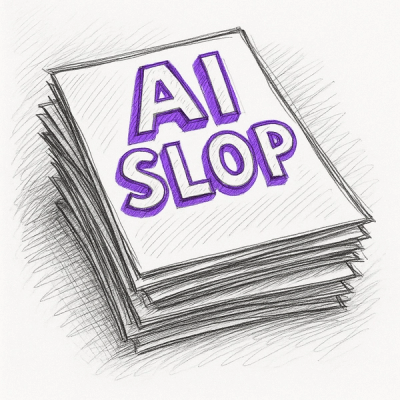
Security News
Django Joins curl in Pushing Back on AI Slop Security Reports
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
@pluginjs/dom
Advanced tools
dom
is a utility JavaScript library for control dom interfaces.
yarn add @pluginjs/dom
npm i @pluginjs/dom
CDN:
Development:
<script src="https://unpkg.com/@pluginjs/dom/dist/dom.js"></script>
Production:
<script src="https://unpkg.com/@pluginjs/dom/dist/dom.min.js"></script>
Alias: parentNode.querySelector
Parameters
| Name | Type | Description |
|||-|
| selector | String
| CSS selectors |
| parent | HTMLElement
| Default: document
|
Returns
| Name | Type | Description |
|||-|
| element | HTMLElement
| An Element object representing the first element in the document that matches the specified set of CSS selectors, or null is returned if there are no matches. |
Alias: parentNode.querySelectorAll
Parameters
| Name | Type | Description |
|||-|
| selector | String
| CSS selectors |
| parent | HTMLElement
| Default: document
|
Returns
| Name | Type | Description |
|||-|
| elements | Array<HTMLElement>
| A non-live Array containing one Element object for each element that matches at least one of the specified selectors or an empty Array in case of no matches. |
Just like query, but it is Curried.
Just like queryAll, but it is Curried.
Parameters
| Name | Type | Description |
|||-|
| element | HTMLElement
| |
Parameters
| Name | Type | Description |
|||-|
| content | String
| html string |
| element | HTMLElement
| container |
Returns
| Name | Type | Description |
|||-|
| element | HTMLElement
| container |
Parameters
| Name | Type | Description |
|||-|
| selector | String
| CSS selectors |
| parent | HTMLElement
| |
Returns
| Name | Type | Description |
|||-|
| elements | Array<HTMLElement>
| a Array which contains all of the child elements that matches the specified set of CSS selectors . |
Parameters
| Name | Type | Description |
|||-|
| element | HTMLElement
| |
Returns
| Name | Type | Description |
|||-|
| element | HTMLElement
| |
Parameters
| Name | Type | Description |
|||-|
| element | HTMLElement
| |
Returns
| Name | Type | Description |
|||-|
| element | HTMLElement
| parentNode |
Support tagged template.
Parameters
| Name | Type | Description |
|||-|
| htmlString | String
| |
Returns
| Name | Type | Description |
|||-|
| element | HTMLElement
| |
Parameters
| Name | Type | Description |
|||-|
| key | String
| |
| value | Any
| |
| el | HTMLElement
| |
Returns
| Name | Type | Description |
|||-|
| element | HTMLElement
| |
Parameters
| Name | Type | Description |
|||-|
| key | String
| |
| el | HTMLElement
| |
Returns
| Name | Type | Description |
|||-|
| element | HTMLElement
| |
Parameters
| Name | Type | Description |
|||-|
| element | HTMLElement
| |
Returns
| Name | Type | Description |
|||-|
| element | HTMLElement
| |
Parameters
| Name | Type | Description |
|||-|
| element | HTMLElement
| |
Returns
| Name | Type | Description |
|||-|
| element | HTMLElement
| |
Alias: node.previousElementSibling
Parameters
| Name | Type | Description |
|||-|
| element | HTMLElement
| |
Returns
| Name | Type | Description |
|||-|
| element | HTMLElement
| |
Alias: node.nextElementSibling
Parameters
| Name | Type | Description |
|||-|
| element | HTMLElement
| |
Returns
| Name | Type | Description |
|||-|
| element | HTMLElement
| |
Parameters
| Name | Type | Description |
|||-|
| args | String | Object
| |
Returns
| Name | Type | Description |
|||-|
| element | HTMLElement
| |
Parameters
| Name | Type | Description |
|||-|
| key | String
| |
Returns
| Name | Type | Description |
|||-|
| element | HTMLElement
| |
Parameters
| Name | Type | Description |
|||-|
| args | String | Object
| |
Returns
| Name | Type | Description |
|||-|
| element | HTMLElement
| |
Parameters
| Name | Type | Description |
|||-|
| content | String
| textContent |
| element | HTMLElement
| |
Returns
| Name | Type | Description |
|||-|
| element | HTMLElement
| |
Parameters
| Name | Type | Description |
|||-|
| child | HTMLElement
| childNode |
| el | HTMLElement
| parentNode |
Returns
| Name | Type | Description |
|||-|
| el | HTMLElement
| parentNode |
Parameters
| Name | Type | Description |
|||-|
| child | HTMLElement
| childNode |
| el | HTMLElement
| parentNode |
Returns
| Name | Type | Description |
|||-|
| el | HTMLElement
| parentNode |
Parameters
| Name | Type | Description |
|||-|
| newElement | HTMLElement
| new element |
| el | HTMLElement
| parentNode |
Returns
| Name | Type | Description |
|||-|
| el | HTMLElement
| parentNode |
Parameters
| Name | Type | Description |
|||-|
| newElement | HTMLElement
| new element |
| el | HTMLElement
| parentNode |
Returns
| Name | Type | Description |
|||-|
| el | HTMLElement
| parentNode |
Parameters
| Name | Type | Description |
|||-|
| wrapElement | HTMLElement
| wrapper |
| el | HTMLElement
| |
Returns
| Name | Type | Description |
|||-|
| wrapElement | HTMLElement
| wrapper |
Parameters
| Name | Type | Description |
|||-|
| newElement | HTMLElement
| new element |
| wrap | HTMLElement
| wrapper |
Returns
| Name | Type | Description |
|||-|
| wrap | HTMLElement
| wrapper |
Parameters
| Name | Type | Description |
|||-|
| wrapElement | HTMLElement
| wrapper |
| elementList | Array<HTMLElement>
| |
Returns
| Name | Type | Description |
|||-|
| wrapElement | HTMLElement
| wrapper |
Parameters
| Name | Type | Description |
|||-|
| el | HTMLElement
| |
Returns
| Name | Type | Description |
|||-|
| el | HTMLElement
| |
Parameters
| Name | Type | Description |
|||-|
| fn | HTMLElement => Boolean
| |
| el | HTMLElement
| |
Returns
| Name | Type | Description |
|||-|
| el | HTMLElement
| parentNode |
Parameters
| Name | Type | Description |
|||-|
| el | HTMLElement
| |
Returns
| Name | Type | Description |
|||-|
| el | HTMLElement
| |
Parameters
| Name | Type | Description |
|||-|
| el | HTMLElement
| |
| parent | HTMLElement
| |
Returns
| Name | Type | Description |
|||-|
| bool | Boolean
| |
Parameters
| Name | Type | Description |
|||-|
| selector | String
| |
| el | HTMLElement
| |
Returns
| Name | Type | Description |
|||-|
| parentElement | HTMLElement
| |
Parameters
| Name | Type | Description |
|||-|
| fn | HTMLElement => Boolean
| |
| el | HTMLElement
| |
Returns
| Name | Type | Description |
|||-|
| nextElement | HTMLElement
| |
Parameters
| Name | Type | Description |
|||-|
| type | String
| |
| Options | { duration: Number, callback: Function }
| |
| element | HTMLElement
|
Returns
| Name | Type | Description |
|||-|
| nextElement | HTMLElement
| |
Parameters
| Name | Type | Description |
|||-|
| Options | { duration: Number, callback: Function }
| |
| element | HTMLElement
|
Returns
| Name | Type | Description |
|||-|
| nextElement | HTMLElement
| |
Parameters
| Name | Type | Description |
|||-|
| Options | { duration: Number, callback: Function }
| |
| element | HTMLElement
|
Returns
| Name | Type | Description |
|||-|
| nextElement | HTMLElement
| |
Tested on all major browsers.
![]() IE / Edge | ![]() Firefox | ![]() Chrome | ![]() Safari | ![]() Opera |
---|---|---|---|---|
IE11, Edge | last 2 versions | last 2 versions | last 2 versions | last 2 versions |
@pluginjs/dom is Licensed under the GPL-v3 license.
If you want to use @pluginjs/dom project to develop commercial sites, themes, projects, and applications, the Commercial license is the appropriate license. With this option, your source code is kept proprietary.
For purchase an Commercial License, contact us purchase@thecreation.co.
Copyright (C) 2022 Creation Studio Limited.
FAQs
A flexible modern dom js plugin.
The npm package @pluginjs/dom receives a total of 25 weekly downloads. As such, @pluginjs/dom popularity was classified as not popular.
We found that @pluginjs/dom demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.
Security News
A new Node.js homepage button linking to paid support for EOL versions has sparked a heated discussion among contributors and the wider community.