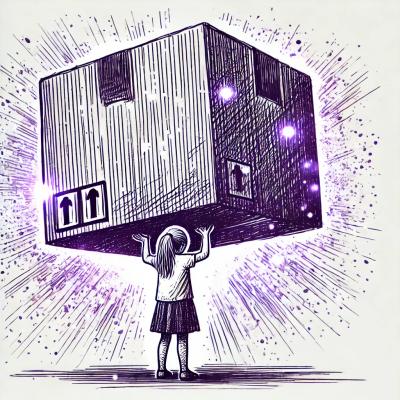
Security News
The Unpaid Backbone of Open Source: Solo Maintainers Face Increasing Security Demands
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
@paystack/checkout-js
Advanced tools
Checkout JS is a billing library for building web checkout flows on Paystack.
npm install @paystack/checkout-js --save
or just grab the js file and add it to your html directly
<script src="https://js.paystack.co/v1/checkout.js"></script>
import { Transaction } from 'checkout-js';
async function chargeCustomerCard() {
const transactionData = {
email: 'test@paystack.com', // replace this with customer email
amount: 100,
key: 'your_paystack_public_key',
};
try {
const transaction = await Transaction.request(transactionData);
await transaction.setCard({
number: '4084084084084081',
cvv: '408',
month: '01',
year: '20',
pin: '1234',
});
// charge resolves to a ChargeResponse
const chargeResponse = await transaction.chargeCard();
if (chargeResponse.status === 'success') {
console.log('card was charged successfully!');
}
} catch (error) {
console.log(error);
}
}
chargeCustomerCard();
Add the Checkout JS script to your HTML
document
<script src="https://js.paystack.co/v1/checkout.js"></script>
And then access the available classes in your JavaScript file;
var transactionData = {
email: 'test@paystack.com', // replace this with customer email
amount: 100,
key: 'your_paystack_public_key',
};
var customerCardDetails = {
number: '4084084084084081',
cvv: '408',
month: '01',
year: '20',
pin: '1234',
};
function chargeCustomerCard() {
Transaction.request(transactionData).then(function(createdTransaction) {
createdTransaction.setCard(customerCardDetails);
return createdTransaction.chargeCard();
}).then(function(chargeResponse) {
if (chargeResponse.status === 'success') {
console.log('card was charged successfully!');
}
}).catch(function(error) {
console.log(error);
});
}
chargeCustomerCard();
These are the classes exported by checkout js
:
The Transaction
class is the most important class in Checkout JS. All the functions required for initializing a transaction, updating it, and charging a customer using the supported payment channels are bound to this class.
For example, charging a customer's card could entail the following steps;
This is a grouping of the methods exported by the Transaction
class:
Transaction.request
Transaction.requestWithAccessCode
Transaction.validate
Transaction.setCard
Transaction.chargeCard
Transaction.authenticateCard
Transaction.listenFor3DSCharge
Transaction.setBankAccount
Transaction.chargeBankAccount
Transaction.registerBankAccount
Transaction.authenticateBankAccount
Transaction.getIbankUrl
Transaction.listenForIbankCharge
Transaction.chargeDigitalBankMandate
Transaction.listenForDigitalBankMandateCharge
Transaction.getUSSDShortcode
Transaction.listenForUSSDCharge
Transaction.getQRCode
Transaction.listenForQRCharge
Transaction.chargeMobileMoney
Transaction.listenForMobileMoneyCharge
Transaction.initializeLog
Transaction.getTimeSpent
Transaction.logPageOpen
Transaction.logChannelSwitch
Transaction.logPageClose
Transaction.logInput
Transaction.logValidationErrors
Transaction.logAuthWindowOpen
Transaction.logAuthWindowClose
Transaction.logAPIResponse
Transaction.logAttempt
Transaction.saveReferrer
Transaction.requery
Utility classes:
This method returns a new transaction instance. The instance returned is what is used to complete the customer’s payment.
Base parameters for this method are:
Parameter | Required | Type | Description |
---|---|---|---|
True | String | The customer's email address | |
amount | True | String | Number |
key | True | String | Your Paystack public key. You can find this on your dashboard in Settings > API Keys & Webhooks |
reference | False | String | Unique case sensitive transaction reference. Only - ,. , = and alphanumeric characters allowed |
channels | False | Array | An array of payment channels to use. Defaults to all available channels on an integration |
currency | False | String | The currency of the transaction. Default is NGN |
amount | True | String | Amount in smallest currency unit (kobo/pesewa/cents). Ignored if creating a subscription |
firstName | False | String | The first name of the customer |
lastName | False | String | The last name of the customer |
phone | False | String | The phone number of the customer |
metadata | False | Object | A valid object of extra information that you want to be saved to the transaction. |
cusotmer_code | False | String | The customer code for the customer on Paystack |
Other optional parameters for Split Payments and Recurring Charges
Parameter | Required | Type | Description |
---|---|---|---|
plan | False | String | If transaction is to create a subscription to a predefined plan, provide plan code here |
subaccountCode | False | String | A valid Paystack subaccount code e.g. ACCT_8f4s1eq7ml6rlzj |
split_code | False | String | A valid Paystack split code e.g. SPL_qQsdYLXddd |
bearer | False | Number | Who bears Paystack charges? account or subaccount (defaults to account ). |
transactionCharge | False | String | A flat fee (in kobo) to charge the subaccount for this transaction. This overrides the split percentage set when the subaccount was created. |
planInterval | False | String | Interval for the plan. Valid intervals are hourly , daily , weekly , monthly , annually |
subscriptionStartDate | False | String | The start date for the subscription (after the first charge) |
planCode | False | String | A valid Paystack plan code e.g. PLN_cujsmvoyq2209ws |
subscriptionCount | False | Number | The number of subscriptions to create for this plan |
subscriptionLimit | False | Number | The number of times to charge for this subscription |
Example
import { Transaction } from 'checkout-js';
interface Parameters {
key: string;
email: string;
amount: number;
ref?: string;
customer_code?: string;
plan?: string;
transaction_charge?: string;
bearer?: string;
channels?: string[];
currency?: string;
firstname?: string;
lastname?: string;
phone?: string;
remark?: string;
payment_page?: string;
payment_request?: string;
quantity?: string;
coupon?: string;
start_date?: string;
interval?: string;
invoice_limit?: number;
subaccount?: string;
hash?: string;
metadata?: string;
}
const transactionData: Parameters = {
email: 'test@paystack.com', // replace this with the customer's email
amount: 100,
key: 'your_paystack_public_key',
};
// returns an instance of the Transaction class
const transaction = await Transaction.request(transactionData);
While the Transaction.request
method lets you create transactions from scratch in the browser, Transaction.requestWithAccessCode
lets you complete a transaction initialized from your server on the frontend. This method can be more secure and it, ensures no new transactions are created on the frontend.
Example
import { Transaction } from 'checkout-js';
const accessCode = 'ltxo947mrhrbz6z'; // Access code for transaction created on your server using the Paystack API
// returns an instance of the transaction class
const transaction = await Transaction.requestWithAccessCode(accessCode);
This is a utility method used to validate the parameters for requesting a new transaction.
Example
import { Transaction } from 'checkout-js';
const transactionData = {
email: 'test@paystack.com', // replace this with the customer's email
amount: 100,
key: 'your_paystack_public_key',
};
try {
Transaction.validate(transactionData); // throws validation errors
} catch (error) {
// handle validation errors thrown by Transaction.validate
}
NB: Transaction.request
calls this validation function implicitly, so you do not need to call it yourself except if you need to handle parameter validation separately.
For each attempt to charge a payment instrument, checkout-js
responds with a ChargeResponse
. This ChargeResponse
has the following interface depending on the kind of response received:
interface ChargeResponse {
status: string;
message: string;
data?: Object
errors?: Array;
}
reference will be made to this ChargeResponse
throughout the rest of the documentation. More details here
Charging a card includes the following steps:
This is an instance method on transactions to set a card to be charged for the transaction. With this, you can
Example
import { Transaction } from 'checkout-js';
const transactionData = { ... };
const transaction = await Transaction.request(transactionData)
interface Card {
number: string;
cvv: string;
month: string;
year: string;
}
/**
* Card number can have varying lengths, typically from 16 to 19 characters
* CVV can be 3 or 4 characters in length
*/
const newCard = {
number: '4084084084084081',
cvv: '408',
month: '01',
year: '20'
}
await transaction.setCard(newCard);
After setting a card on a transaction, you can now attempt to charge it by calling chargeCard()
Example
import { Transaction } from 'checkout-js';
...
const chargeResponse = await transaction.chargeCard();
If chargeResponse
returns a response with status ===
'auth'
you can request the customer's phone number or the OTP sent to their phone number. data.auth
on the chargeResponse
states what type of authentication is required.
Example
import { Transaction } from 'checkout-js';
const newCard = {
number: '4084084084084081',
cvv: '408',
month: '01',
year: '20'
}
await transaction.setCard(newCard);
...
let chargeResponse = await transaction.chargeCard();
if (chargeResponse.status === 'auth') {
let authResponse;
if (chargeResponse.data.auth === 'pin') {
// request customers phone number
newCard.pin = '1234'
await transaction.setCard(newCard)
chargeResponse = await transaction.chargeChard(); // attempt to charge the card again with the pin included
}
if (chargeResponse.data.auth === 'otp') {
// request otp sent to customer
const otp = '123456';
authResponse = await transaction.authenticateCard(otp); // returns a ChargeResponse
}
}
Use this function to wait for the response from the 3DS authentication from the customer. You’d need this if the charge response returns an auth type of 3ds
:
const chargeResponse = {
status: 'auth',
message: 'url-for-3ds-authentication',
data: {
auth: '3ds',
},
}
The callback function will be called with the response and a function to unsubscribe from pusher.
Example
const chargeResponse = await transaction.chargeCard();
...
function handleResponse(response, unsubscribe) {}
if (chargeResponse.status === 'auth') {
if (chargeResponse.data.auth === '3DS') {
// redirect customer to 3ds url in `chargeResponse.message`
// listen for response from 3ds authentication
listenFor3DSCharge(handleResponse);
}
}
To charge a bank account, you need to make sure it’s from one of the banks that Paystack supports bank payments from. To get a list of supported banks, call Toolkit.fetch('banks')
.
import { Toolkit } from 'checkout-js';
const banks = await Toolkit.fetch('banks');
// This will return an array of available banks like;
[
{
"name": "Access Bank",
"slug": "access-bank",
"code": "044",
"longcode": "044150149",
"gateway": "emandate",
"pay_with_bank": true,
"active": true,
"is_deleted": null,
"country": "Nigeria",
"currency": "NGN",
"type": "nuban",
"id": 1,
"createdAt": "2016-07-14T10:04:29.000Z",
"updatedAt": "2016-07-14T10:04:29.000Z"
},
{
"name": "Guaranty Trust Bank",
"slug": "guaranty-trust-bank",
"code": "058",
"longcode": "058152036",
"gateway": "ibank",
"pay_with_bank": true,
"active": true,
"is_deleted": null,
"country": "Nigeria",
"currency": "NGN",
"type": "nuban",
"id": 9,
"createdAt": "2016-07-14T10:04:29.000Z",
"updatedAt": "2016-07-14T10:04:29.000Z"
},
...
]
checkout-js
currently handles three kinds of bank payments determined by the gateway
on the bank object. The gateways are emandate
, ibank
and digitalbankmandate
.
emandate
payments follow a “registration → verification → authentication” payment flow.ibank
payments require that your customers be redirected to their internet banking portal for authentication, after which they will be redirected back to your payment flow.digitalbankmandate
payments need to be authenticated with a phone number and a payId
Most banks use the emandate
flow while Guaranty Trust Bank and First Bank use the ibank
flow and Kuda Bank uses the digitalbankmandate
flow.
This method is used to fetch the internet banking url through which a customer can complete a transaction.
Example
...
const bank = {
...
bankId: 9,
name: 'Guaranty Trust Bank',
gateway: 'ibank',
...
};
await transaction.getIbankUrl(bank);
When the customer has been redirected to their internet banking portal to complete the transaction, you can wait for the final result using this function, passing a callback that will be called with the response and a function to unsubscribe from pusher
.
Example
...
// fetch internet banking URL
const url = await transaction.getIbankUrl(bank);
// redirect customer to internet banking portal
...
// listen for response from internet banking portal
function handleResponse(response, unsubscribe) {}
transaction.listenForIbankCharge(handleResponse);
// resolves to ChargeResponse
This function makes an attempt to charge a kuda bank account using the customer's phone number and a 6-digit payID
which the customer will generate from their kuda bank app
Example
...
const bank = {
...
id: 67,
name: 'Kuda Bank',
gateway: 'digitalbankmandate',
...
};
const phoneNumber = '07033773883'
const payId = 288299;
const params = {
phoneNumber,
bankId: bank.id,
payId
}
// resolves to ChargeResponse
await transaction.chargeDigitalBankMandate(params);
After calling the chargeDigitalBankMandate
function, you need to call the listenForDigitalBankMandateCharge
function to be notified of the status of the payment. This function accepts a callback
which will be called with the response as well as a function to unsubscribe from pusher — and a pusher_channel
, a property on data
gotten from the response to chargeDigitalBankMandate
.
const {
status,
message,
data
} = await transaction.chargeDigitalBankMandate(params);
function handleResponse(response, unsubscribe) {}
transaction.listenForDigitalBankMandateCharge(handleResponse, data.pusher_channel)
Sets the bank account the customer is going to use to complete the transaction.
Example
import { Transaction, Toolkit } from 'checkout-js';
...
const bank = {
...
id: 1,
name: 'Access Bank',
gateway: 'emandate',
type: 'nuban',
...
};
const bankAccount = {
bankId: bank.id,
accountType: bank.type,
accountNumber: '1234567890', // customer account number
}
await transaction.setBankAccount(bankAccount);
This method is responsible for charging the bank account that has been set on the transaction using transaction.setBankAccount()
.
Example
const chargeResponse = await transaction.chargeBankAccount();
// resolves to a ChargeResponse
Use this to register new customer bank accounts for e-mandate so they can be charged. You should do this if you get a response requiring birthday registration after attempting to charge a bank account with transaction.chargeBankAccount
.
{
status: 'auth',
message: 'This customer needs to register their account',
data: {
auth: 'birthday'
}
}
Example
...
await transaction.setbankAccount({...});
const chargeResponse = await transaction.chargeBankAccount();
if (chargeResponse.status === 'auth' && chargeResponse.data.auth === 'birthday') {
// pass the customer's birthday in the format 'dd-MM-yyyy'
const authResponse = await transaction.registerBankAccount('12-05-1980');
// resolves to a ChargeResponse
}
This method is used to authenticate bank transactions using an otp
.
Example
...
await transaction.setbankAccount({...});
const chargeResponse = await transaction.chargeBankAccount();
if (chargeResponse.status === 'auth' && chargeResponse.data.auth === 'otp') {
const authResponse = await transaction.authenticateBankAccount(123456);
// resolves to a ChargeResponse
}
The only available USSD channels for now are GTB’s 737, Sterling's 822, Zenith's 966 and UBA's 919
Use this to generate a USSD shortcode
Example
// initialize transaction
const response = await transaction.getUSSDShortcode({ channel: '919' });
// returns a response in the format
{
"status": true,
"message": "Offline Reference Generated Successfully",
"data": {
"reference": "887462",
"channel": "api_919_shortcode_00000",
"code": "*919*00*1*0000#"
}
}
Present the code in data.code
to your customers for payment.
Listen for when the customer completes the transaction via USSD. This function accepts a callback function to be called with the response and a function to unsubscribe from pusher.
Example
// initialize transaction
...
function handleResponse(response, unsubscribe) {}
transaction.listenForUSSDCharge({ channel: '919' }, handleResponse);
// return a ChargeResponse
The available QR channels are visa
in Nigeria and MPASS_OLTI
in South Africa .
Use this to generate a QR code
Example
// initialize transaction
const response = await transaction.getQRCode({ channel: 'visa' });
// returns a response in the format
{
"status": true,
"message": "QR successfully generated",
"data": {
"errors": false,
"url": "qr code image",
"qr_code": "qr code",
"status": "success",
"channel": "qr channel"
}
}
Display the QR code image in data.url
for your customers to scan.
Listen for when the customer has completed the transaction by scanning the QR code. This function accepts an object containing the QR channel
and a callback function to be called with the response and a function to unsubscribe from pusher.
Example
// initialize transaction
...
function handleResponse(response, unsubscribe) {}
transaction.listenForQRCharge({ channel: 'visa' }, handleResponse);
// return a ChargeResponse
This function is used to attempt a mobile money charge with a phoneNumber
, a provider
and optionally, a voucher
and a registrationToken
Available providers
[
{ name: 'MTN', value: 'MTN', prefix: ['+23354', '+23355', '+23324'] },
{ name: 'TGO',
value: 'Airtel/Tigo',
prefix: ['+23327', '+23357', '+23326', '+23356']
},
{ name: 'VOD', value: 'Vodafone', prefix: ['+23320', '+23350'] },
]
Example
// initialize transaction
...
const response = await transaction.chargeMobileMoney(
{ phoneNumber: '05012345678', provider: 'VOD' }
);
// returns a response in the format
{
"status": true,
"message": "Charge attempted",
"data": {
"transaction": 2,
"phone": "05012345678",
"device": "96640369ca64b60d3dcf",
"provider": "VOD",
"channel_name": "MOBILE_MONEY_2",
"display": {
"type": "voucher",
"message": "Please enter your voucher code to complete this payment"
}
}
}
/**
* Depending on what the `display.type` is from the previous response, you might
* need to collect additional information to complete the payment
*/
/**
* If you get a `display.type === voucher`, collect a voucher from the customer
* and call chargeMobileMoney again, this time including the voucher as one of the
* arguments
*/
const response = await transaction.chargeMobileMoney(
{
phoneNumber: '05012345678',
provider: 'VOD',
voucher: 'voucher-code'
}
);
/**
* If you get a `display.type === registration_token`, collect a registrationToken from the customer
* and call chargeMobileMoney again, this time including the registrationToken
* as one of the arguments
*/
const response = await transaction.chargeMobileMoney(
{
phoneNumber: '05012345678',
provider: 'VOD',
registrationToken: 'token'
}
);
/**
* The `registrationToken` phase should be the last stage in the process after
* which you should start listening for a response as stated in the next section
*/
Listen for when the customer has completed the payment, passing a callback function to be called with the response and a function to unsubscribe from pusher.
Example
// initialize transaction
...
function handleResponse(response, unsubscribe) {}
transaction.listenForQRCharge(handleResponse);
// return a ChargeResponse
These are a group of functions that help to populate the transaction timeline which is attached to every transaction and can be viewed on the Paystack dashboard.
Checkout JS implicitly handles some analytics like logging inputs, bank selection, errors, authentications and transaction success.
This function initializes transaction logging.
Example
// initialize transaction
...
transaction.initializeLog();
This function returns the amount of time since the transaction was requested.
Example
// initialize transaction
...
transaction.getTimeSpent();
Logs the time when the transaction page was opened
Example
// initialize transaction
...
transaction.logPageOpen();
Logs the time when the transaction authentication window was opened
Example
// initialize transaction
...
transaction.logAuthWindowOpen();
Logs the time when the transaction authentication window was closed
Example
// initialize transaction
...
transaction.logAuthWindowClose();
Log switch to a channel with name channelName
Example
// initialize transaction
...
transaction.logChannelSwitch('card');
Log validation errors on an array of inputs
Example
// initialize transaction
...
transaction.logValidationErrors(['card number']);
Log when a customer filled a certain input field
Example
// initialize transaction
...
transaction.logInput('card number');
Log when a customer makes an attempt on a channel
Example
// initialize transaction
...
transaction.logAttempt('card');
Log what bank the user selected while trying to pay with a bank account
Example
// initialize transaction
...
transaction.logBankSelect('Access Bank');
Log when a customer encounters an error while attempting to pay
Example
// initialize transaction
try {
// initialize transaction
// attempt to charge transaction
} catch(error) {
transaction.logError(error.message);
}
Log when a customer needs to be authenticated.
Example
// attempt a charge and get a response
if (response.status === 'auth') {
// log the authentication required
transaction.logAuth(response.data.auth);
}
Log when a customer has successfully completed a transaction
Example
// attempt a charge and get a response
if (response.status === 'success') {
transaction.logSuccess();
}
Log when a transaction status is pending
Example
// attempt a charge and get a response
if (response.status === 'pending') {
transaction.logPending();
}
Log when a customer closes a payment page
Example
// listen for a page close event
transaction.logPageClose();
Log the page a payment page was opened from
Example
// initialize transaction
transaction.saveReferrer(document.referrer)
Used to check the status of a transaction
Example
await transaction.requery();
// responds with a ChargeResponse
Houses various utility functions like error resolutions, supported banks, and celebrations.
Returns an array of possible parameters to be used with the Toolkit.fetch()
and their corresponding usage
Example
import { Toolkit } from 'checkout-js';
Toolkit.contents();
// output
[{
key: 'banks',
usage: 'Returns a list of banks that can be used to complete checkout'
}, {
key: 'celebrations',
usage: 'Returns a list of upcoming celebrations'
}, {
key: 'resolutions',
usage: 'Returns a list of known errors, mapped to their suggested resolutions'
}]
A static method on the Toolkit
class that operates on valid keys
from Toolkit.contents()
and returns data consistent with the usage
of each key
.
Example
import { Toolkit } from 'checkout-js'
...
const celebrations = await Toolkit.fetch('celebrations')
// celebrations.data returns a list of National celebrations and their dates:
{
"2018-06-07": "Happy Valentine's Day ❤️",
"2018-03-06": "Happy Independence Day 🇬🇭",
"2018-04-01": "Happy Easter 🙏",
"2018-05-01": "Happy Worker's Day 💪",
"2018-05-27": "Happy Children's Day 👶",
"2018-05-29": "Happy Democracy Day 🇳🇬",
"2018-06-15": "Barka da Sallah 🎉",
"2018-10-01": "Happy Independence Day 🇳🇬",
"2018-12-25": "Merry Christmas 🎉",
"2018-12-26": "Happy Boxing Day 🎁"
}
...
// params is an optional object which defaults to {pay_with_bank: '1'} if no value is passed.
const banks = await Toolkit.fetch('banks', params)
// banks.data returns;
[{
name: "Access Bank"
slug: "access-bank"
code: "044"
longcode: "044150149"
gateway: "emandate"
pay_with_bank: true
active: true
is_deleted: null
country: "Nigeria"
currency: "NGN"
type: "nuban"
id: 1
createdAt: "2016-07-14T10:04:29.000Z"
updatedAt: "2016-07-14T10:04:29.000Z"
}, { ... } ...]
A static method on the Card
class that takes an object containing cardNumber
, cvv
, month
and year
returning an object specifying if the card is valid.
Example
import { Card } from 'checkout-js';
const cardDetails = {
number: '4084084084084081',
cvv: '408',
month: '03',
year: '21',
}
Card.validate(cardDetails);
// output
// { isValid: true, errors: [] }
A static method on the Card
class that takes a card number and returns true if the card number is valid.
Example
import { Card } from 'checkout-js';
const cardNumber = '4084084084084081';
Card.isValidNumber(cardNumber);
// output
// true
A static method on the Card
class that takes a card number and returns the type of card for a valid card number
Example
import { Card } from 'checkout-js';
const cardNumber = '4084084084084081';
Card.getType(cardNumber);
// output
// {type: 'visa', maxLength: 19}
// output for invalid card number
// {type: null, maxLength: 0}
A static method on the Card
class that takes a cvv returns true if cvv supplied is valid
Example
import { Card } from 'checkout-js';
const cvv = '408';
Card.isCvvValid(cvv);
// output
// true
A static method on the Card
class that takes an expiry month and year returning true
if valid
Example
import { Card } from 'checkout-js';
const expiryMonth = '10';
const expiryYear = '2020'
Card.isExpiryValid(expiryMonth, expiryYear);
// output
// true
A static method that takes an object containing bankId
, accountType
and accountNumber
, returning an object specifying the validity of the account number supplied.
Example
import { BankAccount } from 'checkout-js';
// valid accountTypes: BankAccount.types
// valid bankIds: Toolkit.fetch('banks')
const bankDetails = {
bankId: 1,
accountType: 'nuban',
accountNumber: '0011163526'
}
BankAccount.validate(bankDetails);
// output
// {isValid: true, errors: []}
A static method on the BankAccount
class that takes a bank account number and returns true
if it is a valid ‘nuban’ account number.
Example
import { BankAccount } from 'checkout-js';
const accountNumber = '08033991672'
BankAccount.isValidNubanAccount(accountNumber);
// output
// true / false
This is the format in which all charge responses in checkout-js
are returned. A charge response will always have a status
and a message
.
This means that the charge attempt was successful
Example
{
status: 'success',
message: 'Transaction successful',
data: { ... }
}
This means that additional authentication has to be carried out to complete the transaction
Example
{
status: 'auth',
message: 'Authentication Required', // for 3DS, this would be the 3DS redirect URL
data: {
auth: 'pin' // can either be [pin, otp or 3DS]
}
}
This means that the charge attempt failed
Example
{
status: 'failed',
message: 'Transaction failed',
errors: [],
/* the 'error' object is only available if a card transaction fails. It tells us the bank the failed card was issued by. You can use this to suggest that your customer pays with their bank account, if it's one of the supported banks in Toolkit.fetch('banks') */
error: {
bank: 'Guaranty Trust Bank'
}
}
This library uses a third party service, Pusher, to listen for API responses in the following methods
Transaction.listenFor3DSCharge
Transaction.listenForIbankCharge
Transaction.listenForUSSDCharge
Transaction.listenForQRCharge
Transaction.listenForMobileMoneyCharge
Transaction.listenForDigitalBankMandateCharge
However, this does not work on non-Paystack domains so you have to use the transaction.requery()
method to check the status of the transaction when using any of those payment methods.
To ensure higher success rates, errors and failure messages should not be taken as an indication of finality, for example when a payment fails on the Paystack Checkout, the customer is prompted to retry via the same payment method or an alternative payment method.
FAQs
Client-side JS library for billing on Paystack
The npm package @paystack/checkout-js receives a total of 935 weekly downloads. As such, @paystack/checkout-js popularity was classified as not popular.
We found that @paystack/checkout-js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.
Security News
A developer is accusing Tencent of violating the GPL by modifying a Python utility and changing its license to BSD, highlighting the importance of copyleft compliance.