Tickets
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Ticketing software. |
Return Type
GetTicketsResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.ticketingTickets.getTickets('connection_token', { remoteData: true });
console.log(result);
})();
Update a Ticket
Required Parameters
Name | Type | Description |
---|---|---|
id | string |
Return Type
UnifiedTicketOutput
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.ticketingTickets.updateTicket('id');
console.log(result);
})();
Retrieve a Ticket
Required Parameters
Name | Type | Description |
---|---|---|
id | string | id of the ticket you want to retrive. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Ticketing software. |
Return Type
GetTicketResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.ticketingTickets.getTicket('id', { remoteData: true });
console.log(result);
})();
Add a batch of Tickets
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
input | object | Request body. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Ticketing software. |
Return Type
AddTicketsResponse TicketingTicketsAddTicketsResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const input = [{}, {}];
const result = await sdk.ticketingTickets.addTickets(input, 'connection_token', {
remoteData: true,
});
console.log(result);
})();
Create a Comment
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
input | object | Request body. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Ticketing software. |
Return Type
AddCommentResponse UnifiedCommentOutput
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const input = {
attachments: ['cillum sint dolor anim magna', 'labore mollit magna elit'],
body: 'body',
contact_id: 'contact_id',
creator_type: 'creator_type',
html_body: 'html_body',
is_private: true,
ticket_id: 'ticket_id',
user_id: 'user_id',
};
const result = await sdk.ticketingComments.addComment(input, 'connection_token', {
remoteData: true,
});
console.log(result);
})();
List a batch of Comments
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Ticketing software. |
Return Type
GetCommentsResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.ticketingComments.getComments('connection_token', { remoteData: true });
console.log(result);
})();
Retrieve a Comment
Required Parameters
Name | Type | Description |
---|---|---|
id | string | id of the comment you want to retrive. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Ticketing software. |
Return Type
GetCommentResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.ticketingComments.getComment('id', { remoteData: true });
console.log(result);
})();
Add a batch of Comments
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
input | object | Request body. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Ticketing software. |
Return Type
AddCommentsResponse TicketingCommentsAddCommentsResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const input = [{}, {}];
const result = await sdk.ticketingComments.addComments(input, 'connection_token', {
remoteData: true,
});
console.log(result);
})();
List a batch of Users
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Ticketing software. |
Return Type
GetUsersResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.ticketingUsers.getUsers('connection_token', { remoteData: true });
console.log(result);
})();
Retrieve a User
Required Parameters
Name | Type | Description |
---|---|---|
id | string | id of the user you want to retrieve. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Ticketing software. |
Return Type
GetUserResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.ticketingUsers.getUser('id', { remoteData: true });
console.log(result);
})();
Create a Attachment
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
input | object | Request body. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Ticketing software. |
Return Type
AddAttachmentResponse UnifiedAttachmentOutput
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const input = {
field_mappings: {},
file_name: 'file_name',
file_url: 'file_url',
uploader: 'uploader',
};
const result = await sdk.ticketingAttachments.addAttachment(input, 'connection_token', {
remoteData: true,
});
console.log(result);
})();
List a batch of Attachments
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Ticketing software. |
Return Type
GetAttachmentsResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.ticketingAttachments.getAttachments('connection_token', {
remoteData: true,
});
console.log(result);
})();
Retrieve a Attachment
Required Parameters
Name | Type | Description |
---|---|---|
id | string | id of the attachment you want to retrive. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Ticketing software. |
Return Type
GetAttachmentResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.ticketingAttachments.getAttachment('id', { remoteData: true });
console.log(result);
})();
Download a Attachment
Required Parameters
Name | Type | Description |
---|---|---|
id | string | id of the attachment you want to retrive. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Ticketing software. |
Return Type
DownloadAttachmentResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.ticketingAttachments.downloadAttachment('id', { remoteData: true });
console.log(result);
})();
Add a batch of Attachments
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
input | object | Request body. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Ticketing software. |
Return Type
AddAttachmentsResponse TicketingAttachmentsAddAttachmentsResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const input = [{}, {}];
const result = await sdk.ticketingAttachments.addAttachments(input, 'connection_token', {
remoteData: true,
});
console.log(result);
})();
List a batch of Contacts
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Ticketing software. |
Return Type
GetContactsResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.ticketingContacts.getContacts('connection_token', { remoteData: true });
console.log(result);
})();
Retrieve a Contact
Required Parameters
Name | Type | Description |
---|---|---|
id | string | id of the contact you want to retrieve. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Ticketing software. |
Return Type
GetContactResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.ticketingContacts.getContact('id', { remoteData: true });
console.log(result);
})();
List a batch of Accounts
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Ticketing software. |
Return Type
GetAccountsResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.ticketingAccounts.getAccounts('connection_token', { remoteData: true });
console.log(result);
})();
Retrieve an Account
Required Parameters
Name | Type | Description |
---|---|---|
id | string | id of the account you want to retrieve. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Ticketing software. |
Return Type
GetAccountResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.ticketingAccounts.getAccount('id', { remoteData: true });
console.log(result);
})();
List a batch of Tags
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Ticketing software. |
Return Type
GetTagsResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.ticketingTags.getTags('connection_token', { remoteData: true });
console.log(result);
})();
Retrieve a Tag
Required Parameters
Name | Type | Description |
---|---|---|
id | string | id of the tag you want to retrieve. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Ticketing software. |
Return Type
GetTagResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.ticketingTags.getTag('id', { remoteData: true });
console.log(result);
})();
List a batch of Teams
Required Parameters
Name | Type | Description |
---|---|---|
connectionToken | string | The connection token |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Ticketing software. |
Return Type
GetTeamsResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.ticketingTeams.getTeams('connection_token', { remoteData: true });
console.log(result);
})();
Retrieve a Team
Required Parameters
Name | Type | Description |
---|---|---|
id | string | id of the team you want to retrieve. |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
remoteData | boolean | Set to true to include data from the original Ticketing software. |
Return Type
GetTeamResponse
Example Usage Code Snippet
import { PanoraSDK } from '@panora/sdk-typescript';
const sdk = new PanoraSDK({ accessToken: process.env.PANORASDK_ACCESS_TOKEN });
(async () => {
const result = await sdk.ticketingTeams.getTeam('id', { remoteData: true });
console.log(result);
})();
License: MIT. See license in LICENSE.
FAQs
The Panora SDK description
The npm package @panora/sdk-typescript receives a total of 0 weekly downloads. As such, @panora/sdk-typescript popularity was classified as not popular.
We found that @panora/sdk-typescript demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Security News
Research
The Socket Research Team uncovered a malicious Python package typosquatting the popular 'fabric' SSH library, silently exfiltrating AWS credentials from unsuspecting developers.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.