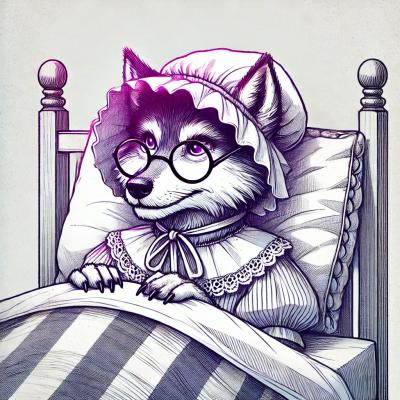
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@protobufjs/codegen
Advanced tools
@protobufjs/codegen is a utility for generating JavaScript code dynamically. It is particularly useful in the context of Protocol Buffers, where it can be used to generate efficient serialization and deserialization code.
Dynamic Code Generation
This feature allows you to dynamically generate JavaScript functions. In this example, a function `encode` is generated which calls a method `m.encode` on a message and returns the result.
const codegen = require('@protobufjs/codegen');
const gen = codegen('m', 'exports')
('function encode(message) {')
(' return m.encode(message).finish();')
('}');
console.log(gen.toString());
Function Compilation
This feature allows you to compile the generated code into a real JavaScript function. In this example, the `encode` function is compiled and can be used directly.
const codegen = require('@protobufjs/codegen');
const gen = codegen('m', 'exports')
('function encode(message) {')
(' return m.encode(message).finish();')
('}');
const encode = gen(m, {});
console.log(encode.toString());
protobufjs is a comprehensive library for working with Protocol Buffers in JavaScript. It includes functionality for parsing .proto files, encoding and decoding messages, and more. While @protobufjs/codegen focuses on dynamic code generation, protobufjs provides a full suite of tools for working with Protocol Buffers.
google-protobuf is the official Protocol Buffers library for JavaScript provided by Google. It offers similar functionalities for encoding and decoding Protocol Buffers messages but does not focus on dynamic code generation like @protobufjs/codegen.
typescript-json-schema is a tool for generating JSON schemas from TypeScript types. While it is not directly related to Protocol Buffers, it shares the concept of generating code (in this case, schemas) from a defined structure. It does not offer dynamic code generation like @protobufjs/codegen.
A minimalistic code generation utility.
codegen([functionParams: string[]
], [functionName: string]): Codegen
Begins generating a function.
codegen.verbose = false
When set to true, codegen will log generated code to console. Useful for debugging.
Invoking codegen returns an appender function that appends code to the function's body and returns itself:
Codegen(formatString: string
, [...formatParams: any
]): Codegen
Appends code to the function's body. The format string can contain placeholders specifying the types of inserted format parameters:
%d
: Number (integer or floating point value)%f
: Floating point value%i
: Integer value%j
: JSON.stringify'ed value%s
: String value%%
: Percent signCodegen([scope: Object.<string,*>
]): Function
Finishes the function and returns it.
Codegen.toString([functionNameOverride: string
]): string
Returns the function as a string.
var codegen = require("@protobufjs/codegen");
var add = codegen(["a", "b"], "add") // A function with parameters "a" and "b" named "add"
("// awesome comment") // adds the line to the function's body
("return a + b - c + %d", 1) // replaces %d with 1 and adds the line to the body
({ c: 1 }); // adds "c" with a value of 1 to the function's scope
console.log(add.toString()); // function add(a, b) { return a + b - c + 1 }
console.log(add(1, 2)); // calculates 1 + 2 - 1 + 1 = 3
License: BSD 3-Clause License
FAQs
A minimalistic code generation utility.
The npm package @protobufjs/codegen receives a total of 14,929,687 weekly downloads. As such, @protobufjs/codegen popularity was classified as popular.
We found that @protobufjs/codegen demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.