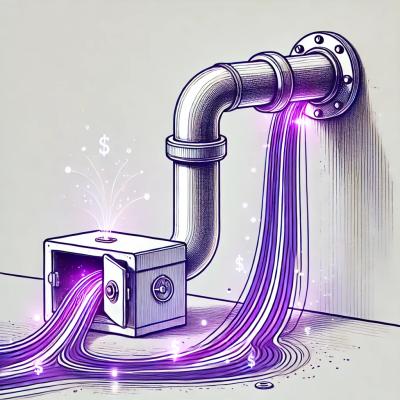
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
@premieroctet/react-native-wallet
Advanced tools
A React-Native wrapper for Apple PassKit and Google Wallet API
A module built with Expo Modules that provides wallet features for iOS and Android.
Uses PassKit on iOS, Google Wallet API on Android.
yarn add @premieroctet/react-native-wallet
This module is not compatible with Expo Go. You will need to build a custom dev client.
You will need to install Expo Modules on your project.
import { Alert, Button, StyleSheet, View } from 'react-native';
import * as RNWallet from 'react-native-wallet';
export default function App() {
const onAdd = async () => {
try {
const isAdded = await RNWallet.addPass('<PassUrlOrToken>');
Alert.alert('Pass added', isAdded ? 'Yes' : 'No');
} catch (error) {
Alert.alert('Error', (error as Error).message);
}
};
const onCheckPassExists = async () => {
try {
const passExists = await RNWallet.hasPass('<PassUrlOrToken>');
Alert.alert('Pass exists', passExists ? 'Yes' : 'No');
} catch (error) {
Alert.alert('Error', (error as Error).message);
}
};
const onRemovePass = async () => {
try {
await RNWallet.removePass('<PassUrlOrToken>');
Alert.alert('Pass removed');
} catch (error) {
Alert.alert('Error', (error as Error).message);
}
};
const onCanAddPasses = async () => {
try {
const canAddPasses = await RNWallet.canAddPasses();
Alert.alert('Can add passes', canAddPasses ? 'Yes' : 'No');
} catch (error) {
Alert.alert('Error', (error as Error).message);
}
};
return (
<View style={styles.container}>
<Button title="Check if can add passes" onPress={onCanAddPasses} />
<Button title="Check if pass exists" onPress={onCheckPassExists} />
<Button title="Remove pass" onPress={onRemovePass} />
<RNWallet.RNWalletView
buttonStyle={RNWallet.ButtonStyle.BLACK}
buttonType={RNWallet.ButtonType.PRIMARY}
onPress={onAdd}
/>
</View>
);
}
canAddPasses(): boolean
: Check if the device can add passes.addPass(urlOrToken: string): Promise<boolean>
: Add a pass to the wallet. Returns true
if the pass was added or if its already added. Returns false
if the user cancelled the operation. urlOrToken
should be the pkpass URL for iOS, and the pass JWT for Android.hasPass(urlOrToken: string): Promise<boolean>
: Check if a pass exists in the wallet. Returns true
if the pass exists, false
otherwise. On Android, this always returns false
.removePass(urlOrToken: string): Promise<void>
: Remove a pass from the wallet. On Android, this is no-op. On iOS, make sure you have the correct entitlements. See documentation.buttonStyle: ButtonStyle
: The button style to use for the iOS button. Can be either BLACK
or BLACK_OUTLINE
. Defaults to BLACK
. Typescript users: use the ButtonStyle enumbuttonType: ButtonType
: The button type to use for the Android button. Can be either PRIMARY
or CONDENSED
. Defaults to PRIMARY
. Typescript users: use the ButtonType enumonPress: () => void
: The callback executed when the button is pressed.style: ViewStyle
: The style to apply to the button. By default, width and height are already set. On Android, the width is set depending on the button type.The library exports a Constants
object with the following properties:
buttonLayout
:
baseWidth
: The base width of the button. It is undefined on Android.baseHeight
: The base height of the button.baseWidthFromType
: Function that takes the button type as parameter and returns the base width of the button depending on it. On iOS, it returns undefined.Contributions are very welcome!
To get started, fork and clone the repo, and install the dependencies in both root and example folders. Don't forget to install the pods in the example's ios folder.
To run the example app, run yarn start
in the root folder, and yarn ios
or yarn android
in the example folder.
To updathe iOS code, run yarn open:ios
, and edit the RNWallet
pod files.
To update the Android code, run yarn open:android
and edit the react-native-wallet
module.
FAQs
A React-Native wrapper for Apple PassKit and Google Wallet API
We found that @premieroctet/react-native-wallet demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.