quill-magic-url
Checks for URLs during typing and pasting and automatically converts them to links and normalizes the links URL.
Thanks to @LFDM for the groundwork with quill-auto-links.
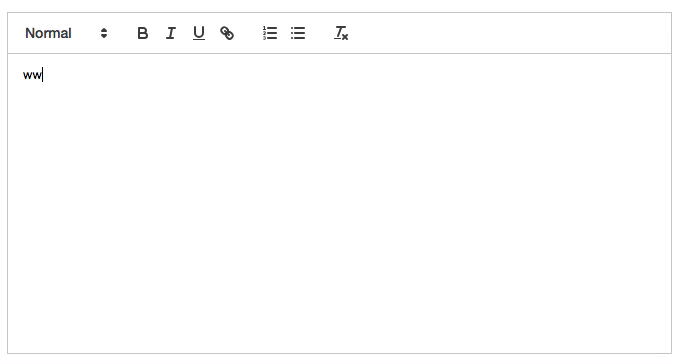
Install
npm install quill-magic-url --save
Basic Usage
import Quill from 'quill';
import MagicUrl from 'quill-magic-url';
Quill.register('modules/magicUrl', MagicUrl);
Basic usage with default options:
const quill = new Quill(editor, {
modules: {
magicUrl: true
}
});
Usage with custom options:
const quill = new Quill(editor, {
modules: {
magicUrl: {
urlRegularExpression: /(https?:\/\/[\S]+)|(www.[\S]+)|(mailto:[\S]+)|(tel:[\S]+)/,
globalRegularExpression: /(https?:\/\/|www\.|mailto:|tel:)[\S]+/g
}
}
});
Options
urlRegularExpression
Regex used to check for URLs during typing.
Default: /(https?:\/\/[\S]+)|(www.[\S]+)/
Example with custom Regex
magicUrl: {
urlRegularExpression: /(https?:\/\/[\S]+)|(www.[\S]+)|(mailto:[\S]+)|(tel:[\S]+)/
}
globalRegularExpression
Regex used to check for URLs on paste.
Default: /(https?:\/\/|www\.)[\S]+/g
Example with custom Regex
magicUrl: {
globalRegularExpression: /(https?:\/\/|www\.|mailto:|tel:)[\S]+/g
}
normalizeRegularExpression
Regex used to check for URLs to be normalized.
Default: /(https?:\/\/[\S]+)|(www.[\S]+)/
You will most likely want to keep this options default value.
normalizeUrlOptions
Options for normalizing the URL
Default:
{
stripWWW: false
}
Example with custom options
magicUrl: {
normalizeUrlOptions: {
stripHash: true,
stripWWW: false,
normalizeProtocol: false
}
}
Available options
We use normalize-url for normalizing URLs. You can find a detailed description of the possible options here.
More infos on URL Regex
For some advanced URL Regex check this out.