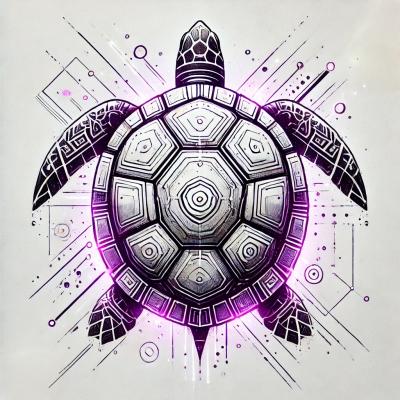
Security Fundamentals
Turtles, Clams, and Cyber Threat Actors: Shell Usage
The Socket Threat Research Team uncovers how threat actors weaponize shell techniques across npm, PyPI, and Go ecosystems to maintain persistence and exfiltrate data.
@pulumi/github
Advanced tools
A Pulumi package for creating and managing github cloud resources.
@pulumi/github is an npm package that allows you to manage GitHub resources using Pulumi, a modern infrastructure as code platform. With this package, you can automate the creation, configuration, and management of GitHub repositories, teams, issues, and more using Pulumi's programming model.
Manage Repositories
This feature allows you to create and manage GitHub repositories. The code sample demonstrates how to create a new public repository named 'my-repo' with a description using Pulumi.
const pulumi = require('@pulumi/pulumi');
const github = require('@pulumi/github');
const repo = new github.Repository('my-repo', {
name: 'my-repo',
description: 'My awesome repository',
private: false
});
exports.repoName = repo.name;
Manage Teams
This feature allows you to create and manage GitHub teams. The code sample demonstrates how to create a new team named 'my-team' with a description and closed privacy setting using Pulumi.
const pulumi = require('@pulumi/pulumi');
const github = require('@pulumi/github');
const team = new github.Team('my-team', {
name: 'my-team',
description: 'My awesome team',
privacy: 'closed'
});
exports.teamName = team.name;
Manage Issues
This feature allows you to create and manage GitHub issues. The code sample demonstrates how to create a new issue in the 'my-repo' repository with a title, body, and assignee using Pulumi.
const pulumi = require('@pulumi/pulumi');
const github = require('@pulumi/github');
const issue = new github.Issue('my-issue', {
repository: 'my-repo',
title: 'Bug: Something is broken',
body: 'Detailed description of the issue',
assignees: ['username']
});
exports.issueTitle = issue.title;
Octokit is the official GitHub SDK for JavaScript. It provides a comprehensive set of tools to interact with the GitHub API, allowing you to manage repositories, issues, pull requests, and more. Unlike @pulumi/github, which integrates with Pulumi for infrastructure as code, Octokit is a standalone library focused solely on GitHub API interactions.
Node-GitHub is a client library for the GitHub API written in JavaScript. It allows you to interact with various GitHub resources such as repositories, issues, and users. While it offers similar functionalities to @pulumi/github, it does not integrate with Pulumi and is used directly for API interactions.
The 'gh' package is a command-line tool for GitHub. It allows you to perform various GitHub operations such as creating issues, managing pull requests, and more from the command line. Unlike @pulumi/github, which is used within a Pulumi program, 'gh' is used directly from the terminal.
The GitHub resource provider for Pulumi lets you use GitHub resources in your infrastructure programs. To use this package, please install the Pulumi CLI first.
This package is available in many languages in the standard packaging formats.
To use from JavaScript or TypeScript in Node.js, install using either npm
:
$ npm install @pulumi/github
or yarn
:
$ yarn add @pulumi/github
To use from Python, install using pip
:
$ pip install pulumi-github
To use from Go, use go get
to grab the latest version of the library
$ go get github.com/pulumi/pulumi-github/sdk/v6
To use from .NET, install using dotnet add package
:
$ dotnet add package Pulumi.Github
The following configuration points are available:
github:token
- (Optional) This is the GitHub personal access token. It can also be sourced from the GITHUB_TOKEN
environment variable. If anonymous is false, token is required.github:baseUrl
- (Optional) This is the target GitHub base API endpoint. Providing a value is a requirement when
working with GitHub Enterprise. It is optional to provide this value and it can also be sourced from the GITHUB_BASE_URL
environment variable. The value must end with a slash, and generally includes the API version, for instance
https://github.someorg.example/api/v3/
.github:owner
- (Optional) This is the target GitHub organization or individual user account to manage. For example,
torvalds
and github
are valid owners. It is optional to provide this value and it can also be sourced from the
GITHUB_OWNER
environment variable. When not provided and a token is available, the individual user account owning
the token will be used. When not provided and no token is available, the provider may not function correctly.github:organization
- (Deprecated) This behaves the same as owner, which should be used instead. This value can also
be sourced from the GITHUB_ORGANIZATION
environment variable.For further information, please visit the GitHub provider docs or for detailed reference documentation, please visit the API docs.
FAQs
A Pulumi package for creating and managing github cloud resources.
The npm package @pulumi/github receives a total of 310,509 weekly downloads. As such, @pulumi/github popularity was classified as popular.
We found that @pulumi/github demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security Fundamentals
The Socket Threat Research Team uncovers how threat actors weaponize shell techniques across npm, PyPI, and Go ecosystems to maintain persistence and exfiltrate data.
Security News
At VulnCon 2025, NIST scrapped its NVD consortium plans, admitted it can't keep up with CVEs, and outlined automation efforts amid a mounting backlog.
Product
We redesigned our GitHub PR comments to deliver clear, actionable security insights without adding noise to your workflow.