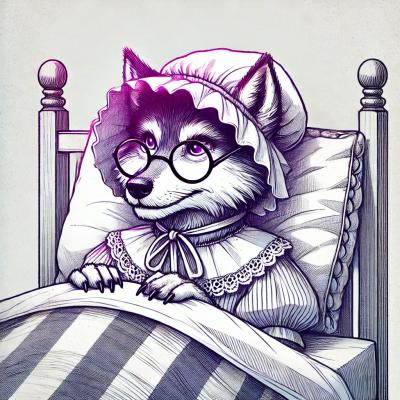
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@phenomnomnominal/tsquery
Advanced tools
The @phenomnomnominal/tsquery package is a powerful tool for querying TypeScript AST (Abstract Syntax Tree) using a CSS-like selector system. It allows developers to easily find nodes within TypeScript source code, making it useful for tasks such as code analysis, refactoring, and more.
Querying TypeScript AST
This feature allows you to query TypeScript AST nodes by using CSS-like selectors. In the provided code, `tsquery.ast` generates an AST from the source code, and `tsquery` function is used to find all identifiers named 'foo'.
import { tsquery } from '@phenomnomnominal/tsquery';
const ast = tsquery.ast(sourceCode);
const nodes = tsquery(ast, 'Identifier[name="foo"]');
Counting specific nodes
This feature is useful for analyzing the code to count specific types of statements or nodes. Here, the code counts how many 'IfStatement' nodes are in the provided source code.
import { tsquery } from '@phenomnomnominal/tsquery';
const ast = tsquery.ast(sourceCode);
const count = tsquery(ast, 'IfStatement').length;
typescript-eslint is a plugin that allows for linting TypeScript code with ESLint. It uses an AST to analyze the code, similar to tsquery, but is more focused on linting and enforcing coding standards rather than general querying.
ts-morph provides an easier API to work with the TypeScript compiler's AST. It offers functionalities similar to tsquery but with additional features like code manipulation and emitting, making it more versatile for tasks that involve modifying the codebase.
TSQuery is a port of the ESQuery API for TypeScript! TSQuery allows you to query a TypeScript AST for patterns of syntax using a CSS style selector system.
Check out the ESQuery demo - note that the demo requires JavaScript code, not TypeScript
You can also check out the TSQuery Playground - Lovingly crafted by Uri Shaked
npm install @phenomnomnominal/tsquery --save-dev
Say we want to select all instances of an identifier with name "Animal", e.g. the identifier in the class
declaration, and the identifier in the extends
declaration.
We would do something like the following:
import { tsquery } from '@phenomnomnominal/tsquery';
const typescript = `
class Animal {
constructor(public name: string) { }
move(distanceInMeters: number = 0) {
console.log(\`\${this.name} moved \${distanceInMeters}m.\`);
}
}
class Snake extends Animal {
constructor(name: string) { super(name); }
move(distanceInMeters = 5) {
console.log("Slithering...");
super.move(distanceInMeters);
}
}
`;
const ast = tsquery.ast(typescript);
const nodes = tsquery(ast, 'Identifier[name="Animal"]');
console.log(nodes.length); // 2
Try running this code in StackBlitz!
The following selectors are supported:
ForStatement
(see common node types)*
[attr]
[attr="foo"]
or [attr=123]
[attr=/foo.*/]
[attr!="foo"]
, [attr>2]
, [attr<3]
, [attr>=2]
, or [attr<=3]
[attr.level2="foo"]
FunctionDeclaration > Identifier.id
:first-child
or :last-child
:nth-child(2)
:nth-last-child(1)
ancestor descendant
parent > child
node ~ sibling
node + adjacent
:not(ForStatement)
:matches([attr] > :first-child, :last-child)
IfStatement:has([name="foo"])
:statement
, :expression
, :declaration
, :function
, or :pattern
Identifier
- any identifier (name of a function, class, variable, etc)IfStatement
, ForStatement
, WhileStatement
, DoStatement
- control flowFunctionDeclaration
, ClassDeclaration
, ArrowFunction
- declarationsVariableStatement
- var, const, let.ImportDeclaration
- any import
statementStringLiteral
- any stringTrueKeyword
, FalseKeyword
, NullKeyword
, AnyKeyword
- various keywordsCallExpression
- function callNumericLiteral
- any numeric constantNoSubstitutionTemplateLiteral
, TemplateExpression
- template strings and expressionsFAQs
Query TypeScript ASTs with the esquery API!
We found that @phenomnomnominal/tsquery demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.