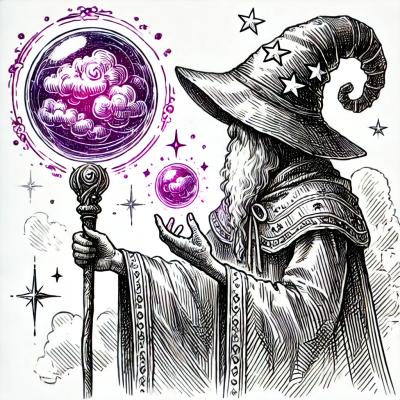
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
@pixi/ticker
Advanced tools
@pixi/ticker is a module from the PixiJS library that provides a highly efficient and flexible ticker for managing animation frames and timed updates. It is particularly useful for game development and other real-time applications where precise control over the rendering loop is required.
Basic Ticker Usage
This code demonstrates how to create a basic ticker, add a callback function to it, and start the ticker. The callback function logs the delta time (time elapsed since the last frame) to the console.
const { Ticker } = require('@pixi/ticker');
const ticker = new Ticker();
ticker.add((deltaTime) => {
console.log(`Delta time: ${deltaTime}`);
});
ticker.start();
Adding Multiple Callbacks
This code shows how to add multiple callback functions to a single ticker. Both callbacks will be executed on each tick, logging their respective messages to the console.
const { Ticker } = require('@pixi/ticker');
const ticker = new Ticker();
const callback1 = (deltaTime) => {
console.log(`Callback 1 - Delta time: ${deltaTime}`);
};
const callback2 = (deltaTime) => {
console.log(`Callback 2 - Delta time: ${deltaTime}`);
};
ticker.add(callback1);
ticker.add(callback2);
ticker.start();
Removing Callbacks
This code demonstrates how to remove a callback from the ticker after a certain period of time (5 seconds in this case). The callback will no longer be executed after it is removed.
const { Ticker } = require('@pixi/ticker');
const ticker = new Ticker();
const callback = (deltaTime) => {
console.log(`Delta time: ${deltaTime}`);
};
ticker.add(callback);
ticker.start();
setTimeout(() => {
ticker.remove(callback);
console.log('Callback removed');
}, 5000);
Pausing and Resuming the Ticker
This code shows how to pause and resume the ticker. The ticker is paused after 3 seconds and resumed after 6 seconds, with appropriate messages logged to the console.
const { Ticker } = require('@pixi/ticker');
const ticker = new Ticker();
ticker.add((deltaTime) => {
console.log(`Delta time: ${deltaTime}`);
});
ticker.start();
setTimeout(() => {
ticker.stop();
console.log('Ticker paused');
}, 3000);
setTimeout(() => {
ticker.start();
console.log('Ticker resumed');
}, 6000);
The 'raf' package provides a simple polyfill for requestAnimationFrame, which is used to create smooth animations in the browser. Unlike @pixi/ticker, 'raf' is more lightweight and does not offer built-in support for managing multiple callbacks or delta time calculations.
Anime.js is a lightweight JavaScript animation library with a simple, yet powerful API. It provides more advanced animation capabilities compared to @pixi/ticker, including keyframes, timelines, and easing functions. However, it is more focused on creating complex animations rather than managing a rendering loop.
GSAP (GreenSock Animation Platform) is a robust JavaScript library for creating high-performance animations. It offers a wide range of features, including timelines, tweens, and various easing options. While GSAP is more feature-rich for animation purposes, @pixi/ticker is more specialized for managing the rendering loop in real-time applications.
npm install @pixi/ticker
Create a Ticker object directly:
import { Ticker } from '@pixi/ticker';
const ticker = new Ticker();
ticker.start();
Use as an Application plugin:
import '@pixi/ticker';
import { Application } from '@pixi/app';
const app = new Application();
app.ticker.start();
FAQs
Tickers are control the timings within PixiJS
The npm package @pixi/ticker receives a total of 97,963 weekly downloads. As such, @pixi/ticker popularity was classified as popular.
We found that @pixi/ticker demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.