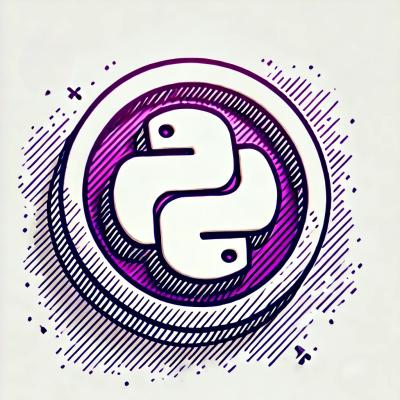
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
@qwick/apollo
Advanced tools
React-apollo with Reason
yarn add reason-apollo
# Add graphql_ppx
yarn add --dev graphql_ppx
# Add JS dependencies
yarn add react-apollo apollo-client apollo-cache-inmemory apollo-link apollo-link-context apollo-link-error apollo-link-http graphql graphql-tag apollo-link-ws apollo-upload-client subscriptions-transport-ws
Add reason-apollo
to your bs-dependencies
and
graphql_ppx/ppx
to your ppx_flags
bsconfig.json
"bs-dependencies": [
"reason-react",
"reason-apollo"
],
"ppx-flags": [
"graphql_ppx/ppx"
]
This will generate a graphql_schema.json
which will be used to safely type your GraphQL queries/mutations.
yarn send-introspection-query http://my-api.example.com/api
Watch its usage in this video:
Client.re
/* Create an InMemoryCache */
let inMemoryCache = ApolloInMemoryCache.createInMemoryCache();
/* Create an HTTP Link */
let httpLink =
ApolloLinks.createHttpLink(~uri="http://localhost:3010/graphql", ());
let instance =
ReasonApollo.createApolloClient(~link=httpLink, ~cache=inMemoryCache, ());
Index.re
/*
Enhance your application with the `ReasonApollo.Provider`
passing it your client instance
*/
ReactDOMRe.renderToElementWithId(
<ReasonApollo.Provider client=Client.instance>
<App />
</ReasonApollo.Provider>,
"index",
);
MyComponent.re
/* Create a GraphQL Query by using the graphql_ppx */
module GetPokemon = [%graphql
{|
query getPokemon($name: String!){
pokemon(name: $name) {
name
}
}
|}
];
module GetPokemonQuery = ReasonApollo.CreateQuery(GetPokemon);
let make = _children => {
/* ... */,
render: _ => {
let pokemonQuery = GetPokemon.make(~name="Pikachu", ());
<GetPokemonQuery variables=pokemonQuery##variables>
...{
({result}) =>
switch (result) {
| Loading => <div> {ReasonReact.string("Loading")} </div>
| Error(error) =>
<div> {ReasonReact.string(error##message)} </div>
| Data(response) =>
<div> {ReasonReact.string(response##pokemon##name)} </div>
}
}
</GetPokemonQuery>;
},
};
MyMutation.re
module AddPokemon = [%graphql
{|
mutation addPokemon($name: String!) {
addPokemon(name: $name) {
name
}
}
|}
];
module AddPokemonMutation = ReasonApollo.CreateMutation(AddPokemon);
let make = _children => {
/* ... */,
render: _ =>
<AddPokemonMutation>
...{
(mutation /* Mutation to call */, _) => {
/* Result of your mutation */
let newPokemon = AddPokemon.make(~name="Bob", ());
<div>
<button
onClick={
_mouseEvent =>
mutation(
~variables=newPokemon##variables,
~refetchQueries=[|"getAllPokemons"|],
(),
)
|> ignore
}>
{ReasonReact.string("Add Pokemon")}
</button>
</div>;
}
}
</AddPokemonMutation>,
};
If you simply want to have access to the ApolloClient, you can use the ApolloConsumer
<ApolloConsumer>
...{apolloClient => {/* We have access to the client! */}}
</ApolloConsumer>;
@bsRecord
on response objectThe @bsRecord
modifier is an extension of the graphql syntax for BuckleScipt/ReasonML. It allows you to convert a reason object to a reason record and reap the benefits of pattern matching. For example, let's say I have a nested object of options. I would have to do something like this:
switch response##object {
| Some(object) => {
switch object##nestedValue {
| Some(nestedValue) => nestedValue
| None => ""
}
}
| None => ""
}
Kind of funky, huh? Let's modify the response and convert it to a reason record.
type object = {
nestedValue: option(string)
}
module GetObject = [%graphql {|
object @bsRecord {
nestedValue
}
|}
];
This time we can pattern match more precisely.
switch response##object {
| Some({ nestedValue: Some(value) }) => value
| Some({ nestedValue: None }) => ""
| None => ""
}
You might find yourself consuming an API with field names like Field
. Currently, reason object field names are required to be camel case. Therefore if you have a request like this:
{
Object {
id
title
}
}
You will attempt to access the response object but it will throw an error:
response##Object; /* Does not work :( */
Instead, use an alias
to modify the response:
{
object: Object {
id
title
}
}
Then you can access the object like this:
response##object
You can create a generic error and Loading component and compose them like this example:
module QueryView = {
let component = ReasonReact.statelessComponent(__MODULE__);
let make =
(
~result: ReasonApolloTypes.queryResponse('a),
~accessData: 'a => option('b),
~render: ('b, 'c) => ReasonReact.reactElement,
~onLoadMore: ('b, 'unit) => unit=(_, ()) => (),
_children,
) => {
...component,
render: _self =>
switch (result) {
| Error(error) => <Error />
| Loading => ReasonReact.null
| Data(response) =>
switch (accessData(response)) {
| Some(data) => render(data, onLoadMore(data))
| _ => <Error error="" />
}
},
};
};
In some cases, it seems like there are some differences between the provided send-introspection-query
and output from tools you might be using to download the schema (such as apollo-codegen
or graphql-cli
).
If your build is failing, please make sure to try with the provided script. In your project root, run:
$ yarn send-introspection-query <url>
FAQs
BuckleScript bindings for Apollo Client and React Apollo
The npm package @qwick/apollo receives a total of 0 weekly downloads. As such, @qwick/apollo popularity was classified as not popular.
We found that @qwick/apollo demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.